Top Strategies for Effective React Native State Management
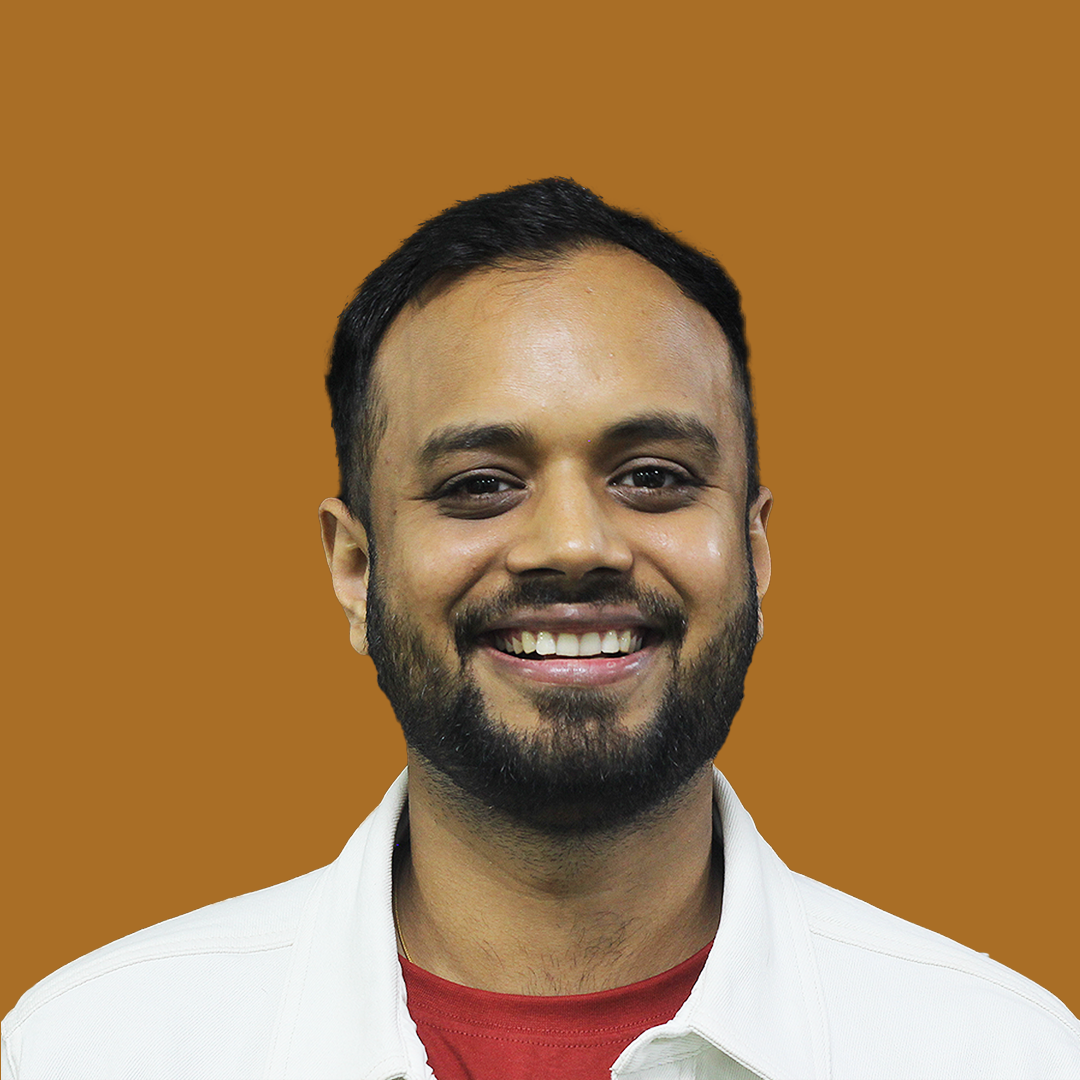
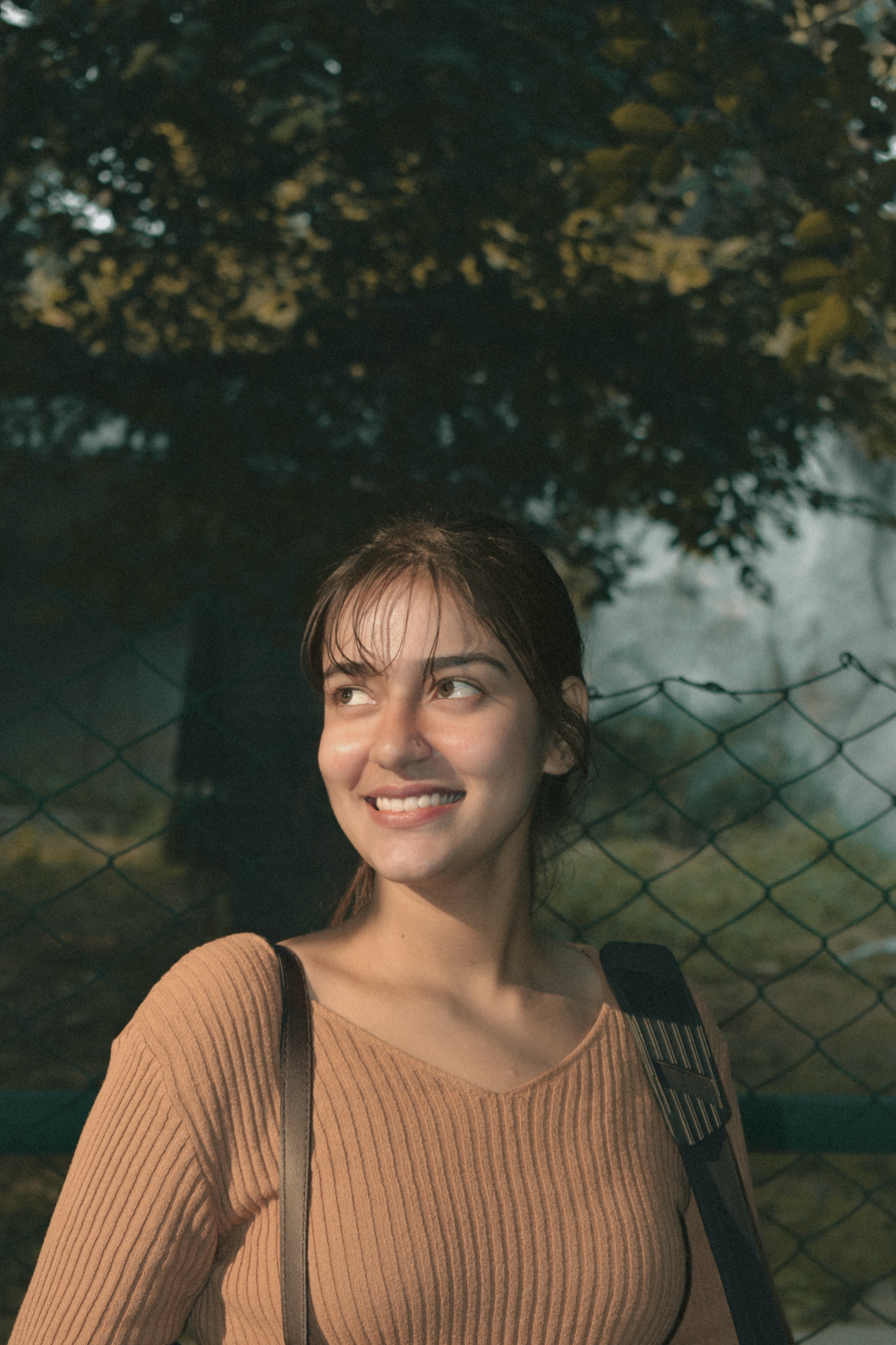
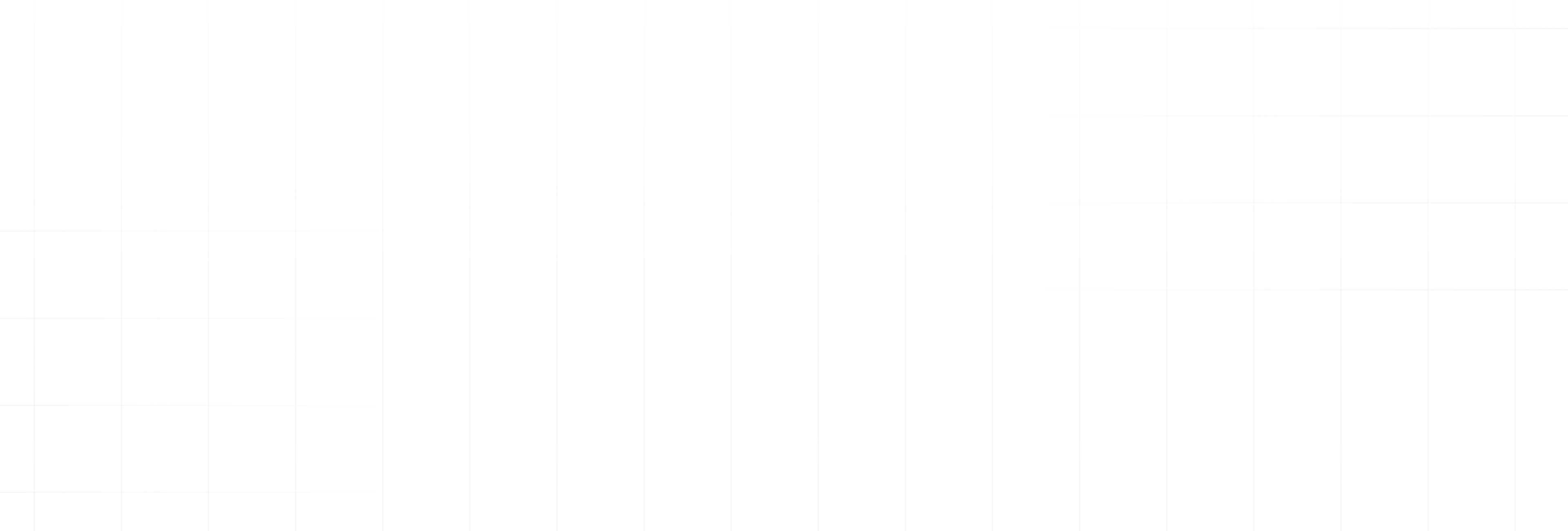
Why Settle for Mediocre When You Can Build Exceptional? React Native is an open-source framework developed by Facebook that allows developers to build mobile applications using JavaScript and React, enabling the creation of apps that work seamlessly on iOS and Android platforms. By utilizing a single codebase, React Native bridges the gap between web and mobile development, offering a cost-effective and efficient solution for cross-platform app creation.
Why React Native State Management is Crucial? The Backbone of Scalable and Maintainable Apps.
State management is pivotal in any application, especially those built with React Native. State refers to the data that determines the behavior and appearance of a component at any given time. Effective state management ensures that your app remains responsive, predictable, and scalable, even as you add new features and functionalities.
In any React Native application, managing state is not just a technical necessity—it's the backbone of the app's behavior and user experience. As applications grow more complex, keeping track of the state, which represents data that determines how components render and behave, becomes crucial.
Key State Management Libraries in React Native
React Native provides several popular state management libraries with strengths and use cases. Here's an overview of the most commonly used ones:
1. Redux: Structured State Management
Redux is a robust solution for managing state, particularly in large applications where data flow can become intricate. Redux provides clarity and consistency by centralizing the state in a single store and controlling its updates through defined actions. This makes it easier to debug issues and understand how data moves through your app, making it a go-to choice for many developers dealing with complex state management needs.
Example:
2. Context API: Simple Yet Effective
The Context API, built into React, is ideal for scenarios where you need to share data across multiple components without the overhead of prop drilling. It's particularly useful for managing global app-wide data, like user preferences or themes. While it’s simpler than other state management libraries, the Context API shines in applications where the state isn’t overly complex but still needs to be accessible throughout the component tree.
3. Recoil: Modern and Flexible
Recoil offers a fresh take on state management, focusing on simplicity and flexibility. It introduces concepts like atoms and selectors, allowing fine-grained control over your app’s state. Recoil is designed to minimize boilerplate while providing powerful tools for managing global and derived states. It is a strong choice for developers who want a modern, straightforward approach to state management.
export default App;
Each of these libraries offers a different way to handle state in React Native, from the structured approach of Redux to the simplicity of the Context API and the modern flexibility of Recoil. The best choice depends on your specific needs, the complexity of your application, and how you prefer to manage your app's state.
Role of State in React Native Applications
State in React Native determines how components behave and display at any given time. The dynamic data drives your app, ensuring user interactions lead to the correct outcomes. Proper state management is key to maintaining a responsive and reliable app, especially as your application grows in complexity.
How to Choose the Right State Management Tool for Your Project
Choosing the right state management tool can make or break your project’s success. It’s not just about picking a popular option; it’s about finding the tool that best fits the unique demands of your application. Whether your app is simple or complex, your team is seasoned with certain tools or venturing into new territory, the decision should align with your current needs and future growth. Here's how to navigate this critical choice effectively:
1. Assess Your Application’s Complexity
Simpler tools like the Context API might be sufficient for smaller applications or projects with straightforward data flows. However, as your app scales and the state becomes more intricate, tools like Redux or Recoil offer better control and structure.
2. Consider Your Team’s Expertise
If your team is already familiar with a particular tool, leveraging that knowledge can save time and effort. For instance, if your team has experience with Redux, it may be a more efficient choice, even if a more straightforward tool could theoretically handle the project.
3. Evaluate Performance Needs
Some state management solutions are more efficient than others, especially when dealing with large amounts of data or high-frequency updates. Consider the performance implications of each tool, mainly if your app handles complex, real-time interactions.
4. Look at Community Support and Ecosystem
Tools like Redux have extensive documentation, community support, and various middleware and plugins. This ecosystem can be a significant advantage when solving unique problems or extending your app's functionality.
By carefully weighing these factors, you can choose a state management tool that meets your project’s current needs and supports future growth and complexity.
Factors to Consider when Choosing the Right State Management Library
Selecting the perfect state management library for your React Native project is not a one-size-fits-all decision. The right choice hinges on understanding the specific demands of your application and how they align with the strengths of each tool. Choose a state management library that not only fits your project today but also supports its growth and evolution in the future. Here’s what you need to consider
Application Complexity
The complexity of your app should be the first factor guiding your decision. For straightforward apps with limited state management needs, simpler solutions like the Context API can be effective and easy to implement. However, as your app’s architecture becomes more intricate, you’ll need a more robust solution like Redux or Recoil to manage the state efficiently across multiple components and layers.
Team Expertise and Experience
Your team’s familiarity with a particular state management tool can significantly influence decisions. For example, if your developers are well-versed in Redux, it might make sense to stick with it, as it allows the team to hit the ground running. Conversely, if your team is exploring new tools, opting for something with a gentle learning curve like Recoil might be a better choice to avoid steep onboarding times.
Scalability and Performance
Consider how your application will grow over time. Will it require handling large datasets or complex user interactions? If so, your state management library's scalability and performance characteristics become critical. With its predictable state and middleware options, libraries like Redux are well-suited for applications anticipating significant scaling.
Community Support and Ecosystem
Finally, the community around a state management library can be a game-changer. Tools with active communities, extensive documentation, and a robust ecosystem of plugins and middleware can save you time and headaches. Redux, for example, has a vast ecosystem that can provide solutions to almost any challenge you encounter.
Best Practices for State Management for React Native Applications
Effective state management is essential for keeping your React Native application scalable, maintainable, and responsive. By following a few key best practices, you can manage state more efficiently, leading to better performance and a cleaner codebase:
- Keep State as Local as Possible: Avoid making the state global unless necessary. Localizing state to the smallest possible component or module reduces complexity and makes your application easier to debug and maintain. Global state should be reserved for data that needs to be shared across multiple components.
- Use Immutable Data Structures: Immutability ensures that state changes are predictable and traceable, reducing the likelihood of bugs caused by unintended side effects. Libraries like Redux promote immutability by design, helping you maintain control over your application's state.
- Minimize State Duplication: Duplicating state across different parts of your application can lead to inconsistencies. Instead, derive state from existing data whenever possible, keeping your logic clean and reducing the risk of errors.
- Centralize Complex State Logic: For complex state transitions, centralize the logic in a single location, such as a reducer in Redux or a custom hook in React. This approach simplifies your state logic's management, testing, and debugging.
- Leverage Selectors for Derived State: Use selectors or similar functions to compute the derived state, which depends on other state values. This practice helps keep your components lean and ensures they only re-render when necessary, enhancing performance.
- Optimize Performance with Memoization: Implement memorization techniques, such as React’s useMemo and useCallback, to prevent unnecessary re-renders, especially when dealing with expensive computations or frequent state updates.
- Consistently Name Actions and State Variables: Maintain consistent naming conventions for actions and state variables to enhance code readability and maintainability, making it easier for team members to understand the data flow.
- Test State Logic Thoroughly: Given its critical role in application behavior, thoroughly testing state logic is vital. Unit tests for reducers, actions, and stateful components can help catch potential issues early, ensuring your state management is robust and reliable.
How to Optimize State Management Performance in React Native
Optimizing state management performance in React Native is key to ensuring your app runs smoothly, especially as it grows in complexity. Here are some strategies to help you get the most out of your state management setup. By implementing these strategies, you can significantly improve the performance of your React Native app’s state management.
Avoid Unnecessary Re-renders
Re-renders can significantly impact performance, especially in large applications. Use techniques like React’s useMemo and useCallback to memoize expensive calculations and functions. This ensures that components only re-render when necessary, reducing the load on your application.
Split State into Smaller, Manageable Units
Large, monolithic state objects can slow down your app and make debugging more difficult. Instead, break down the state into smaller, more manageable units. This not only enhances performance but also makes your code easier to maintain.
Use Selectors Wisely
Selectors can help derive and memoize state, ensuring that your components access only the data they need. Libraries like Recoil use selectors to efficiently manage derived state, preventing unnecessary re-renders and improving overall performance.
Optimize Data Fetching
Avoid fetching data that isn't needed immediately. Instead, use lazy loading or caching to manage data more effectively. This reduces the state your app needs to manage at any given time, improving performance.
Batch State Updates
Whenever possible, batch multiple state updates into a single update. React automatically batches updates in event handlers, but you can optimize performance by grouping related state changes together in other parts of your application.
Minimize Global State
A global state can be convenient, but it often leads to performance bottlenecks when too many components depend on it. Limit the use of global state and keep as much state local to components as possible, which reduces the risk of unnecessary re-renders.
Leverage Asynchronous State Management
Consider using asynchronous state management techniques for operations that don’t need to be handled immediately. Tools like Redux Thunk or Redux Saga allow you to handle side effects outside the main thread, preventing your UI from freezing during complex operations.
Profile and Monitor Performance
Regularly profile your application using React DevTools or the React Native Profiler to identify performance issues. Monitoring allows you to pinpoint which components are causing slowdowns and optimize accordingly.
Top 10 Recommended React Native State Management Libraries for Seamless User Experience
State management is crucial to developing React Native applications, especially as the app's complexity increases. The right state management library can significantly affect how data flows through your app and how easily you can manage and scale it.
Here’s a look at the top 10 state management libraries that are highly recommended for ensuring a seamless user experience:
- Redux: Redux is the React ecosystem's most popular state management library. It provides a single source of truth for the application state and enables predictable state transitions. Redux is well-suited for large-scale applications where maintaining a consistent state across various components is critical.
- Recoil: Recoil is a modern state management library that offers a more straightforward and flexible approach than Redux. It introduces atoms and selectors to manage both global and local state efficiently. Recoil is ideal for applications that require fine-grained control over state and high performance.
- MobX: MobX is known for its simplicity and ease of use. It uses observables to track state changes and update the UI accordingly automatically. MobX is perfect for developers who prefer an intuitive and less verbose approach to state management, making it a good fit for medium to large applications.
- Context API: The Context API is a built-in feature of React that allows developers to pass data through the component tree without prop drilling. While not as powerful as Redux or Recoil, it's sufficient for managing state in smaller applications or specific parts of larger applications where simplicity is key.
- Zustand: Zustand is a small, fast, and scalable state management library that provides a straightforward API. It’s built on top of React's Context API but offers a more optimized and performant way to manage global state. Zustand is ideal for developers who want minimal boilerplate and maximum performance.
- Jotai: Jotai is inspired by Recoil and provides a minimalistic and flexible approach to state management. It’s designed to work seamlessly with React and React Native, offering atomic state management with a clean and simple API. Jotai is great for applications that need both simplicity and performance.
- Redux Toolkit: Redux Toolkit is an official, opinionated toolset for Redux that simplifies the process of setting up and managing state. It includes utilities for creating slices of state, writing reducers, and managing middleware. Redux Toolkit is perfect for developers who appreciate Redux but want a more streamlined experience.
- Apollo Client: Apollo Client is primarily used to manage state in GraphQL applications. It offers features like caching, query management, and local state management. Apollo Client is ideal for applications that rely heavily on GraphQL for data fetching and need a powerful tool to manage both remote and local state.
- Rematch: Rematch is a Redux wrapper that simplifies the Redux setup process while retaining all of its benefits. It reduces boilerplate and provides a more modern API, making state management easier to implement. Rematch is a good choice for developers who like Redux but want a cleaner and more efficient workflow.
- XState: XState is a state management library based on finite state machines. It’s excellent for managing complex state logic that involves different states and transitions. XState is particularly useful in scenarios where state transitions are complex and must be handled precisely, such as in multi-step workflows or animations.
Insider Tip:
While the above libraries are all powerful tools in their own right, the best choice depends heavily on your project’s specific needs and your team’s expertise.
Choosing the right state management library can dramatically impact the efficiency of your development process and the performance of your application. Before making a decision, evaluate your project's unique demands and strengths. For example:
- If your team is well-versed in Redux, Redux or Redux Toolkit would be a natural choice, offering a familiar environment with added benefits.
- For projects with complex state transitions, XState provides the precision and clarity needed to manage intricate state logic effectively.
- Recoil or Context API might be the best starting point for those new to state management due to their simplicity and ease of use.
Advanced Techniques for State Management in React Native
As your React Native app grows, advanced state management techniques are essential for maintaining performance and scalability. Here are some key strategies:
Handling Complex Application States
Libraries like Redux or MobX offer structured solutions for apps with intricate state dependencies. They centralize state management, ensuring consistency across the application. XState is also an excellent option for modeling complex state transitions, especially in applications requiring precise control over state changes.
The Role of Hooks in State Management
React Hooks, such as useState
, useReducer
, and useContext
, provide a flexible approach to managing state in functional components. Custom hooks can encapsulate complex state logic, promoting code reuse and keeping components clean and focused.
Enhancing State Access Control with Context API
The Context API, combined with hooks like useContext
, allows for refined state access control. By using provider components to manage state updates, you can limit access to specific parts of the state, reducing the risk of unintended changes and improving maintainability.
Leveraging Middleware for Complex State Management
Managing side effects such as API calls, logging, and other asynchronous actions can become cumbersome in more complex applications. Middleware, especially in libraries like Redux, offers a powerful way to handle these side effects in a clean and scalable manner. Middleware such as redux-thunk
or redux-saga
allows you to handle asynchronous operations in a testable and maintainable way, keeping your business logic decoupled from your UI logic.
Conclusion
Mastering state management in React Native is crucial for building robust and scalable apps. The right approach depends on your app’s complexity and your team’s expertise, whether you choose simple tools like the Context API or more advanced libraries like Redux and XState. By aligning your state management strategy with your project’s needs, you can create a seamless, responsive user experience that stands the test of time.
Ultimately, the goal of mastering state management isn’t just to manage data more effectively—it’s to build applications that stand out in functionality and user experience.
Book a Discovery Call.
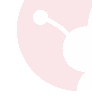