Enhancing Animation Performance in React Native with Reanimated
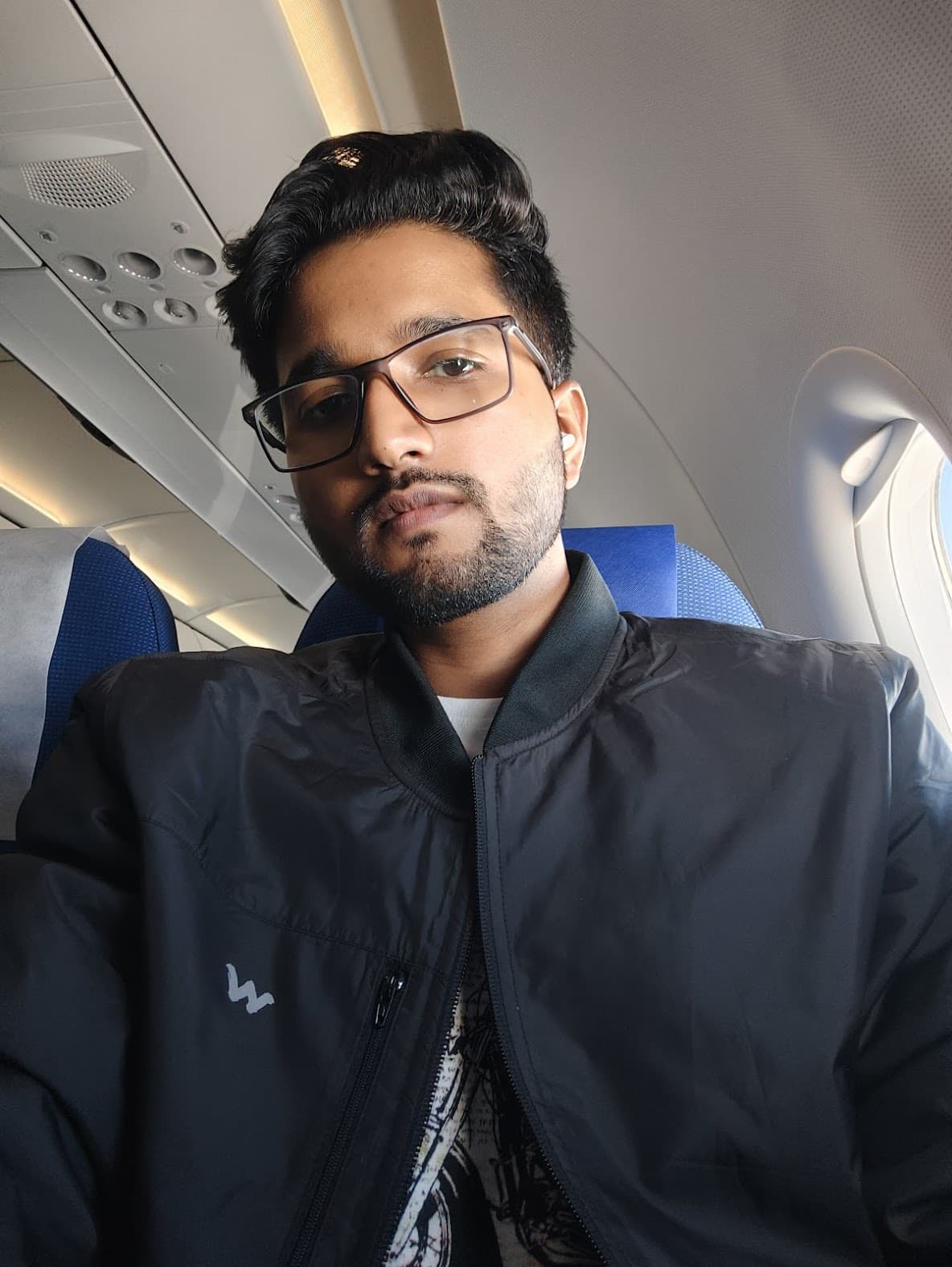
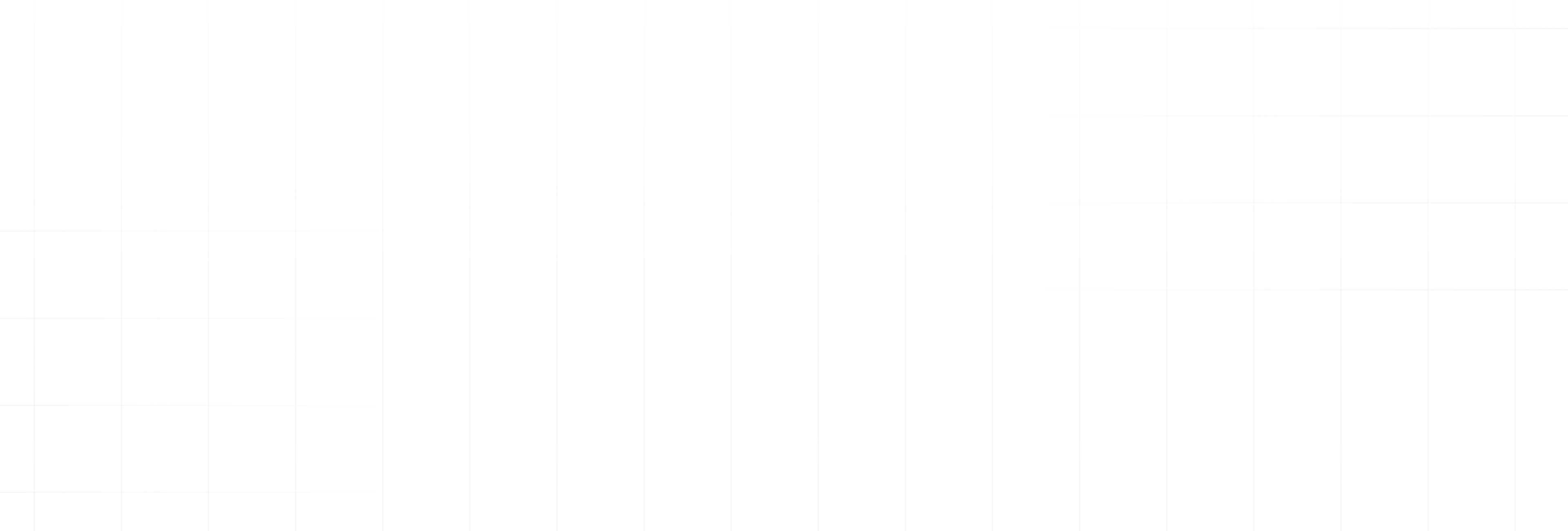
React Native is a popular framework for building cross-platform mobile applications. One of its powerful features is the ability to create smooth and performant animations. However, optimizing animation performance can be challenging. In this post, we’ll explore how to supercharge your animations in React Native using the Reanimated library and custom Babel plugins.
Get ready to make your animations smoother than butter on a hot pancake!
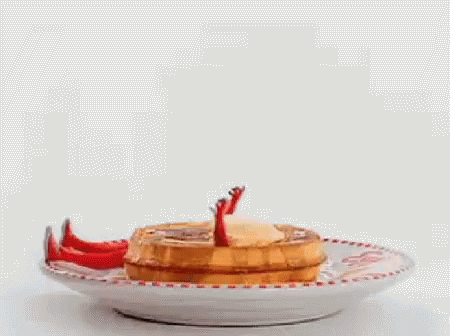
The Challenge
By default, when initializing any animated value using the useSharedValue hook in Reanimated, the native driver is set to false. Don't believe us? Check out the source code. This can lead to suboptimal performance, especially for complex animations. Additionally, we encountered specific performance issues on Android when animating transform properties, causing animations to lag and flicker.
Lagging animations? Ain’t nobody got time for that!
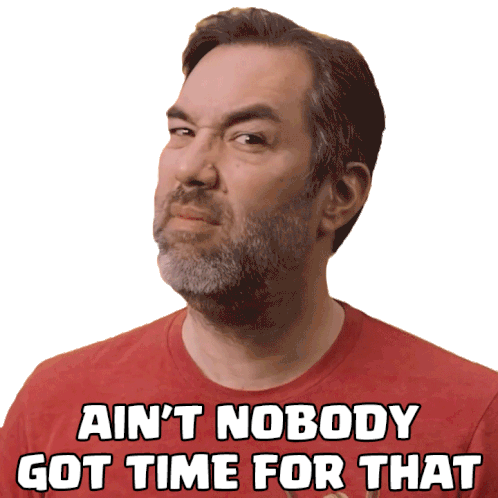
We developed a custom Babel plugin to ensure that the native driver is always set to true when using useSharedValue. This plugin automatically modifies the arguments passed to useSharedValue, enabling the native driver by default.
Here’s the code for the custom Babel plugin:
Solution 2: Handling Transform Properties on Android
For Android, we found that animations using the style's transform properties caused lagging and flickering. To overcome this, we opted to animate using deprecated props. Although the reason for the improved performance is unclear, the official documentation notes these deprecated props here.
Why go deprecated? Because sometimes, the old ways are the best ways! 😉
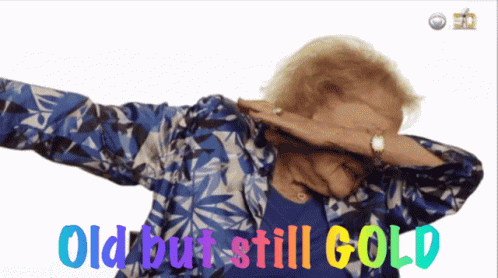
To automate this process, we wrote another custom Babel plugin that checks for the usage of transform properties in the useAnimatedStyle hook and converts them to deprecated props if the platform is Android.
Here’s the code for the custom Babel plugin:
A Brief Overview of Babel and AST
Babel
Babel is a popular JavaScript compiler that helps you use next-generation JavaScript, today. It allows developers to write modern JavaScript code that can run on older browsers or environments by transforming it into a backward-compatible version. Babel also provides powerful plugins and presets that enable a wide range of functionalities, including code optimization and transformation.
Think of Babel as your code’s personal translator, ensuring it speaks every browser’s language!
Abstract Syntax Tree (AST)
An Abstract Syntax Tree (AST) is a tree representation of the structure of source code. It breaks down the code into its syntactical elements, such as statements, expressions, and declarations. This structure allows tools like Babel to analyze and transform the code. By traversing the AST, Babel plugins can modify specific parts of the code, enabling powerful transformations and optimizations.
We are going to use babel to our advantage here to manipulate the AST as per our needs.
How It Works
useSharedValuePlugin
The plugin works by traversing the abstract syntax tree (AST) of your code and looking for calls to useSharedValue. When it finds one, it checks the number of arguments passed. If only one argument is present, the plugin adds a second argument, setting the native driver to true.
useAnimatedStylePlugin
This plugin detects the usage of transform properties in the useAnimatedStyle hook and converts them to deprecated props for better performance on Android. It traverses the AST to find transform properties and replaces them with their deprecated equivalents.
Implementation Steps
- Install Babel and the Plugins: First, ensure that you have Babel installed in your project. Then, add the custom plugins to your Babel configuration.
- Configure Babel: Add the custom plugins to your Babel configuration file (e.g., babel.config.js).
- Add the Plugins: Save the custom plugin codes in the babel.config.js file or you can save them in separate files as well.
Benefits
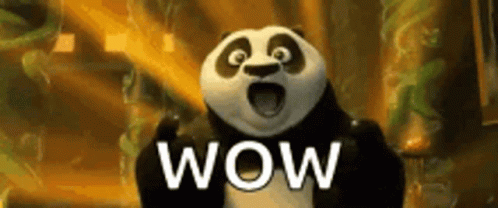
By automatically setting the native driver to true and converting transform properties to deprecated props on Android, these plugins ensure that your animations run more smoothly and efficiently. This is particularly beneficial for complex animations that can be resource-intensive.
Your users will be like: “Wow, how did they make these animations so smooth?”
Conclusion
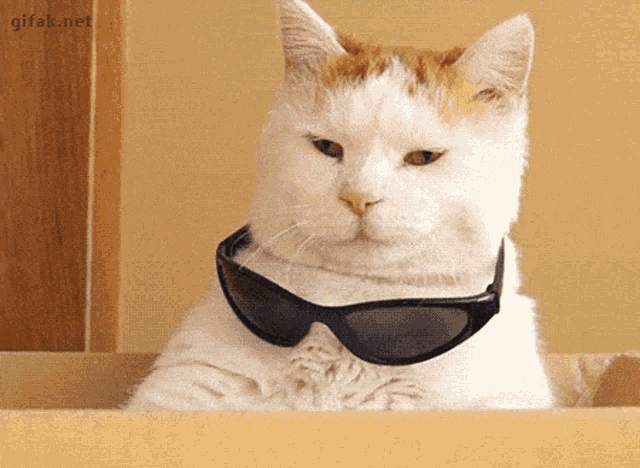
Optimizing animation performance in React Native can significantly enhance the user experience. By using custom Babel plugins to handle useSharedValue and transform properties, you can achieve smoother and more performant animations. Feel free to try out these approaches in your projects and experience the performance improvements firsthand.
Happy coding, and may your animations be ever so smooth!
Book a Discovery Call.
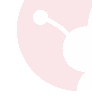