Table of Contents
A Beginner's Guide to Front-End Security for Fintech Apps Using ReactJS and React Native
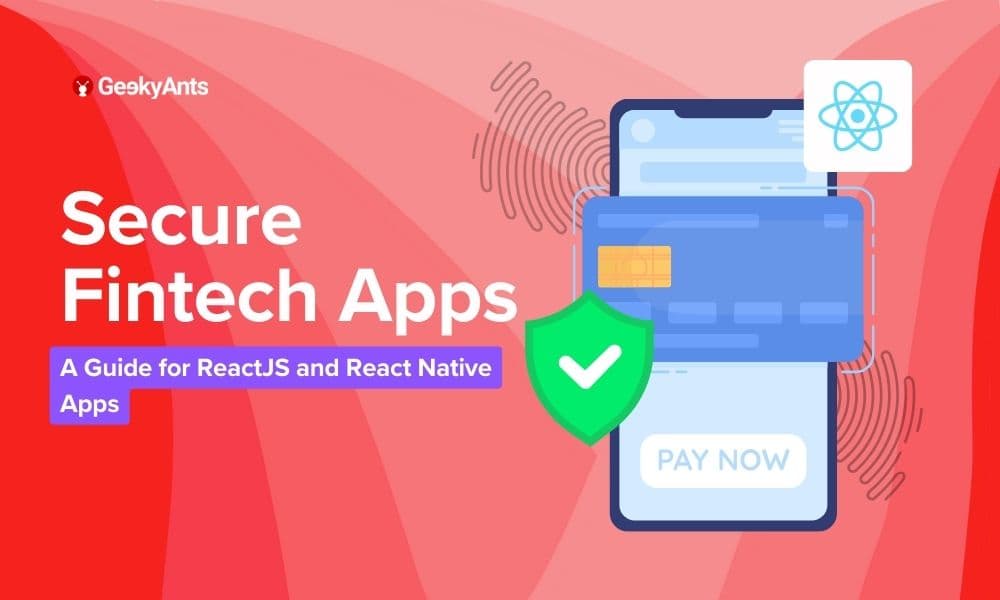
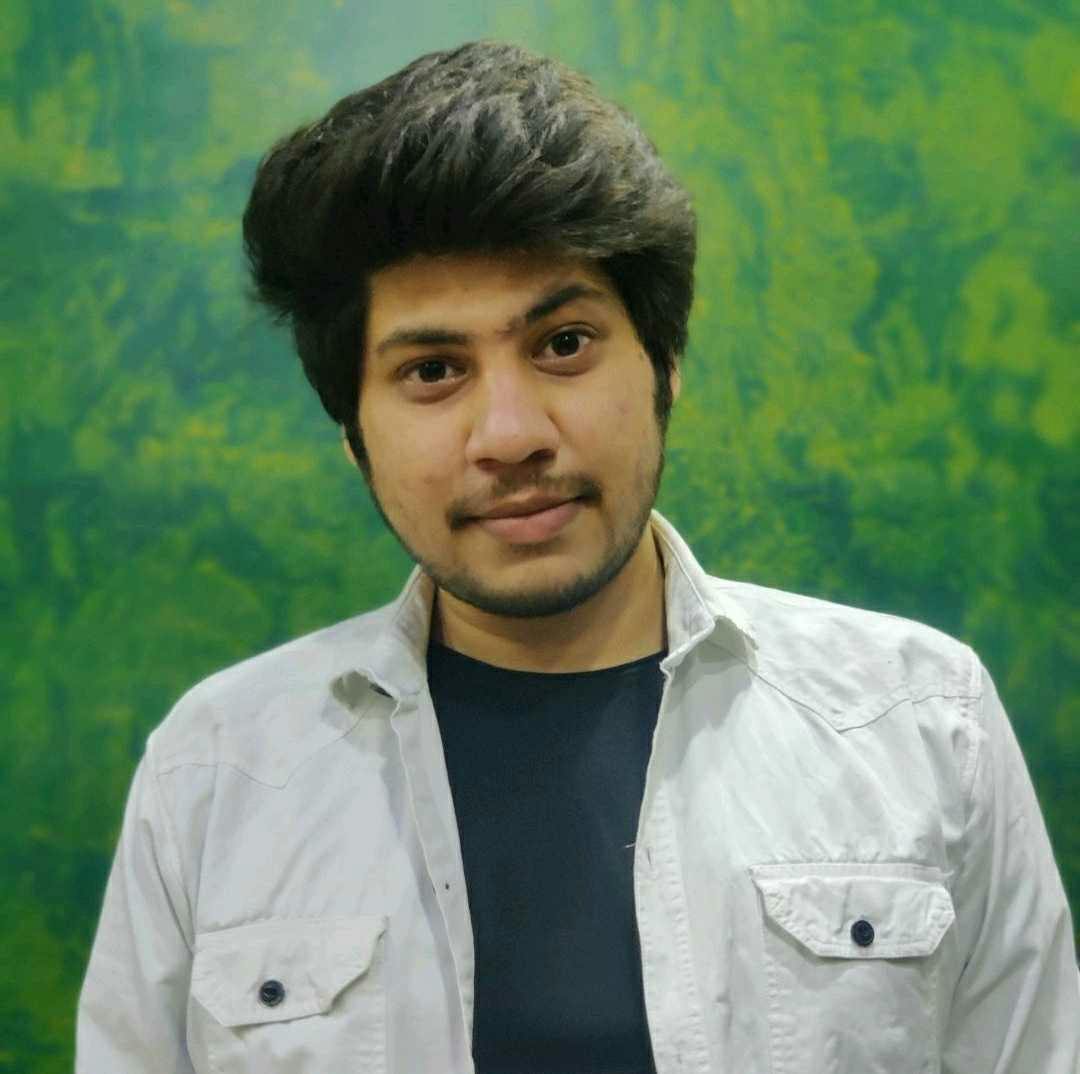
Book a call
With the rapid growth of the fintech industry, projected to reach $699.5 billion by 2030, the demand for secure and reliable fintech applications is higher than ever. As front-end developers, ensuring the security of these applications is a critical task. In this guide, we'll explore essential security practices for fintech app development in ReactJS and React Native, complete with practical code examples. As the fintech industry continues to thrive, ensuring the security of front-end applications becomes increasingly paramount. In this guide, we'll delve into the intricacies of front-end security for fintech apps built with ReactJS and React Native. We'll explore security measures at both the application level and the network layer, along with common security issues faced by fintech applications.
Application-Level Security in Fintech
Application-Level Security in Fintech refers to the measures and practices implemented within fintech applications to ensure the security of sensitive data, protect against unauthorized access, and mitigate the risk of cyber threats. It includes implementing features such as transaction monitoring, user data privacy measures, and masking sensitive data to safeguard financial information and maintain regulatory compliance.
1. Secure Authentication: Multi-Factor Authentication (MFA)
Multi-Factor Authentication (MFA) is a security mechanism that requires users to provide two or more verification factors to gain access to a resource such as an application, online account, or VPN. Instead of just asking for a username and password, MFA requires one or more additional verification factors, which decreases the likelihood of a successful cyber attack. The factors can include something the user knows (password), something the user has (security token, phone), and something the user is (fingerprint, facial recognition).
Implementing MFA adds an extra layer of security by requiring users to provide a second form of identification. This makes it significantly more challenging for unauthorized users to gain access, even if they have obtained the user's password.
ReactJS Example:
Implementing MFA adds an extra layer of security by requiring users to provide a second form of identification.
React Native Example:
2. Secure Input Handling: Preventing XSS
Use React’s built-in mechanisms to prevent Cross-Site Scripting (XSS) attacks by automatically escaping content.
Cross-Site Scripting (XSS) is a type of security vulnerability typically found in web applications. It allows attackers to inject malicious scripts into webpages viewed by other users. These scripts can steal data, hijack user sessions, or perform other malicious actions.
In the examples below, React's built-in mechanisms and best practices help prevent XSS attacks:
- Automatic Escaping: React automatically escapes any content inserted between curly braces
{}
. This means that any HTML tags or JavaScript code included in user input will be rendered as plain text rather than being executed. - Sanitization: In React Native, even though the environment is less prone to XSS due to its native context, sanitizing inputs is a good practice. Using libraries like DOMPurify (or similar) helps ensure that any potentially harmful content is neutralized before being rendered.
Pseudo-code for Both ReactJS and React Native:
React Native Example:
While React Native is less prone to XSS due to its native environment, it's still good practice to sanitize inputs.
3. Role-Based Access Control (RBAC)
What is RBAC?
Role-Based Access Control (RBAC) is a method of regulating access to resources based on the roles of individual users within an organization. In an RBAC system, permissions to perform certain operations are assigned to specific roles rather than to individual users. Users are then assigned particular roles, granting them the associated permissions.
Benefits of RBAC:
- Enhanced Security: By restricting access to sensitive data and operations to only those users who need it, RBAC helps prevent unauthorized access.
- Simplified Management: Managing permissions becomes easier as they are grouped into roles, allowing for more straightforward updates and audits.
- Compliance and Governance: RBAC supports regulatory compliance by ensuring that access controls are consistently applied and documented.
Example Scenario:
- Admin Role: Can view the dashboard and manage users.
- User Role: Can only view the dashboard.
ReactJS Example:
Implementing RBAC restricts access based on user roles, enhancing security.
Explanation of the Code:
- Role Definitions: An object
roles
defines what permissions each role has. - Permission Check: The function
hasAccess
checks if a given role includes a specific permission. - Conditional Rendering: In both examples, the
Dashboard
component renders UI elements conditionally based on the user's role. For example, the "Manage Users" button is only shown if the user has the 'manageUsers' permission.
By using RBAC, we can ensure that only authorized users have access to certain features, thereby enhancing the security and integrity of the application.
4. Handling KYC (Know Your Customer) in Fintech Apps
What is KYC?
Know Your Customer (KYC) is a process used by financial institutions and fintech companies to verify the identity, suitability, and risks involved with maintaining a business relationship. This process helps prevent fraud, money laundering, and other illicit activities by ensuring that customers are who they claim to be.
Why is KYC Important?
- Compliance: Adhering to legal and regulatory requirements to prevent financial crimes.
- Risk Management: Assessing and managing the risks associated with customer relationships.
- Trust Building: Enhancing trust and security by verifying the legitimacy of customers.
- Fraud Prevention: Reducing the likelihood of fraud and identity theft.
KYC Process Steps:
- Customer Identification: Collecting and verifying customer information such as name, address, date of birth, and government-issued ID.
- Customer Due Diligence (CDD): Assessing the risk level of the customer based on the information provided.
- Enhanced Due Diligence (EDD): Conducting more extensive checks for high-risk customers.
- Ongoing Monitoring: Continuously monitoring transactions and updating customer information.
ReactJS Example for KYC:
5. Handling Transaction Monitoring in Fintech
What is Transaction Monitoring?
Transaction monitoring is the process of reviewing, analyzing, and managing financial transactions on a continual basis. This is crucial in fintech for detecting suspicious activities, preventing fraud, complying with regulatory requirements, and ensuring the security and integrity of financial systems.
Why is Transaction Monitoring Important?
- Fraud Detection: Identifying and preventing fraudulent activities such as unauthorized transactions, identity theft, and account takeovers.
- Regulatory Compliance: Ensuring adherence to regulations such as Anti-Money Laundering (AML) and Counter-Terrorism Financing (CTF) laws.
- Risk Management: Assessing and mitigating risks associated with customer transactions and behavior.
- Customer Protection: Safeguarding customers’ assets and financial information.
Steps in Transaction Monitoring:
- Data Collection: Gathering transaction data from various sources such as banking systems, payment gateways, and user accounts.
- Data Analysis: Analyzing transaction data using rules-based systems, machine learning models, and anomaly detection techniques.
- Alert Generation: Generating alerts for suspicious transactions that require further investigation.
- Investigation: Investigating alerts to determine if they represent legitimate activity or fraud.
- Reporting: Reporting confirmed fraudulent activities to regulatory authorities and affected customers.
- Continuous Improvement: Updating monitoring rules and models based on new patterns and regulatory changes.
ReactJS Example for Transaction Monitoring:
Common Transaction Monitoring Techniques:
- Rules-Based Monitoring: Setting predefined rules to flag suspicious transactions, such as high-value transactions or transactions from high-risk countries.
- Statistical Analysis: Using statistical methods to identify anomalies in transaction patterns.
- Machine Learning Models: Training machine learning models to detect fraud based on historical data and patterns.
- Behavioral Analysis: Monitoring changes in user behavior that could indicate fraudulent activity.
- Network Analysis: Analyzing the relationships between entities to detect money laundering or fraud rings.
Challenges in Transaction Monitoring:
- Data Volume: Handling large volumes of transaction data in real-time.
- False Positives: Reducing the number of false positives to avoid unnecessary investigations.
- Regulatory Changes: Keeping up with changing regulatory requirements and updating monitoring systems accordingly.
- Integration: Integrating transaction monitoring systems with existing IT infrastructure and financial systems.
- Privacy Concerns: Ensuring customer privacy while monitoring transactions.
By implementing robust transaction monitoring systems and leveraging advanced techniques, fintech companies can enhance security, comply with regulations, and protect their customers from fraud and other financial crimes.
6. Handling User Monitoring in Fintech
What is User Monitoring?
User monitoring involves tracking and analyzing user activities within an application to ensure security, compliance, and enhance user experience. In fintech, user monitoring is crucial for detecting fraudulent activities, ensuring compliance with regulatory requirements, and improving the overall security posture of the platform.
Why is User Monitoring Important?
- Fraud Detection: Identifying suspicious behavior that may indicate fraudulent activities.
- Compliance: Ensuring adherence to regulatory requirements such as GDPR, AML, and CTF.
- User Behavior Analysis: Understanding user behavior to improve user experience and service offerings.
- Incident Response: Quickly responding to security incidents by monitoring and analyzing user activities.
- Access Control: Ensuring users have appropriate access levels and detecting unauthorized access attempts.
Key Aspects of User Monitoring:
- Activity Logging: Recording user activities such as logins, transactions, and profile changes.
- Behavioral Analytics: Analyzing user behavior to detect anomalies.
- Real-Time Alerts: Generating alerts for suspicious activities.
- Access Management: Monitoring and managing user access to sensitive information and functionalities.
- Compliance Reporting: Generating reports to demonstrate compliance with regulatory requirements.
ReactJS Example for User Monitoring:
Common Techniques for User Monitoring:
- Log Management: Collecting and managing logs of user activities.
- User Behavior Analytics (UBA): Using machine learning to analyze user behavior and detect anomalies.
- Security Information and Event Management (SIEM): Aggregating and analyzing logs from different sources to detect and respond to security incidents.
- Multi-Factor Authentication (MFA): Adding an extra layer of security by requiring multiple forms of verification.
- Access Control and Management: Monitoring user access and ensuring it aligns with their roles and responsibilities.
- Alerting and Notifications: Setting up alerts for suspicious activities and notifying relevant personnel for quick action.
Challenges in User Monitoring:
- Data Volume: Handling large volumes of user activity data in real-time.
- Privacy Concerns: Ensuring user monitoring does not violate privacy regulations or user trust.
- False Positives: Minimizing false positives to avoid alert fatigue and unnecessary investigations.
- Integration: Integrating monitoring tools with existing systems and applications.
- Scalability: Ensuring monitoring systems can scale with the growing number of users and transactions.
Best Practices for Effective User Monitoring:
- Define Clear Policies: Establish clear monitoring policies that comply with legal and regulatory requirements.
- Use Advanced Analytics: Leverage machine learning and behavioral analytics to detect anomalies.
- Regularly Update Monitoring Rules: Keep monitoring rules and models up-to-date to adapt to new threats and changes in user behavior.
- Ensure Data Security: Protect monitoring data with encryption and access controls.
- Provide Transparent Communication: Inform users about monitoring practices and ensure transparency to maintain trust.
By implementing robust user monitoring systems and adhering to best practices, fintech companies can enhance their security, ensure compliance, and provide a safer environment for their users.
7. Handling User Data Privacy in Fintech
What is User Data Privacy?
User data privacy refers to the practice of ensuring that personal information shared by users is handled securely and used only in ways that users have consented to. In the fintech industry, protecting user data privacy is paramount due to the sensitive nature of financial information.
Why is User Data Privacy Important?
- Compliance: Adhering to regulations like GDPR (General Data Protection Regulation), CCPA (California Consumer Privacy Act), and other data protection laws.
- Trust: Building and maintaining user trust by demonstrating a commitment to protecting their personal information.
- Risk Management: Minimizing the risk of data breaches and their associated financial and reputational damage.
- User Rights: Ensuring users have control over their personal data, including the right to access, correct, and delete their information.
Key Principles of User Data Privacy:
- Data Minimization: Collect only the data that is necessary for the intended purpose.
- Consent: Obtain explicit consent from users before collecting, using, or sharing their data.
- Transparency: Clearly inform users about what data is being collected, how it will be used, and who it will be shared with.
- Security: Implement robust security measures to protect user data from unauthorized access and breaches.
- User Rights: Respect and facilitate users' rights to access, correct, and delete their personal data.
ReactJS Example for Handling User Data Privacy:
Common Techniques for Handling User Data Privacy:
- Data Encryption: Encrypting data at rest and in transit to protect it from unauthorized access.
- Access Controls: Implementing strict access controls to ensure only authorized personnel can access sensitive data.
- Data Anonymization: Anonymizing data to protect user identities while allowing analysis.
- User Consent Management: Keeping detailed records of user consent for data collection and usage.
- Regular Audits: Conducting regular audits to ensure compliance with data privacy regulations.
- Data Retention Policies: Establishing and enforcing data retention policies to ensure data is not kept longer than necessary.
Challenges in Handling User Data Privacy:
- Regulatory Compliance: Keeping up with and ensuring compliance with evolving data privacy regulations.
- Data Breaches: Preventing and responding to data breaches.
- User Consent: Effectively managing and documenting user consent.
- Data Minimization: Balancing the need for data with the principle of data minimization.
- User Rights: Facilitating user rights such as data access, correction, and deletion.
Best Practices for User Data Privacy:
- Transparent Privacy Policies: Clearly communicate privacy policies to users.
- Robust Security Measures: Implement advanced security measures to protect user data.
- User Education: Educate users about data privacy and security.
- Regular Training: Provide regular training for employees on data privacy and security practices.
- Incident Response Plan: Develop and maintain a robust incident response plan for data breaches.
By implementing comprehensive user data privacy practices, fintech companies can protect sensitive user information, comply with regulatory requirements, and build trust with their customers.
Network-Layer Security in Fintech
Network-Layer Security in Fintech refers to the measures taken to secure the communication channels and data transmission between different components of fintech applications. This includes encryption, authentication, access control, and other techniques to protect against unauthorized access, data breaches, and cyber attacks.
1. Secure Communication: Using HTTPS
Ensuring secure communication between your app and the server is crucial. Always use https://
URLs for API calls.
What is HTTPS?
HTTPS (Hypertext Transfer Protocol Secure) is an extension of HTTP and is used for secure communication over a computer network, primarily the internet. HTTPS encrypts the data sent between the client (e.g., a web browser or mobile app) and the server using the SSL/TLS protocol. This encryption ensures that even if the data is intercepted by a malicious actor, it cannot be read or tampered with.
How Does HTTPS Help Secure the Data on the Network Layer?
- Data Encryption: HTTPS uses SSL/TLS to encrypt the data transmitted between the client and the server. This encryption makes it extremely difficult for attackers to intercept and read the data.
- Data Integrity: HTTPS ensures that the data sent and received has not been altered during transit. Any modification of the data would be detected, providing assurance that the content received is exactly what was sent by the server.
- Authentication: HTTPS uses certificates to verify the identity of the server. This helps ensure that the client is communicating with the legitimate server and not an imposter.
- Privacy and Confidentiality: By encrypting the data, HTTPS helps maintain the privacy and confidentiality of the information being exchanged, protecting sensitive data such as personal information, login credentials, and financial details.
ReactJS Example:
React Native Example:
2. Masking Webpack Mappings in ReactJS and React Native - Release Build
What is Webpack Mapping?
Webpack mapping involves generating source maps that link your minified code back to its original source code. This is crucial for debugging, as it allows developers to see the original source code when an error occurs in the minified code. However, in a production environment, exposing source maps can reveal the application's internal structure and make it easier for attackers to exploit vulnerabilities.
Why is Masking Webpack Mappings Important?
- Security: Prevents attackers from easily understanding and exploiting your code.
- Obfuscation: Makes it more difficult for unauthorized users to reverse-engineer your application.
- Protection of Intellectual Property: Helps protect proprietary algorithms and business logic from being exposed.
Key Principles of Masking Webpack Mappings
- Disable Source Maps in Production: Ensure source maps are not generated or accessible in the production build.
- Obfuscate Code: Use obfuscation techniques to make the code harder to read and understand.
- Minify Code: Minification reduces the size of the code and obfuscates variable names, making it less readable.
ReactJS Example for Masking Webpack Mappings
React Native Example for Masking Webpack Mappings:
Common Techniques for Masking Webpack Mappings
- Source Map Disabling: Configure your build tools to disable source map generation in production.
- Code Obfuscation: Use tools like
javascript-obfuscator
to obfuscate your code. - Environment Configuration: Use environment variables to differentiate between development and production builds.
- CI/CD Integration: Integrate source map handling into your CI/CD pipeline to ensure they are not included in production.
Challenges in Masking Webpack Mappings
- Debugging: Without source maps, debugging production issues can be challenging.
- Performance Overhead: Obfuscation and minification can add overhead to the build process.
- Configuration Complexity: Setting up the build environment correctly to handle different environments can be complex.
Best Practices for Masking Webpack Mappings
- Separate Configurations: Maintain separate Webpack or Metro configurations for development and production.
- Use Environment Variables: Leverage environment variables to control source map generation.
- Automate with CI/CD: Automate the build process with CI/CD tools to ensure consistency.
- Regularly Update Tools: Keep build and obfuscation tools up-to-date to benefit from the latest security improvements.
By effectively masking Webpack mappings in your ReactJS and React Native applications, you can enhance security and protect your code from unauthorized access and reverse-engineering
3. Masking Sensitive Data in Network Tab for ReactJS and React Native - Release Build
What is Masking Sensitive Data?
Masking sensitive data involves hiding or obfuscating sensitive information, such as user credentials, personal identification details, and financial data, to prevent it from being exposed in logs, network requests, or other debug tools. In web and mobile applications, sensitive data can often be exposed in the network tab of developer tools, posing a security risk.
Why is Masking Sensitive Data Important?
- Security: Prevents sensitive information from being exposed to unauthorized parties.
- Compliance: Helps ensure compliance with data protection regulations like GDPR and CCPA.
- User Trust: Maintains user trust by protecting their personal and financial information.
- Risk Management: Reduces the risk of data breaches and their associated consequences.
Key Principles of Masking Sensitive Data
- Encryption: Use encryption to protect data in transit.
- Data Redaction: Redact sensitive information from logs and debug outputs.
- Secure Storage: Store sensitive data securely using industry best practices.
- Access Control: Restrict access to sensitive data to only those who need it.
ReactJS Example for Masking Sensitive Data
In ReactJS, you can use middleware to intercept and mask sensitive data in network requests.
React Native Example for Masking Sensitive Data
In React Native, you can use a similar approach with the fetch
API or an HTTP client like axios
.
Common Techniques for Masking Sensitive Data
- Request/Response Interceptors: Use interceptors to modify requests and responses.
- Encryption: Encrypt sensitive data before sending it over the network.
- Tokenization: Replace sensitive data with tokens that have no exploitable value outside a specific context.
- Environment Configuration: Use environment-specific configurations to control the exposure of sensitive data.
Challenges in Masking Sensitive Data
- Performance Overhead: Encryption and data masking can introduce performance overhead.
- Complexity: Implementing comprehensive data masking solutions can be complex.
- Debugging Difficulty: Masking data can make debugging more challenging for developers.
- Consistency: Ensuring consistent data masking across different parts of the application can be difficult.
Best Practices for Masking Sensitive Data
- Consistent Masking Policies: Implement consistent data masking policies across all environments.
- Use Secure Protocols: Always use HTTPS to encrypt data in transit.
- Regular Audits: Conduct regular security audits to ensure sensitive data is properly masked.
- Educate Developers: Train developers on the importance of data masking and secure coding practices.
- Automate: Use automated tools and scripts to enforce data masking policies during development and deployment.
By implementing effective data masking techniques in your ReactJS and React Native applications, you can enhance security, protect user privacy, and comply with regulatory requirements.
4. Avoiding iframes, innerHTML, and Inline Styling in ReactJS and React Native
What are iframes, innerHTML, and Inline Styling?
- iframes: HTML elements used to embed another HTML page within a current page.
- innerHTML: A property used to insert HTML content directly into the DOM.
- Inline Styling: Adding CSS directly to HTML elements using the
style
attribute.
Why Avoid iframes, innerHTML, and Inline Styling?
- Security Risks: Using iframes and
innerHTML
can introduce security vulnerabilities such as Cross-Site Scripting (XSS) attacks. - Maintainability: Inline styling makes the code harder to maintain and update.
- Performance: Excessive use of iframes and inline styles can lead to performance issues.
- Best Practices: Following best practices in web development promotes better security, maintainability, and performance.
Key Principles for Avoiding iframes, innerHTML, and Inline Styling
- Use React Components: Replace iframes and
innerHTML
with reusable React components. - CSS-in-JS: Use libraries like styled-components or Emotion for styling in ReactJS.
- StyleSheet in React Native: Use StyleSheet API for styling in React Native.
ReactJS Example for Avoiding iframes, innerHTML, and Inline Styling:
React Native Example for Avoiding iframes, innerHTML, and Inline Styling:
Common Techniques for Avoiding iframes, innerHTML, and Inline Styling
- React Components: Build reusable and secure React components instead of using iframes.
- Sanitizing Content: Use libraries like DOMPurify to sanitize any HTML content before rendering.
- CSS-in-JS Libraries: Use styled-components, Emotion, or other CSS-in-JS libraries to handle styling.
- External CSS Files: Manage styles using external CSS files and import them into your components.
Challenges in Avoiding iframes, innerHTML, and Inline Styling
- Learning Curve: Developers may need to learn new libraries and techniques for managing styles and content safely.
- Legacy Code: Refactoring legacy code that relies heavily on iframes,
innerHTML
, and inline styles can be time-consuming. - Consistency: Ensuring consistent styling and content rendering across the application.
Best Practices for Avoiding iframes, innerHTML, and Inline Styling
- Sanitize Inputs: Always sanitize user inputs to prevent XSS attacks.
- Component-based Architecture: Build applications using a component-based architecture to promote reusability and maintainability.
- Centralized Styling: Use centralized styling approaches such as CSS-in-JS or external CSS files.
- Code Reviews: Conduct regular code reviews to ensure best practices are followed.
- Training: Provide training and resources to developers on secure coding practices and modern styling techniques.
By avoiding iframes, innerHTML
, and inline styling, you can enhance the security, maintainability, and performance of your ReactJS and React Native applications.
Common Security Issues in Fintech Apps
- Insecure Data Storage: Storing sensitive data, such as personal information and financial records, in plaintext or inadequately protected databases can lead to data breaches. Ensure all sensitive data is encrypted both in transit and at rest.
- Weak Authentication: Relying solely on username and password authentication without additional security measures such as MFA can make applications vulnerable to brute-force attacks and credential stuffing. Implement strong authentication mechanisms.
- Insufficient Authorization: Failing to properly implement role-based access control (RBAC) can allow users to access resources or perform actions they shouldn't be allowed to. Ensure that each role has the appropriate permissions and that authorization checks are consistently applied.
- Lack of Input Validation: Not validating user inputs can lead to various types of injection attacks, including SQL injection and XSS. Always validate and sanitize inputs on both the client and server sides.
- Insecure API Communication: Using HTTP instead of HTTPS for API communication can expose data to interception and tampering. Always use HTTPS to encrypt data in transit.
- Improper Session Management: Not securely managing user sessions can result in session hijacking. Ensure that session tokens are securely generated, stored, and invalidated after logout or timeout.
- Exposure of Sensitive Data in Logs: Logging sensitive data such as passwords or credit card information can lead to data leaks if logs are compromised. Ensure sensitive data is masked or not logged at all.
- Outdated Dependencies: Using outdated libraries and frameworks can introduce known vulnerabilities. Regularly update dependencies and apply security patches.
- Poor Error Handling: Revealing too much information in error messages can help attackers gain insights into the application's structure. Implement generic error messages for users and detailed logs for developers.
- Inadequate Security Testing: Failing to conduct regular security testing, such as vulnerability scanning and penetration testing, can leave the application susceptible to undetected vulnerabilities. Integrate security testing into the development lifecycle.
By being aware of these common security issues and implementing best practices, fintech apps can enhance their security posture and protect sensitive user data from potential threats.
Conclusion
Ensuring the security of fintech applications is a multifaceted task that requires a combination of robust authentication methods, secure communication, proper input handling, and role-based access control. By implementing these practices in your ReactJS and React Native applications, you can significantly enhance their security and protect sensitive financial data from potential threats.
Dive deep into our research and insights. In our articles and blogs, we explore topics on design, how it relates to development, and impact of various trends to businesses.