Table of Contents
Xamarin to React Native: A Comprehensive Migration Guide
Author
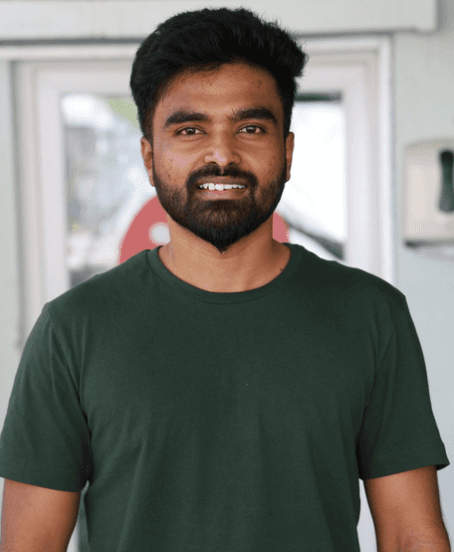
Date
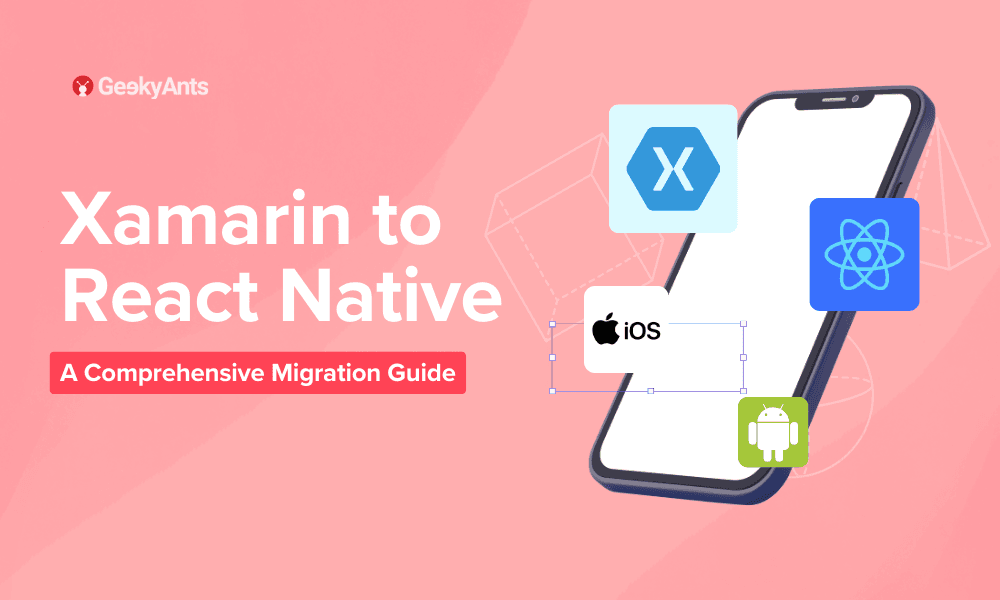
Book a call
In the ever-evolving world of mobile app development, staying current with the latest technologies is crucial. Many developers are at a crossroads, considering migrating their iOS and Android applications from Xamarin to React Native. This comprehensive guide will walk you through converting your Xamarin app to a React Native app, providing insights, best practices, and practical steps to ensure a smooth transition for both platforms.
Xamarin, a popular cross-platform development framework, has been a go-to choice for many developers. However, since Xamarin support has been discontinued and with the rise of React Native, many are considering the switch due to its performance benefits, large community support, and the ability to share code between iOS and Android platforms more efficiently.
Facebook's React Native has gained significant traction in mobile development. It allows developers to build native mobile applications using JavaScript and React, offering a more web-like development experience while still producing truly native apps for iOS and Android.
The reasons for migrating from Xamarin to React Native can vary but often include the following:
- Improved performance and user experience on both platforms
- Larger community and ecosystem of third-party libraries
- More frequent updates and better long-term support
- Easier recruitment of JavaScript developers
- Potential for code sharing between web, iOS, and Android platforms
Preparing for the Migration
Before starting the migration process, it's essential to properly assess your current Xamarin app and understand the differences between Xamarin and React Native for iOS and Android.
Assessing Your Current Xamarin App
Start by thoroughly analyzing your existing app:
- Document all features and functionalities for both iOS and Android versions
- Identify platform-specific implementations for each OS
- List all third-party dependencies and libraries used
- Review the app's architecture and data flow
- Analyze the app's performance metrics on both platforms
Understanding the Differences Between Xamarin and React Native
While both frameworks aim to provide cross-platform development capabilities, they have some fundamental differences:
- Language: Xamarin uses C# and .NET, while React Native uses JavaScript (or TypeScript) and React.
- UI Rendering: Xamarin.Forms uses native UI components, whereas React Native uses a JavaScript bridge to render native components on both iOS and Android.
- Development Paradigm: Xamarin follows an object-oriented approach, while React Native is based on functional programming and component-based architecture.
- Styling: Xamarin uses XAML for UI design, while React Native uses a subset of CSS with Flexbox for layout on both platforms.
Setting Up the React Native Development Environment
To begin your migration journey, set up your React Native development environment:
- Install Node.js and npm (Node Package Manager)
- Install the React Native CLI
- Set up Xcode for iOS development
- Set up Android Studio and Android SDK for Android development
- Install a code editor (e.g., Visual Studio Code) with relevant extensions for React Native development
Planning the Migration Strategy
A well-thought-out migration strategy is crucial for a successful transition on both iOS and Android.
Deciding Between Gradual Migration or a Complete Rewrite
You have two main options:
- Gradual Migration: This approach involves slowly replacing parts of your Xamarin app with React Native components. It's less risky but can be more complex and time-consuming.
- Complete Rewrite: This involves rebuilding your entire app in React Native from scratch. It's more straightforward but requires more upfront time and resources.
The choice depends on app complexity, team size, timeline, and budget. Consider how this decision will affect both your iOS and Android versions.
Identifying Core Functionalities and Components to Migrate
Prioritize your app's features based on:
- Importance to user experience on both platforms
- Complexity of implementation
- Dependency on native iOS or Android features
Create a list of components and screens to be migrated, starting with the most critical ones that are common to both platforms.
Creating a Migration Roadmap and Timeline
Develop a detailed plan that includes:
- Milestones for each major feature or component on both iOS and Android
- Allocation of resources and team members
- Testing phases and quality assurance checkpoints for each platform
- Contingency plans for potential setbacks
Migrating the UI
Transitioning your user interface from Xamarin to React Native requires careful mapping of controls and rethinking your layout approach for iOS and Android.
Using React Native UI Libraries
To streamline the UI migration process and ensure a consistent look across platforms, consider using React Native UI libraries. Two popular options are:
- gluestack-ui: A universal UI library that offers a set of accessible, customizable components. It's designed to work seamlessly across iOS and Android, providing a consistent look and feel.
Key features: - Highly customizable components
- Accessibility-focused design
- Theme support for easy styling
- TypeScript support
Example usage:
- Tamagui: A universal UI kit that focuses on performance and developer experience. It offers various customizable components and utilities for iOS and Android.
Key features: - Optimized for performance on both platforms
- Support for React Native and web
- Powerful theming system
- Built-in animations
Example usage:
Using these libraries can help you:
- Accelerate the UI migration process for both platforms
- Ensure consistency across your app on iOS and Android
- Reduce the amount of custom CSS you need to write
- Take advantage of pre-built, optimized components
When choosing a UI library, consider factors like component availability, customization options, platform performance, and community support.
Mapping Xamarin.Forms Controls to React Native Components
Create a mapping of Xamarin. Forms control to their React Native equivalents. For example:
Label
→<Text>
Entry
→<TextInput>
Button
→<Button>
or<TouchableOpacity>
ListView
→<FlatList>
or<ScrollView>
Recreating Layouts Using React Native's Flexbox
React Native uses flexbox for layout, which works consistently across iOS and Android. Key points to remember:
- Use
flex
properties to control component sizing and positioning - Implement responsive designs using percentage-based dimensions and flexbox
- Utilize
StyleSheet
to create reusable styles
Handling Platform-Specific Designs and Responsiveness
To ensure your app looks great on all iOS and Android devices:
- Use the
Dimensions
API to get screen size and adjust layouts accordingly - Implement responsive typography using
PixelRatio
- Utilize the
SafeAreaView
component for iOS notches and Android cutouts - Use
Platform.OS
to apply platform-specific styles when necessary
Most of this will be handled by the UI library though.
Porting Business Logic
Transitioning your app's core functionality requires careful translation of your C# code to JavaScript or TypeScript.
Converting C# Code to JavaScript/TypeScript
When converting your business logic:
- Rewrite C# classes as JavaScript classes or functional components
- Replace LINQ queries with JavaScript array methods (map, filter, reduce)
- Adapt to JavaScript's asynchronous nature using Promises or async/await
- Consider using TypeScript for stronger type checking and better IDE support
Adapting to React Native's Component Lifecycle
React Native components have a different lifecycle compared to Xamarin:
- Replace
OnAppearing
andOnDisappearing
withuseEffect
for functional components (orcomponentDidMount
andcomponentWillUnmount
for class components). - Implement state updates using React Hooks
useState
,useEffect
(orsetState
for class components).
Implementing State Management
For complex apps, consider using a state management solution that works well for both iOS and Android:
- Redux: Offers a centralized store and predictable state updates
- MobX: Provides a more flexible, observable-based state management
- React Context: Built-in solution for simpler state management needs
Handling Platform-Specific Features
Many platform-specific features in your Xamarin app will need special attention during migration.
Identifying Platform-Specific Functionalities
Review your Xamarin code for iOS and Android-specific implementations, such as:
- Custom renderers
- DependencyService implementations
- Platform-specific API calls
Using React Native Modules and Native Modules
React Native provides several ways to access native functionality on both platforms:
- Use built-in React Native modules for common features
- Leverage community-built native modules for additional functionality
- Create custom native modules for specific iOS or Android features not available in React Native
Implementing Platform-Specific Capabilities
Adapt key platform features to React Native:
- Push Notifications: Use libraries like
react-native-push-notification
- Deep Linking: Implement using React Navigation's deep linking support
- Biometric Authentication: Utilize
react-native-biometrics
for fingerprint and face recognition on both platforms
- Use
Data Management and API Integration
Efficient data handling is crucial for your app's performance and user experience on both iOS and Android.
Migrating Local Data Storage Solutions
Transition your local storage approach:
- Replace SQLite with AsyncStorage or realm for simple data storage
- Use libraries like WatermelonDB for more complex local databases
- Implement caching strategies to optimize data access on both platforms
Adapting API Calls and Networking Code
Update your networking layer:
- Use fetch API or Axios for making HTTP requests
- Implement proper error handling and request cancellation
- Consider using React Query or SWR for efficient data fetching and caching
Implementing Caching and Offline Capabilities
Enhance your app's offline experience for both iOS and Android:
- Implement offline-first architecture using libraries like Redux Offline
- Use background sync to update data when the app comes online
- Provide clear feedback to users about the app's online/offline status
Testing and Quality Assurance
Thorough testing is essential to ensure your migrated app functions correctly and provides a smooth user experience on both platforms.
Setting Up a Testing Environment for React Native
Establish a robust testing setup:
- Use Jest for unit and integration testing
- Implement Detox for iOS and Espresso for Android end-to-end testing
- Set up continuous integration (CI) using tools like Jenkins or GitHub Actions
Writing Unit Tests and Integration Tests
Focus on comprehensive test coverage:
- Write unit tests for individual components and functions
- Create integration tests for complex interactions between components
- Use snapshot testing to catch unintended UI changes on both platforms
Performing Manual Testing and UI/UX Reviews
Complement automated tests with thorough manual testing:
- Conduct usability testing to ensure a smooth user experience on both iOS and Android
- Test on various devices and OS versions for both platforms
- Perform accessibility testing to ensure your app is usable by all
Performance Optimization
Optimizing your React Native app's performance is crucial for user satisfaction on both iOS and Android.
Identifying and Resolving Performance Bottlenecks
Use React Native's built-in performance tools and third-party solutions to identify issues:
- Utilize the Performance Monitor to track JS frame rate and UI updates
- Use libraries like
react-native-performance
for detailed performance metrics - Profile your app to identify slow functions or excessive re-renders on both platforms
Optimizing React Native App Size and Load Time
Reduce your app's footprint and improve startup time on both iOS and Android:
- Use app size optimization techniques like removing unused resources
- Implement code splitting to load only necessary components
- Optimize images and assets using tools like ImageOptim
Implementing Lazy Loading and Code Splitting Techniques
Improve app performance by loading content as needed:
- Use dynamic imports to load non-critical components on demand
- Implement infinite scrolling for long lists instead of loading all items at once
- Utilize React Suspense and lazy loading for code splitting on both platforms
Conclusion
Converting a Xamarin app to React Native for iOS and Android is a significant undertaking, but it offers numerous benefits. This comprehensive guide outlines the key steps and considerations for successfully migrating across both platforms. Remember that the process requires patience, careful planning, and a commitment to continuous learning and improvement.
The transition to React Native opens up new possibilities for your app, including improved performance, a larger developer community, and the potential for easier cross-platform development. As you embark on this journey, stay focused on delivering value to your users and maintaining the quality and functionality they've come to expect from your app on both iOS and Android.
With dedication and the right approach, your newly migrated React Native app will be well-positioned for future growth and success in the ever-evolving mobile app landscape across both major mobile platforms.
Dive deep into our research and insights. In our articles and blogs, we explore topics on design, how it relates to development, and impact of various trends to businesses.