Performing Functional Testing Using Jest For QA
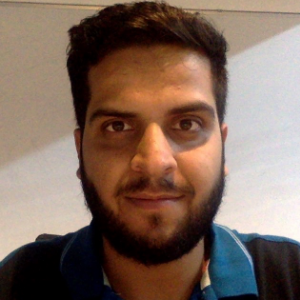
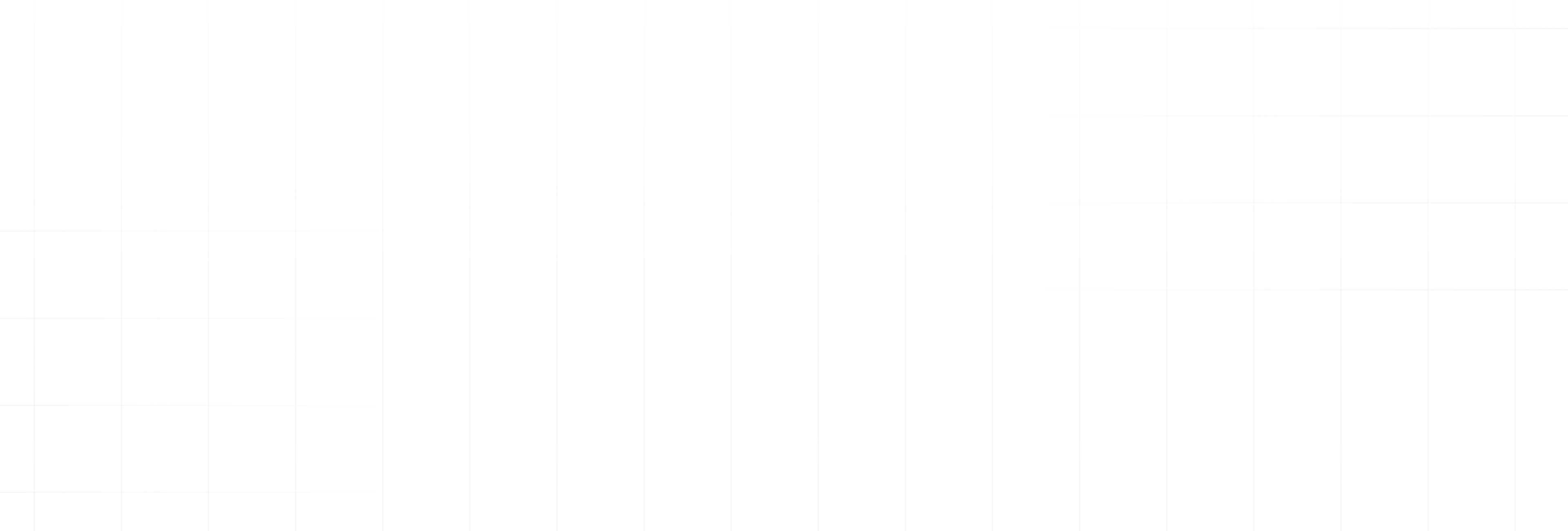
Functional testing is black-box testing that ensures software behaves as expected, validating that each application function operates in conformance with the requirement specification. Jest, a robust JavaScript testing framework developed by Facebook, is particularly effective for testing JavaScript applications, including those built with React. This comprehensive guide will walk you through setting up and performing functional testing using Jest.
Why Jest for Functional Testing?
Jest is favored for functional testing due to its numerous advantages:
- Ease of Use: Minimal configuration is required, making setting up and testing simple.
- Rich Assertion Library: Comes with built-in assertions, eliminating the need for additional libraries.
- Snapshot Testing: Allows easy UI testing by comparing the current output with a stored snapshot.
- Coverage Reporting: Integrated coverage reporting helps to assess the thoroughness of tests.
- Parallel Execution: Tests run parallel, speeding up the overall test suite execution.
Prerequisites
Before starting, ensure you have the following:
- Node.js and npm: Installed and set up on your machine.
- Basic JavaScript Knowledge: Understanding of JavaScript and testing concepts.
- Initialized Project: Your JavaScript project should have a
package.json
file.
Setting Up Jest
Install Jest: Open your terminal and run:
Configure Jest: Add a test script in your package.json
file to enable running tests using npm:
Create a Configuration File (Optional): For more advanced configurations, create a jest.config.js
file:
Writing Functional Tests with Jest:-
Functional tests typically involve writing test cases for individual functions or components to ensure they meet the expected behavior.
- Creating a Test File: Jest automatically recognizes files with extensions
.test.js
or.spec.js
.
Create a file namedsum.test.js.
2. Writing a Simple Test:
3. Running the Test: Execute your tests by running:
Advanced Functional Testing Techniques :-
- Testing Asynchronous Code: Handling asynchronous code in Jest can be done using
async/await
or Promises.
2. Mocking Functions: Jest provides powerful tools for mocking functions to isolate and test units of code.
3. Snapshot Testing: Snapshots are particularly useful for testing UI components to ensure they render correctly.
4. Integration with React Testing Library: Combining Jest with React Testing Library enhances the testing capabilities for React components.
Best Practices for Functional Testing with Jest :-
- Isolate Tests: Ensure that tests are independent and do not rely on each other. Each test should set up and tear down its environment.
- Use Descriptive Test Names: Clear and descriptive test names help understand what is being tested and the expected outcome.
- Mock External Dependencies: Mock any external services or APIs to ensure tests are reliable and run quickly.
- Maintainable Test Code: Write clean and maintainable test code. Refactor tests when necessary to improve readability and maintainability.
- Test Coverage: Utilize Jest’s coverage reporting feature to ensure comprehensive testing. This helps identify untested parts of the code.
Example of Comprehensive Functional Testing :-
Let's consider a more comprehensive example involving a simple user authentication system.
Authentication Logic:
Authentication Test:
Conclusion
Jest is a powerful and versatile tool for functional testing in JavaScript applications. Its ease of use, integrated features, and ability to handle asynchronous code, mocks, and snapshots make it an excellent choice for ensuring software quality. By following the detailed steps and best practices outlined in this guide, you can leverage Jest to write effective and maintainable tests that enhance the reliability and robustness of your codebase.
Book a Discovery Call.
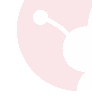