Table of Contents
Image Moderation Using Node.js, Amazon Rekognition and SQS
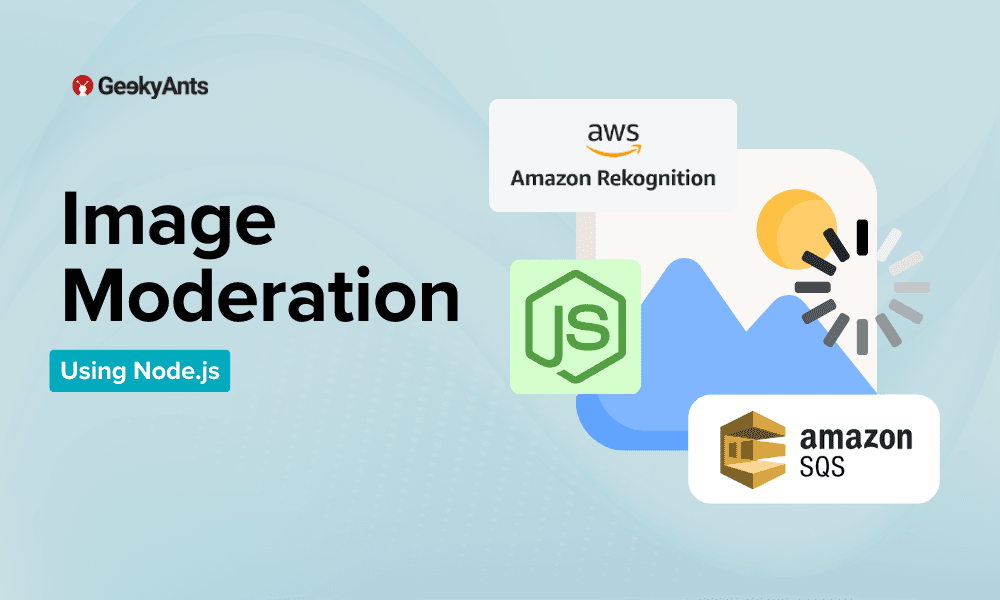
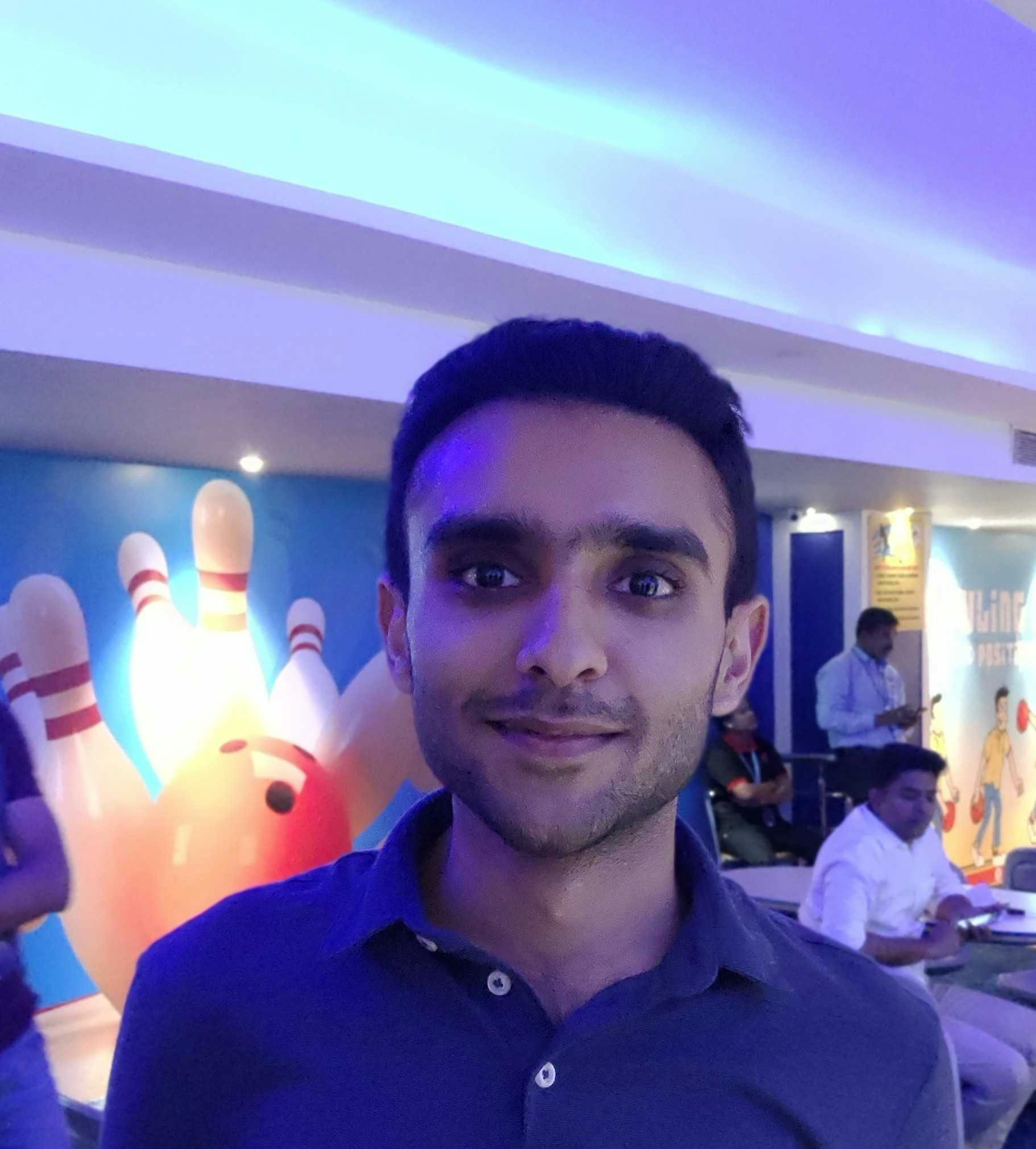
Book a call
This article outlines leveraging Amazon services like Amazon Content Rekognition and Amazon SQS in Node.js. These services enable the detection of NSFW (Not Safe For Work) images in the background and potentially notify or block the user.
The article assumes that the Node.js project and AWS account are set up and that image uploads to storage buckets have been completed separately. We’ll focus on connecting all the AWS services to build the content moderation system.
Ensure you’ve reviewed the prerequisites below and set up a functional working environment.
Prerequisites
Ensure you have the following set up and working:
- A working AWS account.
- Node.js:- I’ve used v20 LTS while writing this article.
- A working Node.js Express project (or any other framework of your choice) that at least has a working API for uploading the image to the storage bucket.
TL;DR
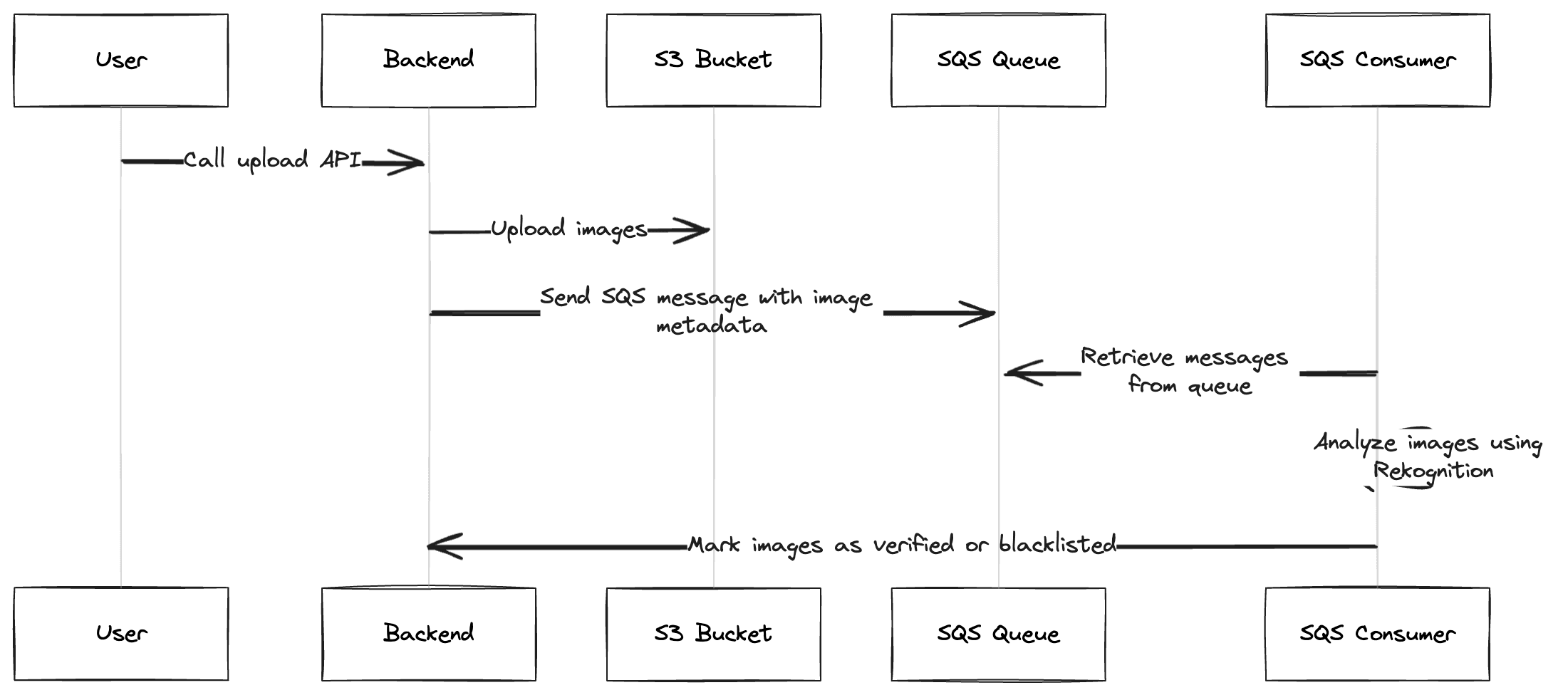
- Initialize the Node.js project with the required dependencies.
- Upload images to the S3 bucket.
- Send messages to the SQS queue to process the uploaded images.
- Retrieve messages from the queue and use Amazon Rekognition to analyze the images.
- Mark images as verified or blacklisted based on the analysis.
A Brief about AWS services used here
You can skip this one if you know the services used here.
Amazon Rekognition: We have used it to perform image moderation, i.e., to get the labels and sub-labels from the image that will tell us the percentage of explicit, violent, and not safe-for-work content in that image.
Amazon SQS: We have used it to separate the image upload and moderation processes. This ensures that the image upload process can be completed quickly, and the moderation can be handled asynchronously in the background.
Amazon S3: S3 is cloud storage service that we’ll use to store the uploaded images. The benefit of using this is Amazon Rekognition can pick up the images from S3 directly using the S3 object key. You can also directly send the image byte data to Rekognition API if you use another storage provider.
Set up Services on the AWS Console
- AWS Credentials: Retrieve your access key and secret key. Go to Summary → Security Credentials to create them and download them.
- S3 Bucket: Follow these steps from the AWS documentation to create the bucket. If you want to display the images directly on our front end, you can set the permission to public, read-only. But we don’t need it for this article.
- SQS Queue: Follow these steps to create a standard queue.
TIP: To prevent unwanted cost, you can also simulate S3 and SQS on local system using tools like localstack, MinIO, and ElasticMQ to test the integration. For Amazon Rekognition, you can only test it with a live AWS account.
Let’s get started with Implementation:
This section will describe the steps in more detail. I’ve also included the example code with all the steps and commented on the lines to explain what they do.
- Install the following dependencies into your Node.js project.
Most of the packages are self-explanatory. The additional package used here is sqs-consumer. SQS is a poll-based queue. Hence, to get the messages, you need to poll them yourself. To ease the development, we’ve used sqs-consumer that provides helper methods to effortlessly set up consumers.
2. Set the following environment variables in your project. Don’t change the key names of AWS variables because keeping the key name the same as below will allow AWS SDK to automatically pick up the correct credentials from the environment.
3. Create the aws services helper class in awsService.ts.
This class creates and maintains the instance of different aws services used in the project.
TIP: If you are simulating some of the services on your local system, don’t forget to pass the custom endoint that points to your localhost, for example, new AwsService.SQS({ ..., endpoint: '<http://localhost>:SERVICE_PORT' })
4. Create a route that uploads the image to the s3 service and queues the image for content moderation. An example snippet uses an express framwork. This does not include request validations and other sanity checks. Make sure to include them in the actual codebase.
- Create the consumer in your entry point i
ndex.ts
file. This will poll the queue and get the message we put in the above code snippet.
TIP 1: Make sure to go through the response of the Rekognition API in detail. It returns the individual percentage of detected labels and sub-labels, which you can use to filter the images more accurately based on your requirements instead of just filtering all of them directly by 50% as I have done above. To go through the list of all labels and sub-labels visit this link:
https://docs.aws.amazon.com/rekognition/latest/dg/moderation.html#moderation-api
TIP 2: Be sure to check out the documentation at https://docs.aws.amazon.com/rekognition/latest/APIReference/API_DetectModerationLabels.html to find out more request parameters and response values to suit your needs.
Conclusion
That’s it! We have demonstrated how to build a content moderation system using Node.js and AWS services. We can also extend this by setting up Custom Moderation Labels that can help enhance the accuracy, tailored more towards your project requirements. Thanks a lot for reading.
Dive deep into our research and insights. In our articles and blogs, we explore topics on design, how it relates to development, and impact of various trends to businesses.