Creating Nested Reorderable Lists with Custom Scrolling in Flutter
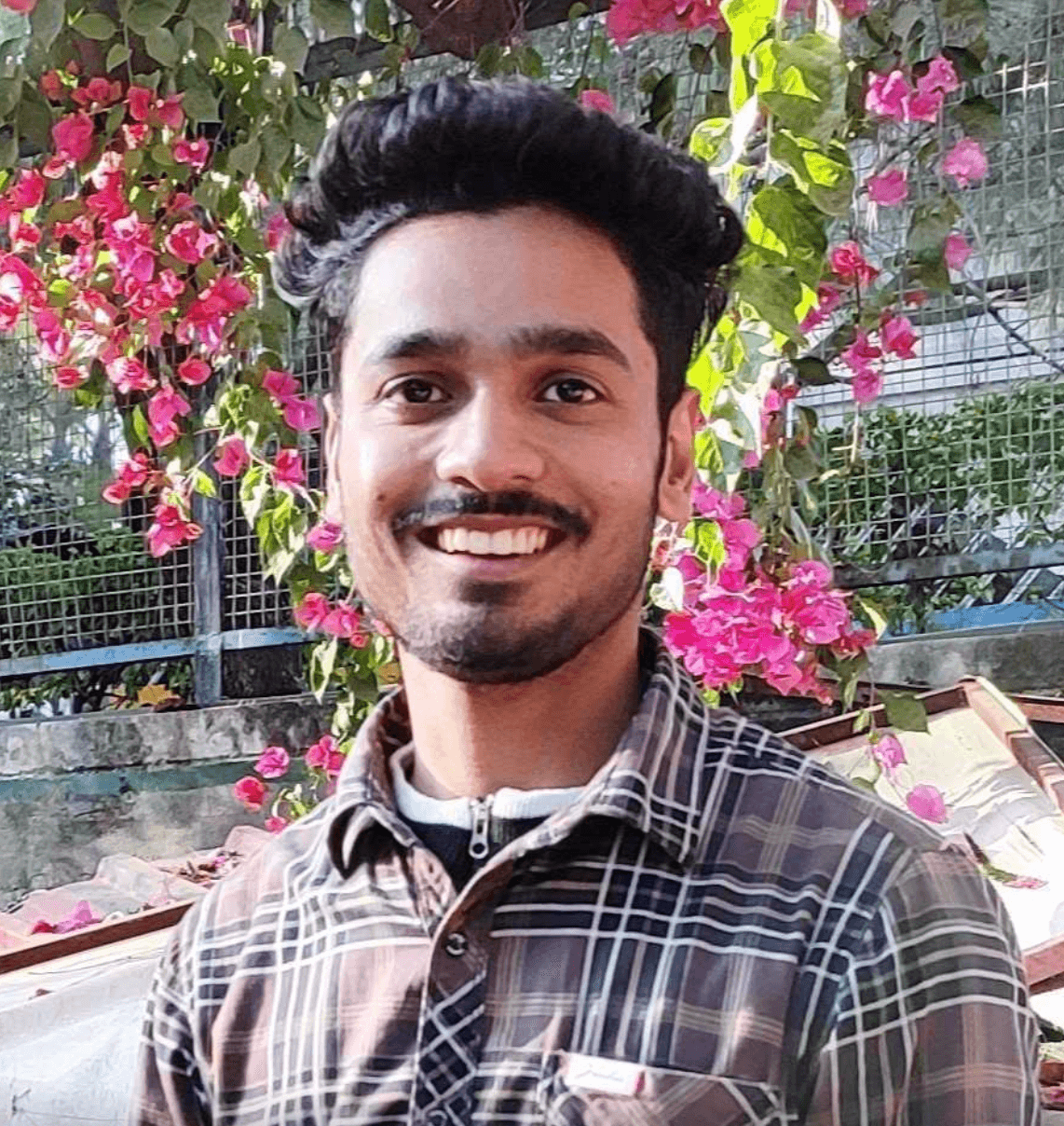
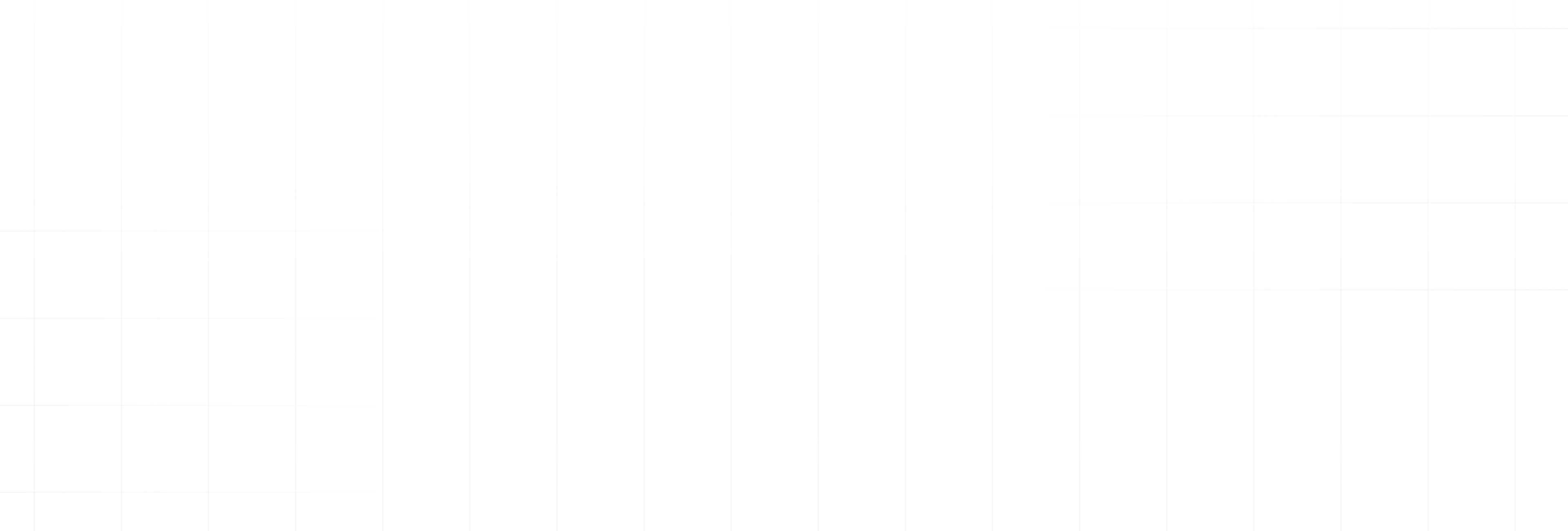
In this article, we will explore the process of creating nested reorderable lists in Flutter, with a focus on implementing custom scrolling behaviour. Reorderable lists are a powerful UI component that allow users to rearrange items within a list by dragging and dropping. While Flutter provides a built-in ReorderableListView
widget that handles most of the functionality out of the box, certain use cases such as nested reorderable lists introduce challenges, particularly with scrolling behavior.
We will cover:
- The basics of
ReorderableListView
- Creating a nested reorderable list
- Implementing custom scrolling to handle edge cases
- Full example with code and explanation
Understanding ReorderableListView
The ReorderableListView
widget in Flutter makes it easy to implement drag-and-drop reordering within a list. Here’s a simple example to demonstrate the basic usage:
Challenges with Nested Reorderable Lists
The Problem
When implementing a nested ReorderableListView
inside a SingleChildScrollView
, the scrolling behaviour can be problematic. Specifically, the list does not scroll automatically when you drag an item near the edges of the screen, which can lead to a poor user experience.
When using nested ReorderableListView
widgets, you might encounter issues, particularly with scrolling behavior. In a single ReorderableListView
, scrolling is handled automatically as you drag an item near the top or bottom of the list. However, when nesting reorderable lists inside a scrollable parent, you might find that scrolling doesn’t behave as expected, especially when dragging items between different lists.
Implementing Nested Reorderable Lists
Let's build a more complex example with nested reorderable lists, and address the custom scrolling behavior to handle edge cases.
Step 1: Creating the Main Structure
First, we will set up the main structure with a scrollable parent that contains two reorderable lists.
Step 2: Handling Custom Scrolling
To handle custom scrolling when dragging items near the top or bottom, we need to implement logic that smooths out the scrolling behavior. The goal is to ensure the parent scroll view scrolls appropriately when dragging items in nested reorderable lists.
The Solution
To solve this issue, we need to:
- Use a
ScrollController
for the main scroll view. - Handle continuous scrolling by monitoring the pointer's position relative to the screen.
- Use a
Timer
to ensure smooth and continuous scrolling when the user drags an item near the edges.
Complete Implementation
Below is the complete implementation of a nested reorderable list with custom scroll behavior in Flutter:
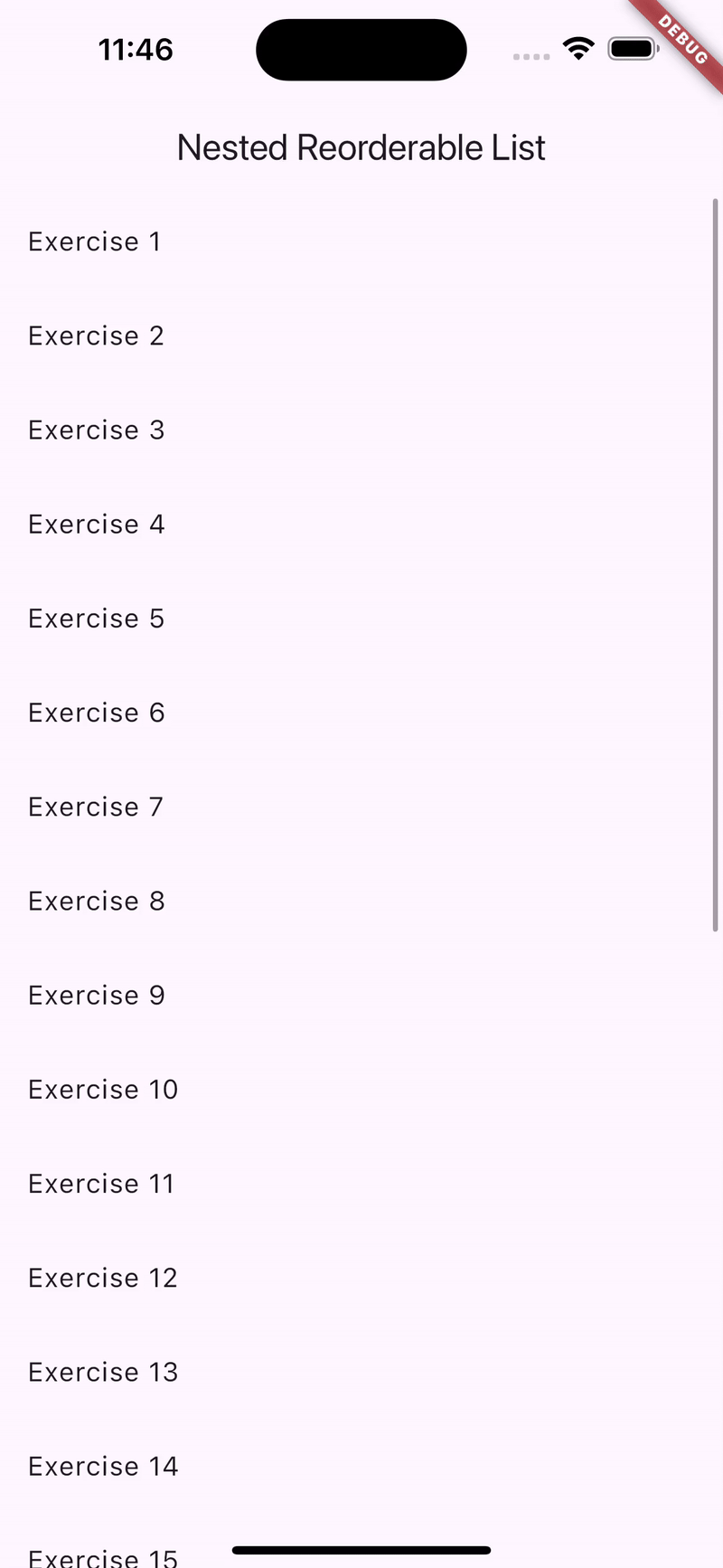
Explanation of Custom Scrolling Logic
- Listener Widget:
- The
Listener
widget is used to capture pointer move events. - Calls
_startAutoScroll
with thedy
position of the pointer to determine if scrolling should be started.
- The
- _startAutoScroll Method:
- Checks if the pointer is near the top or bottom of the screen.
- Calls
_startScrolling
to initiate scrolling in the respective direction if the pointer is within the scroll threshold.
- _startScrolling and _stopScrolling Methods:
- These methods handle the actual scrolling using a periodic timer to ensure smooth and continuous scrolling.
jumpTo
is used to update the scroll position instantly, and the timer ensures regular updates for smooth scrolling.
- SingleChildScrollView with ScrollController:
- Ensures the entire content is scrollable with a single
ScrollController
. - This controller is used to manage scrolling when dragging items near the edges.
- Ensures the entire content is scrollable with a single
By using these techniques, you can ensure a smooth and intuitive scrolling experience when dragging items in nested reorderable lists.
Conclusion
Creating nested reorderable lists in Flutter with custom scrolling behavior can be challenging but rewarding. By understanding the basics of ReorderableListView
and implementing custom logic for handling edge cases, you can build a robust and user-friendly interface.
The full example provided in this article should give you a solid foundation to start with nested reorderable lists in your Flutter applications. Feel free to adjust the scrolling parameters and behavior to fit your specific needs. Happy coding!
Book a Discovery Call.
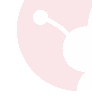