React Hooks vs Class Component
Author
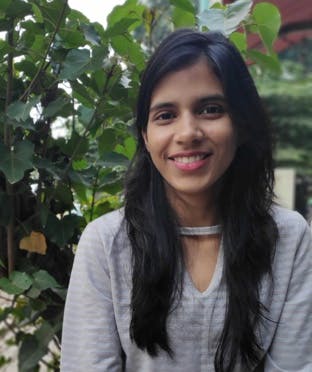
Date
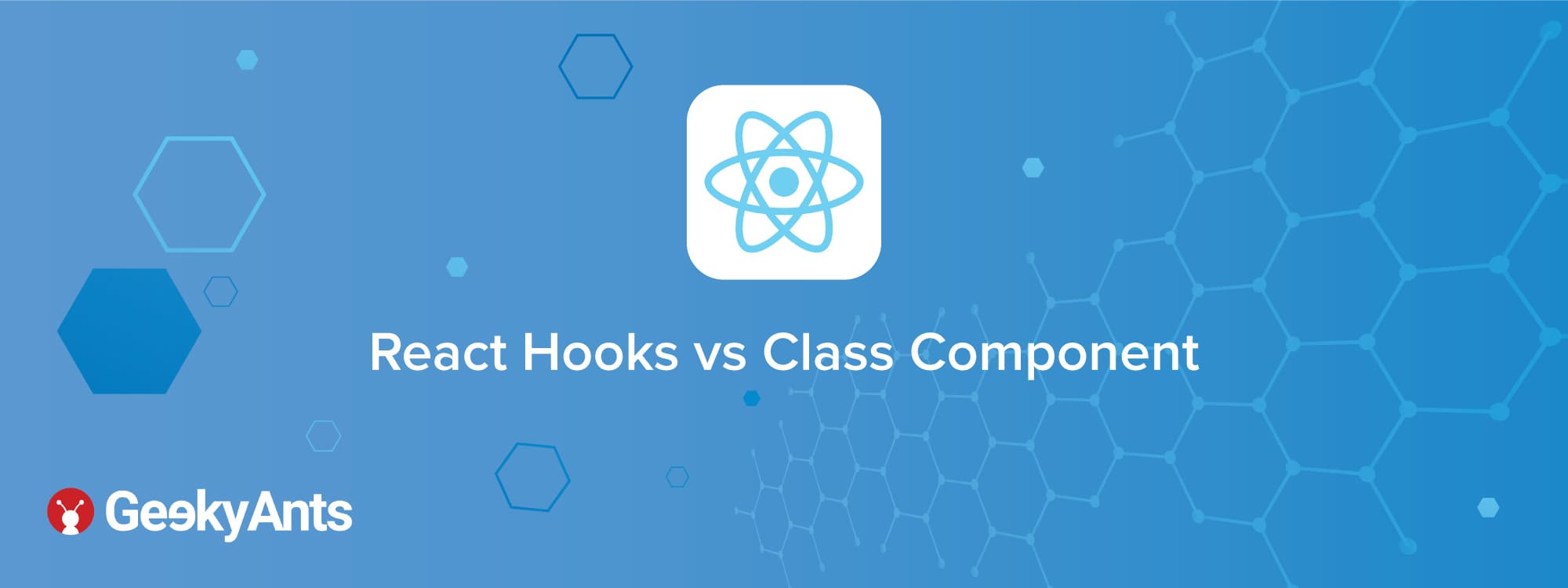
Book a call
Before the React 16.8 Hooks update in 2018, state only belonged to Class components and were therefore Stateful, while functional components were plain Javascript functions and couldn’t use state. Since functional components were just for presentation purposes i.e. to present something to the DOM, they were also called Presentational or Dumb Components.
...and then the hooks update was released. Looks like someone wasn’t supposed to stay dumb forever :-)
In this article, I will talk about my experiences with them and discuss the key differences between these two that I came across.
- Import & Structure: Class components extend from
React.Component
(inheritance) and give the component access toReact.Component
's functions. This class component should have an only required render() method further to return a React element and call it instead of implementing its own render logic.
- Constructor: You will also see a constructor being used but it’s not mandatory or required to have it in your class as you can get things done without it too. Phew!
- State: React components have a built-in state object where you can store property values of the component. These state objects and their properties can be accessed by this keyword throughout the component. When you want to update the state value, you can do it using setState. But in function, the value of this depends upon how that function is invoked. Before discussing the previous statement, let’s discuss event and event handlers.
- Event and Event handlers: An event is an action that occurs as a result of the user or any other source, such as a mouse click etc. An event handler is a routine that deals with the event.
Suppose you want to handle some event on the click of a button. Your event handler will be something like this:
... and if this is how you call the event handler:
You will get this
value as undefined
, this is because the handleClick()
has lost its component object or this
value, i.e. inside the method it cannot identify this
reference of the component. Now, this is what I meant when I said it depends on how the function is invoked. Therefore, in order to get the value of this
inside the function we need to either:
- Bind the function
2. Or use an arrow function
a) As a callback:
...or
b) In the event handler
So, that’s how things work in class. Now let’s see how it works with hooks.
In functional components, it's super easy to work because the curse of using this everywhere you use your local variable or call functions doesn't exist. Therefore, no significant use of arrow functions as well. With less code compared to class, it's already a win.
Now how does this ‘dumb’ function gets state? Simple. Through theuseState
hook. Everything else is exactly the same as that of the class.
What are lifecycle methods?
React class has lifecycle methods which are a series of events that happen from the time component is mounted to till it is unmounted.
For the component these series of events can be divided into 3 parts :
- Mount
- Update
- Unmount
Now that must make you wonder if render()
is a lifecycle method or not. Of course it is. It occurs between mounting of the component and its update.
Corresponding to these three events, we have three common lifecycle methods.
ComponentDidMount
ComponentDidUpdate
ComponentWillUnmount
Coming to functions, we have one hook that does all these lifecycle functions- useEffect()
.
Interesting isn’t it?
But how does it have all 3 lifecycle functions in one?
Let’s find out.
The useEffect()
takes two arguments: a callback function and an array of dependencies. The second argument is more interesting. When an empty array is given, it means you want to run an effect and clean it up only once, thereby achieving mount
and unmount,
but on passing a state variable in the array calls the useEffect()
each time the state variable value changes, thus causing an update.
The demo for the same using functional components is given here, if you're interested to see how it works.
Hope this article helped you, thanks for reading.
Happy Coding!
Dive deep into our research and insights. In our articles and blogs, we explore topics on design, how it relates to development, and impact of various trends to businesses.