Mastering the useThrottle Hook in React
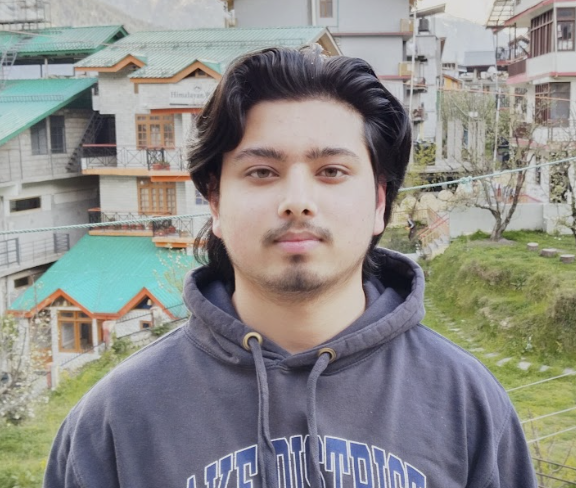
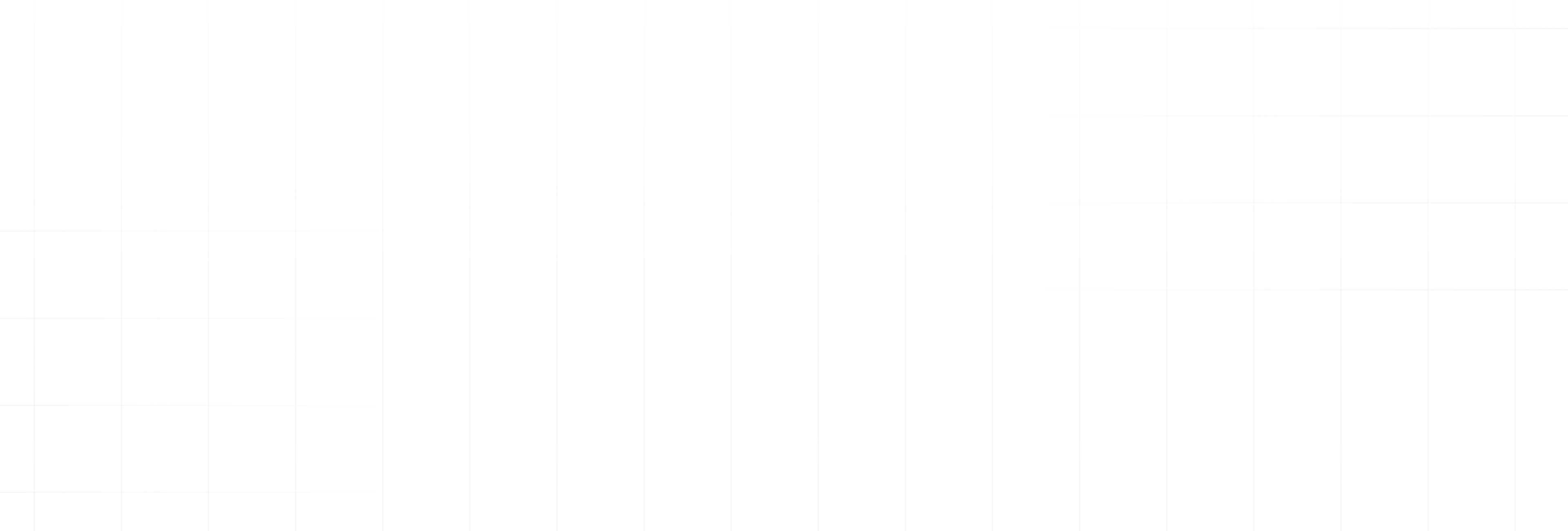
In the fast-paced world of web applications, user interactions can trigger a flurry of events. While responsiveness is crucial, excessive function calls can lead to performance bottlenecks. Enter the useThrottle
hook, a powerful tool in React's arsenal for managing execution frequency.
Understanding Throttling
Throttling ensures that a function executes at most once within a specified time interval, regardless of how many times it is called. It is similar to a leaky faucet that only releases water intermittently, preventing a deluge.
Why Use Throttling?
- Performance Optimization: Throttling prevents repetitive, expensive operations, such as API calls or complex calculations, from overwhelming the browser. This smooths out user experience and enhances application responsiveness.
- Event Debouncing: It is ideal for scenarios where rapid user input (for example, continuous scrolling, resize events) might trigger unnecessary updates. Throttling ensures they are handled efficiently, minimizing redundant computations.
How Does useThrottle Work?
Let us dissect the provided code:
Import and Setup:
JavaScript
import { useRef } from 'react';
We import useRef
from React to create a reference that persists across re-renders, ideal for tracking the last execution time.
useThrottle
Hook Function:
Parameters:
cb
: The callback function to be throttled. It takes no arguments and returns nothing (void).limit
: The time interval (in milliseconds) between allowed executions.
lastRun
Reference:
We create a useRef
hook named lastRun
to store the timestamp (milliseconds since epoch) of the most recent execution. This ensures the function is called at most once within the specified limit
.
Returned Function:
This is the throttled function you will use in your React components. It checks the time difference between the current time (Date.now()
) and lastRun.current
. If the difference is greater than or equal to limit
, it executes the cb
function and updates lastRun.current
with the current timestamp.
Using useThrottle in React Components
Here is an example of how to use the useThrottle
hook in a React component to throttle a scroll event handler:
In this example, the throttledScroll
function is created using useThrottle
and passed to the scroll event listener. This ensures that the handleScroll
function is only called at most every 2000 milliseconds, even if the user scrolls rapidly.
Conclusion
The useThrottle
hook is a valuable tool for enhancing performance and managing function execution frequency in React applications. By understanding its purpose and implementation, you can make informed decisions about when to use it to improve the responsiveness and efficiency of your web applications.