Table of Contents
How Meta Platforms Handle Image Resize & Compression: Optimizing Image Resize and Compression with JavaScript Techniques
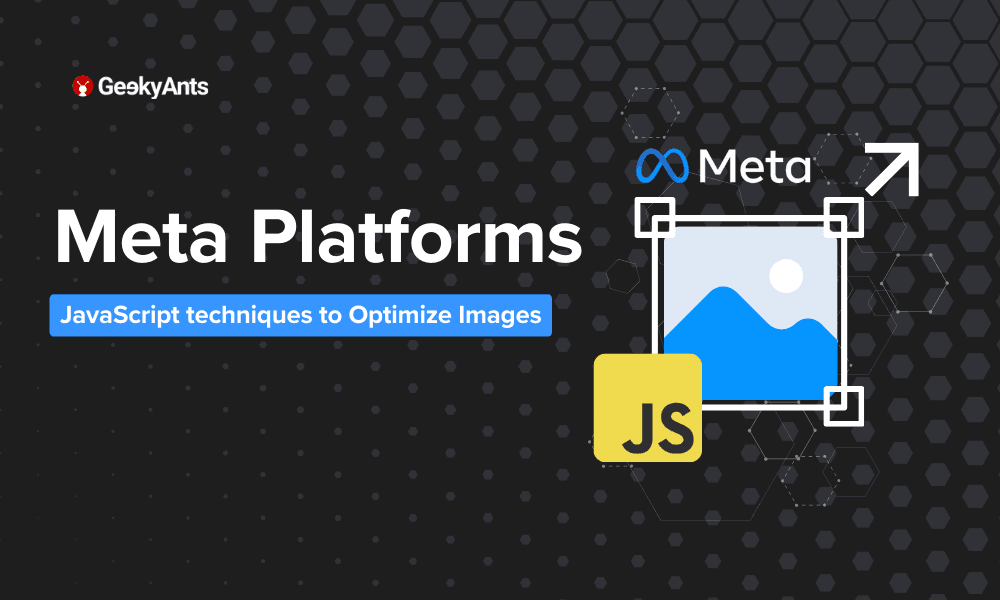
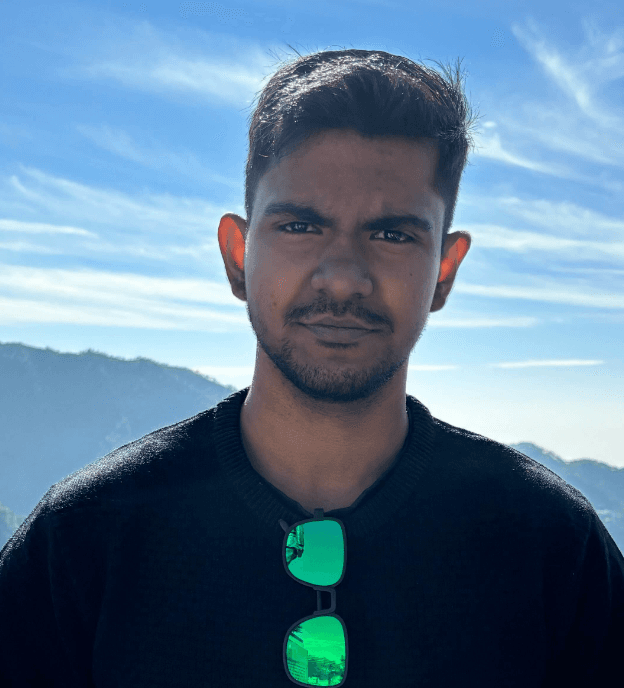
Book a call
We often notice that on every platform where image uploads are needed, the images are compressed before being uploaded. Popular social media platforms like Instagram, Facebook, and WhatsApp compress images before uploading them. But why do they do this, and how can we achieve the same?
The primary reason for compressing images is to reduce the file size, which helps in faster uploads and downloads. Smaller file sizes also save server storage space and reduce bandwidth usage, making the platform more efficient and cost-effective. Additionally, compressed images load faster for users, improving the overall user experience.
Here, you will learn different techniques for resizing and compressing images before uploading. Each technique has unique pros and cons, and you will discover which technique to use in your application based on your needs.
Prerequisites
In the frontend, it will look something like this:
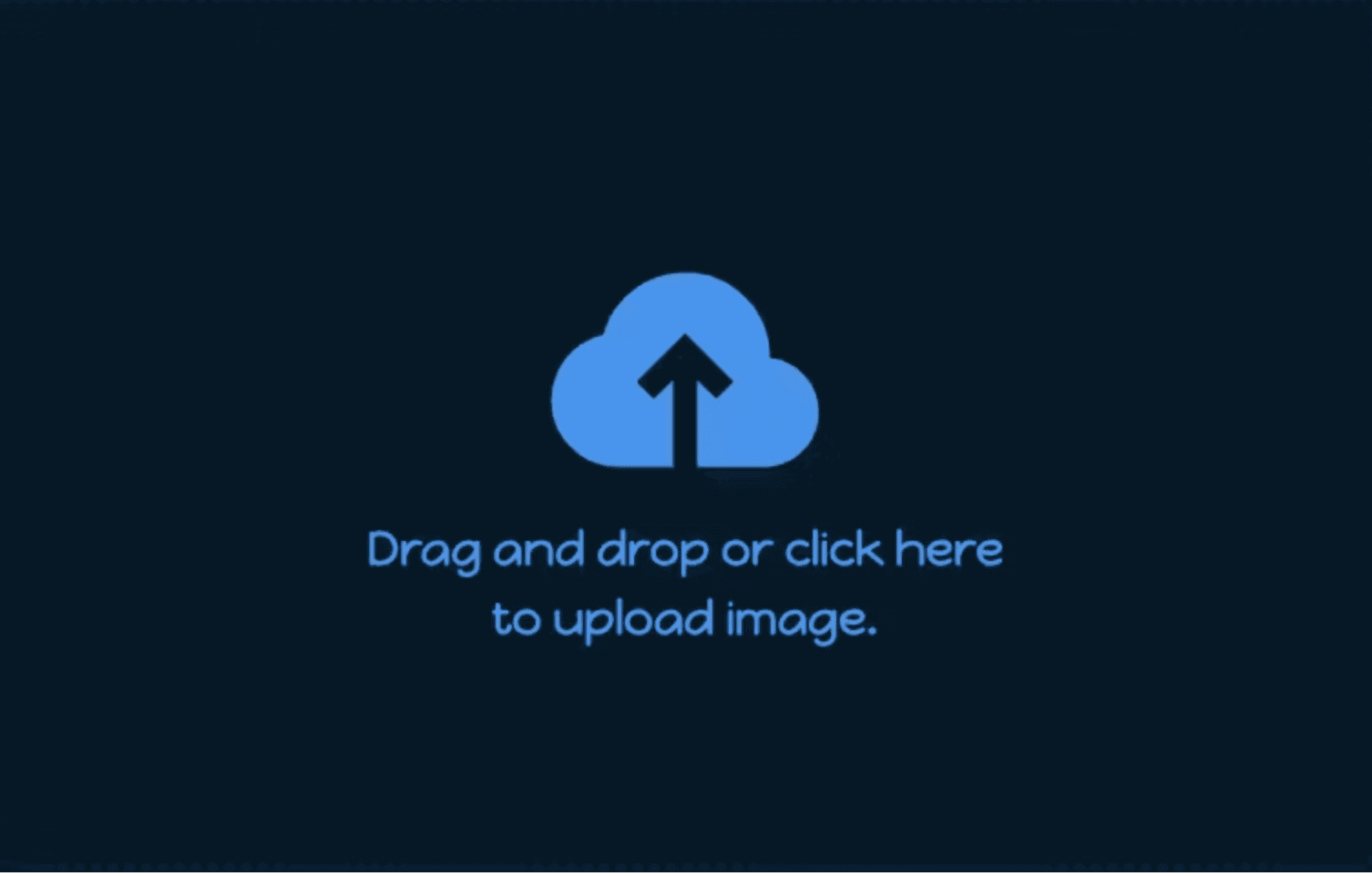
We call this uploadFile function from the UI whenever there is any change in the input file.
Techniques
Now you might be wondering what the techniques are. So, let's go through it.
- Using canvas API .toDataURL() method.
- Using canvas API .toBlob() method.
- Using third party external library Pica.
- Using third library compress.js.
Let's understand it one by one:
NOTES: We are considering resizing and compression of image. (In every implementation we have maxWidth=1200
and maxHeight=1200
, and imageQuality=0.8
)
canvas API .toDataURL():
Introduction
The toDataURL(type, encoderOptions)
method of the Canvas API allows us to convert the image in the canvas to a data URL. The encoderOptions
parameter can specify the image quality, with a value between 0 and 1, for formats that support lossy compression.
Implementation
Pros
Provides a blob object, which is useful for file uploads and further processing.
Control over image quality.
Cons
Slightly more complex than
toDataURL
.Similar performance limitations as
toDataURL
for large images.
Pica:
Introduction
Pica is a high-quality image resizing library for Canvas that also supports image compression. You can use the resize method to resize
images and either pica.toBlob
or canvas.toBlob
to compress the image.
Implementation
Pros
- Fast and efficient image resizing.
- High-quality resizing algorithms.
- Easy to integrate and use.
Cons
- Requires adding an external library.
- The bundle size is slightly larger due to the library.
compress.js:
Introduction
compress.js is a library that provides high-level abstraction for resizing and compressing images. It balances ease of use and functionality.
Implementation
Pros
- High level of abstraction and ease of use.
- Includes both resizing and compression.
- Good balance between image quality and file size.
Cons
- Adding an external library increases the project size.
- Might not support all image formats natively.
These are some ways to handle image compression and resizing images. Let me know in the comments if I forgot to add any methods for resize and compression.
Conclusion
Choosing the right method for image compression and resizing in JavaScript depends on your specific requirements and constraints; for simplicity and minimal setup: Use canvas.toDataURL
if you only need a base64 string, or canvas.toBlob
if you need a Blob object.
For better performance and quality: Use Pica if you are dealing with large images and need high-quality resizing or Compress.js if you want an easy-to-use library that handles both resizing and compression effectively
- Canvas API to
DataURL()
: - Simple and straightforward.
- Provides control over image quality.
- Suitable for moderate image sizes but can be slow for larger images.
- Canvas API to
Blob()
: - Produces a
Blob
object, ideal for file uploads. - Offers control over image quality.
- Slightly more complex than to
DataURL
but has similar performance limitations for large images. - Pica:
- High-quality and efficient image resizing.
- Easy to use with powerful algorithms.
- Requires an external library, which increases bundle size.
- Compress.js:
- High level of abstraction with ease of use.
- Combines both resizing and compression effectively.
- Requires an external library, which may not support all image formats natively.
Key Takeaways:
Simplicity vs. Control: The
canvas.toDataURL()
andcanvas.toBlob()
methods are easy to implement and provide control over the image quality. However, they might not be the most efficient for large images.Performance: For high-performance needs, especially with large images, using a specialized library like Pica can provide better results with high-quality resizing and compression.
Ease of Use:
Compress.js
offers a simple and comprehensive solution for both resizing and compressing images but comes with the trade-off of adding an external library.Blob Handling: Methods that produce
Blob
objects (toBlob
andpica
) are particularly useful when dealing with file uploads and further processing on the server side.Library Overhead: While external libraries provide advanced functionalities and ease of use, they increase the overall bundle size of your project, which might be a consideration for performance-sensitive applications.
By understanding these methods' strengths and weaknesses, you can make an informed decision on the best approach for your image processing needs in JavaScript.
Dive deep into our research and insights. In our articles and blogs, we explore topics on design, how it relates to development, and impact of various trends to businesses.