Apr 25, 2024
Introducing the useDebounce Hook
Learn how to optimize your React applications with the useDebounce hook. This powerful tool simplifies debouncing, enhancing performance and user experience.
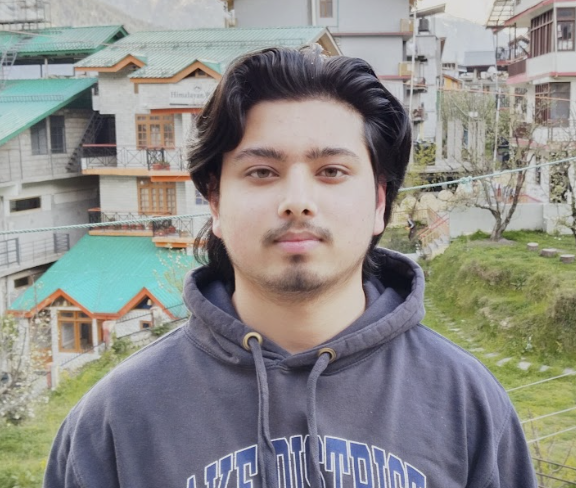
DayaSoftware Engineer - I
Tarun Bhagchand SoniSenior Software Engineer - I
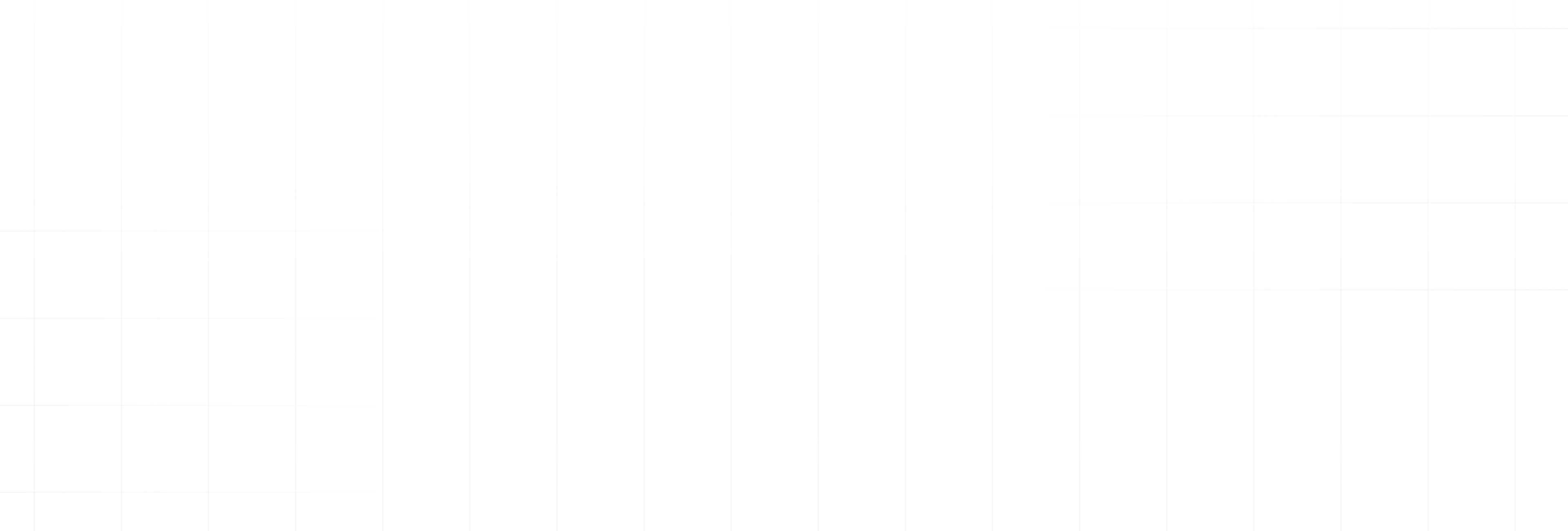
The useDebounce
hook is a functional component that provides a reusable way to implement debouncing in your React applications. It takes two arguments:
value
: This is the value you want to debounce, typically a state variable holding user input (e.g., search term). It can be any data type (T
).delay
(optional): This is the delay in milliseconds after which the debounced value will be updated. It defaults to 500ms (half a second).
Under the Hood: How the Hook Works
Under the Hood: How the Hook Works
- State Management: The hook utilizes the
useState
hook to create two state variables:debouncedValue
: This stores the currently debounced value. It's initialized with the initialvalue
passed to the hook.- (Optional, but recommended)
timeoutId
: This can store the ID of the currently running timeout for better cleanup.
useEffect
for Debouncing Magic: TheuseEffect
hook is responsible for the debouncing logic and runs whenever thevalue
ordelay
dependencies change:- Timeout Creation: Inside the
useEffect
, a timeout is created usingsetTimeout
. This timeout is set to trigger after the specifieddelay
milliseconds. - Debounced Value Update: When the timeout fires, the
setDebouncedValue
function is called. This function updates thedebouncedValue
state with the currentvalue
. - Cleanup Function: A cleanup function is returned by the
useEffect
. This function is crucial for preventing memory leaks. It usesclearTimeout
to clear any pending timeout when the component unmounts or the dependencies change.
- Timeout Creation: Inside the
- Returning the Debounced Value: Finally, the hook returns the current
debouncedValue
. This value will only be updated after the specified delay has passed since the last change in the originalvalue
.
Benefits of Using useDebounce
Benefits of Using useDebounce
- Improved Performance: By using the debounced value for actions like API calls or UI updates, you avoid unnecessary requests and computations, leading to a smoother user experience.
- Optimized Server Interactions: Debouncing helps prevent overloading servers with rapid requests, improving both user and server performance.
- Enhanced User Experience: By delaying actions until user input settles, you reduce flickering or premature updates, making the UI feel more responsive and intuitive.
- Code Reusability: The
useDebounce
hook is a reusable component, promoting better code organization and maintainability across your React projects.
Putting It into Practice: Integrating useDebounce
Putting It into Practice: Integrating useDebounce
Let's see how you can utilize the useDebounce
hook in your React component:
Conclusion
Conclusion
In conclusion, the useDebounce hook is a valuable tool for React developers. It provides a clean and reusable way to implement debouncing, improving the performance and user experience of your applications. By delaying actions until user input settles, you can optimize server interactions, reduce unnecessary computations, and create a more responsive and intuitive UI. Consider incorporating the useDebounce hook into your React projects to enhance their overall quality and efficiency.