Understanding and Implementing the Custom React Hook for Network State Monitoring
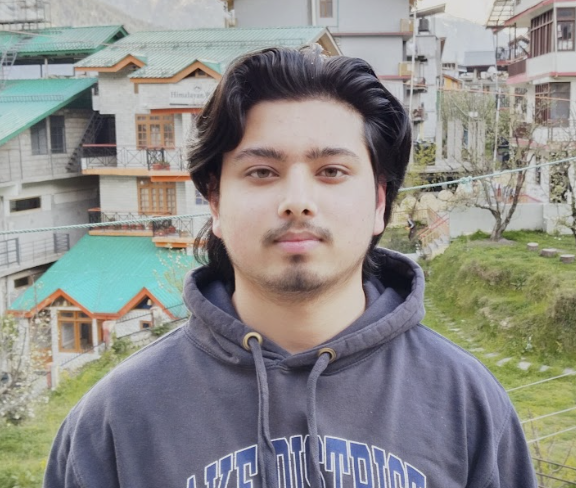
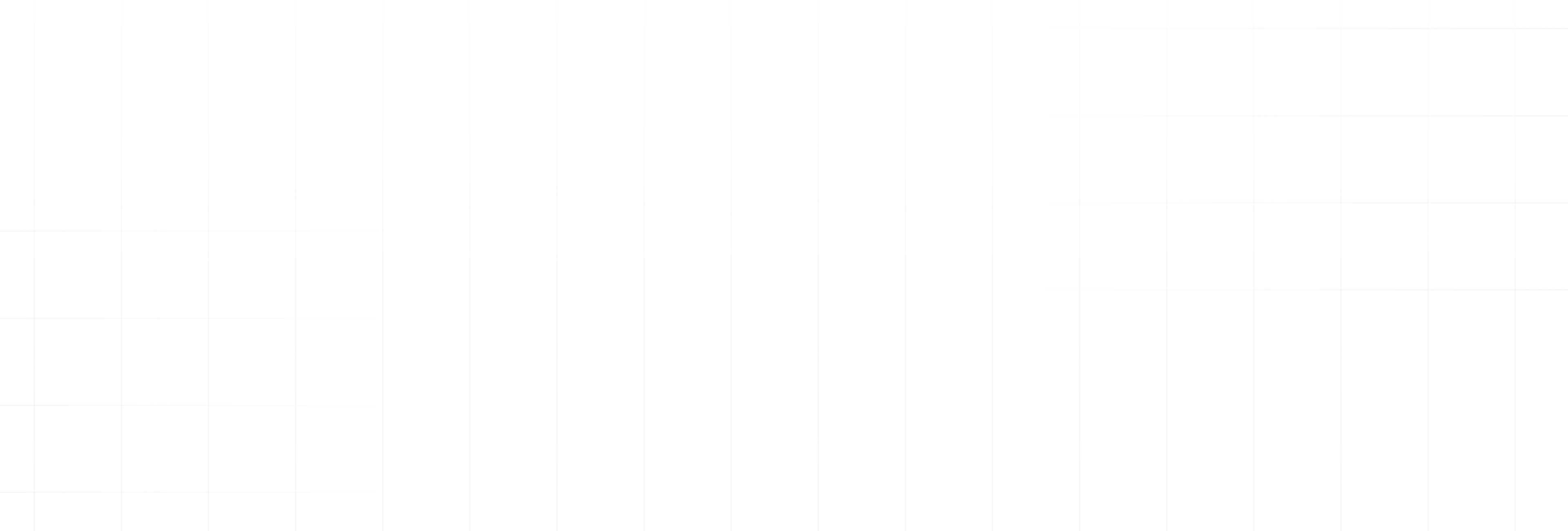
What is the useNetworkState Hook?
The useNetworkState
hook is a custom React hook designed to monitor the user's online/offline status and provide relevant information within your React components. It utilizes the browser's navigator.onLine
property to determine the current network state and maintains its own state within the hook using the useState
hook.
Breakdown of the Code
Let us dissect the individual parts of the useNetworkState
hook:
- State Definition:
- The hook starts by defining a type alias named
NetworkState
to represent the structure of the data it will manage. This type includes two properties:online
: A boolean value indicating the current online status (true if online, false if offline).error
: An optional string value to store any potential error messages during state updates.
- The
useState
hook is used to create a state variable namednetworkState
with the initial value set based on the currentnavigator.onLine
property. This ensures the hook reflects the initial network state upon first render.
- The hook starts by defining a type alias named
updateNetworkState
Function:- This function, declared using
useCallback
, is responsible for updating thenetworkState
based on the current online status. It performs the following actions:- Attempts to update the
online
property of the state with the currentnavigator.onLine
value. - If any error occurs during the update, it logs the error message and sets the
error
property of the state with a generic error message.
- Attempts to update the
- This function, declared using
useEffect
Hook:- This hook manages the lifecycle of event listeners and state updates related to network changes. It performs the following actions:
- Adds event listeners for both "online" and "offline" events to the
window
object, triggering theupdateNetworkState
function whenever the network status changes. - Calls the
updateNetworkState
function immediately after theuseEffect
hook runs to ensure the state reflects the initial network status. - In the cleanup function returned by
useEffect
, removes the event listeners when the component unmounts to prevent memory leaks and unnecessary event handling.
- Adds event listeners for both "online" and "offline" events to the
- This hook manages the lifecycle of event listeners and state updates related to network changes. It performs the following actions:
- Returning the State:
- The hook simply returns the current value of the
networkState
object, allowing components that use this hook to access theonline
anderror
properties for further processing and UI updates.
- The hook simply returns the current value of the
Using the useNetworkState Hook in Your Components
To utilize the useNetworkState
hook in your React components, follow these steps:
Import the Hook:
JavaScript
import useNetworkState from './path/to/useNetworkState';
Call the Hook and Access the State:
JavaScript
const { online, error } = useNetworkState();
// Use the online and error values in your component's logic and U
I
For example, you can conditionally render different UI elements based on the online
state or display an error message if the error
property is populated.
Conclusion
The useNetworkState
hook demonstrates the power of custom hooks in managing application-specific logic within React components. By encapsulating the network state management and providing a clean interface, this hook simplifies the development process and promotes code reusability. You can further enhance this hook by adding features like debouncing the state updates to avoid excessive re-renders or implementing more specific error handling based on the error types.