KBC Game in Python
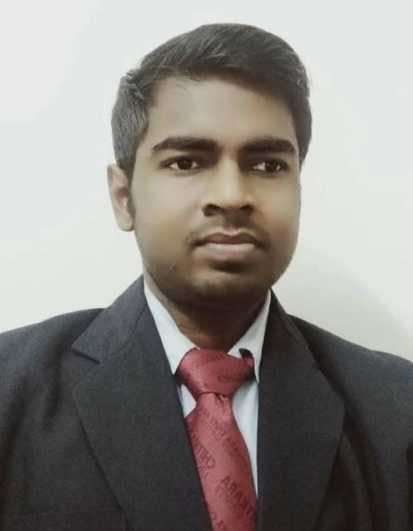
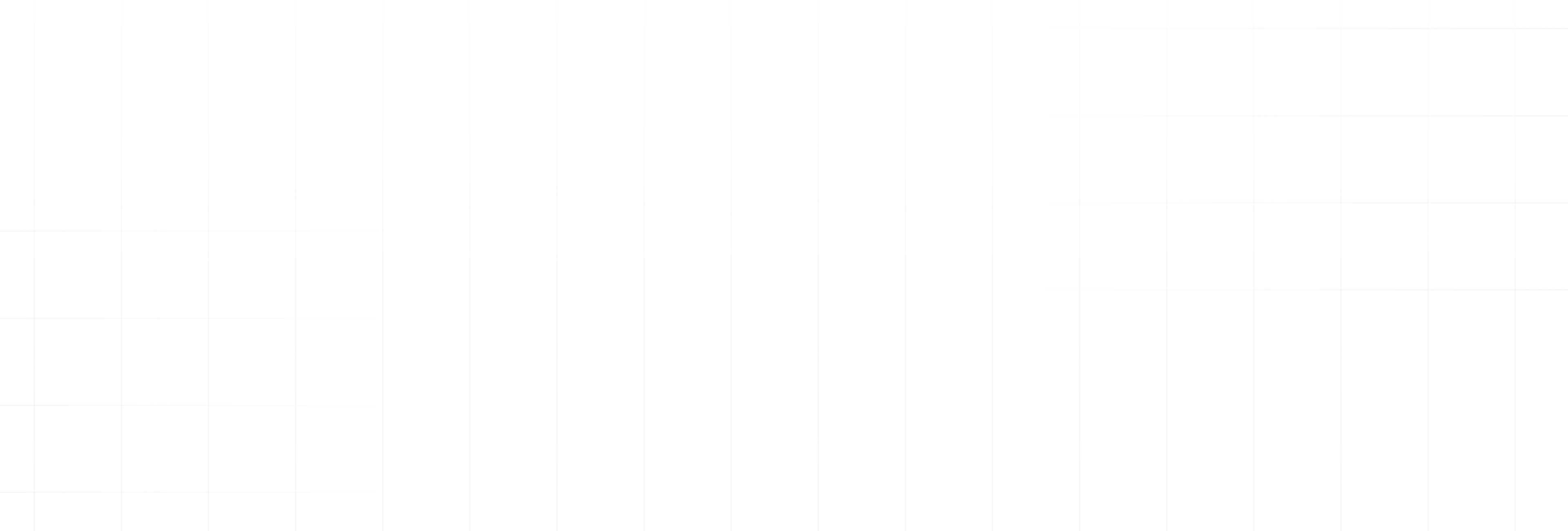
Hello Everyone! You all must have watched or heard about the very famous Television game show called KBC(Kaun Banega Crorepati), originally Who Wants to Be a Millionaire?. I took a chance to re-create that popular game show and refresh all those good old memories which we got sitting in front of the TV Screen.
Let’s Get Started
I have used Pygame module of Python 3 to create the game. Pygame is a cross-platform set of Python modules designed for writing video games. It includes computer graphics and sound libraries that are designed to be used with the Python programming language.
So, before proceeding further, make sure you have Python 3 and Pygame module installed in your system.
First, we need to define some color constant, amount list and question data set consisting of questions, options and its correct answers. You can create a custom question data set in a separate .json file or as a list of map objects in same file. I have used the latter option.
Setting Display Window
We can set our game screen display window to a fixed resolution and add title to our display window. Now we need to add some background properties to it to make it look more interactive. We can use an image as background and scale it to our desired resolution or just use color as background. I have used an image. Make sure you do all these things in the constructor of the class. Below is the code snippet for all that stuff:
Before Proceeding further let me tell you one thing, all the positional parameters I have used in below code is in relative to the game screen window size.So you have to adjust your positional parameters according to your window size.
Creating First Screen-Game Play Settings
First of all we have to notify users about how to play game. Pygame can detect keyboard events, so we have used onKeyPress
for event handling. We can display text on Screen using the render
method. We can also add font color and position to text.
Below is the code snippet for this part for better understanding:-
One thing we need to make sure of is to call pygame.display.update()
wherever required. It re-renders the screen with updated values just like setstate
in React.
Creating Game Play Screen
This is the main screen of the game where all fun stuff happens. It keeps track of the current status of the game and keeps us engaged with some interesting challenging questions.
This screen has a common component 'Rounded Rectangular Box' which is used as a basic building block for UI of this screen. You can find code for this component here
Now coming to the main UI part of this screen, first of all we have to add Price Tile which is nothing but a list of rounded rectangular boxes which contain the respective amount prize according to its level. The current level is denoted by red background color. Below is the code snippet for that:-
Now we have to just add a Question Box and an Option Box. In Question Box, we have to just render a large rectangular rounded box with the question as text on that surface. For Options, we have to render four small rectangular rounded boxes with their corresponding options as text. To make it more interactive, I have added a change in background color whenever user selects any option.Below is the code snippet for this part:-
Now we have to just merge all sub part of this screen into one which will act as the starting point of this screen. Here is the code snippet for this one:-
Creating Result Screen
This Screen is similar to the first screen. We have just replaced gameplay settings description text with final result description text. You can refer to the github repo for its code.
That’s all folks! This game is ready to play now. I hope you had a good time reading this. You can find the GitHub repo for the game here. You can refer to it for better understanding in case I missed out on something important. You can also add various new features to it also.
I am Vikram Kumar working as a Software Engineer Intern at GeekyAnts. Do reach out to me if you require assistance or anything else at all. I will be happy to help you.
Thank you. Bye Bye!