Full Stack (MERN) + GraphQL Boilerplate / Starter Part-II
Author
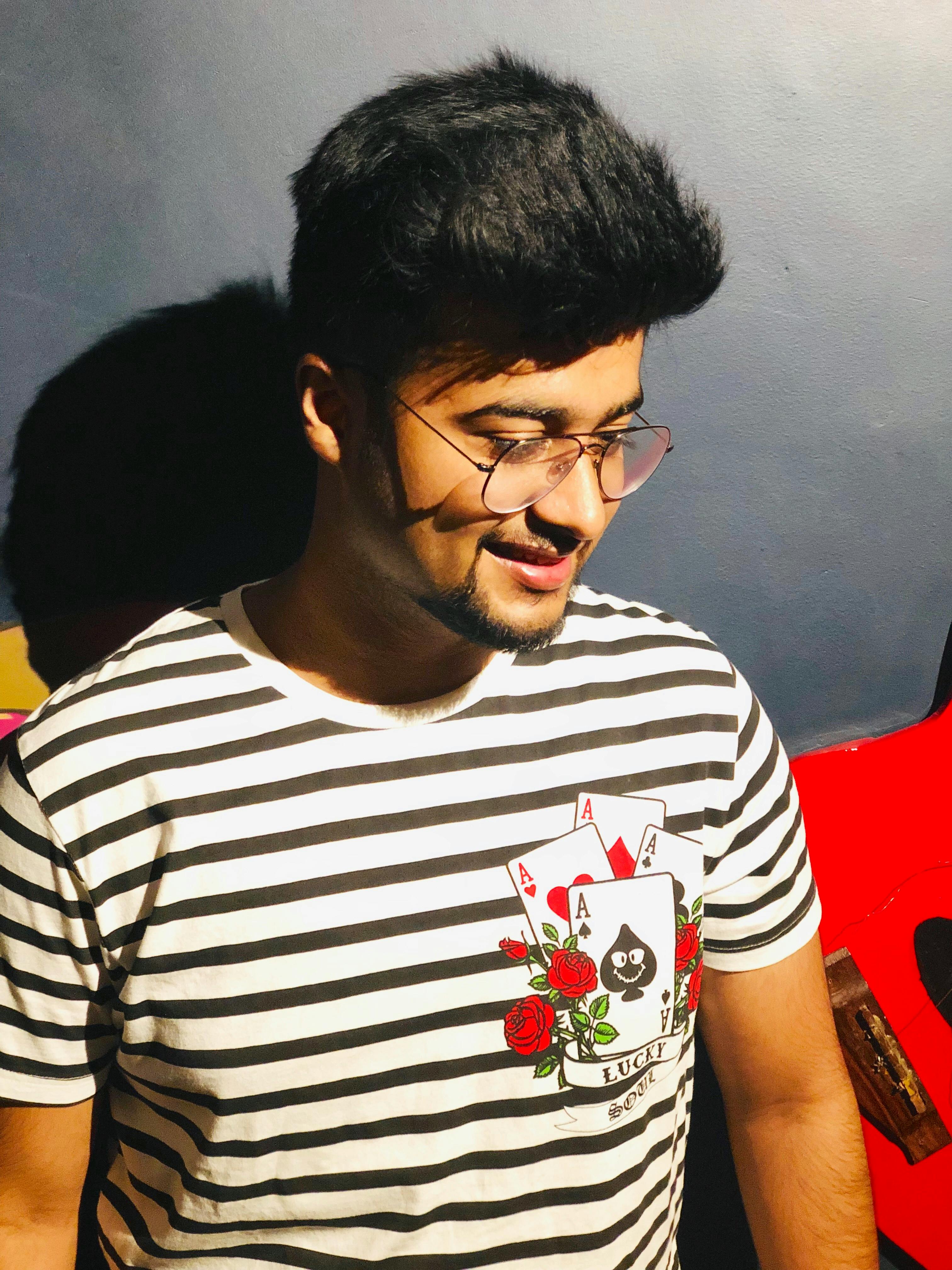
Date
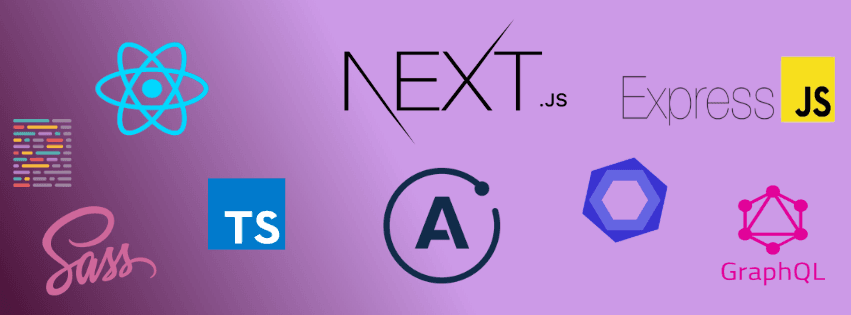
Book a call
This is the second article of the Full Stack (MERN) + GraphQL Boilerplate / Starter series:
Note: Before proceeding with this article, you need to set up the server for the boilerplate first, which is mentioned in the first part of the series. Follow the link above to read it.
Repository Link: - https://github.com/garganurag893/Next.js_GraphQL_Express_Apollo_Boilerplate
Next.js
Building a server-side rendered React website is hard. Next.js commits to solving this problem beautifully.
It comes with some handy features:
So, Let’s start by creating a new app :
Folder structure
Make the following folder structure to house everything:
Adding Dependencies:
Now we will add all GraphQL dependencies required for next.js :
Adding TypeScript:
TypeScript is a typed superset of JavaScript that compiles to plain JavaScript.
The presence of a tsconfig.json
file in a directory indicates that the directory is the root of a TypeScript project. The tsconfig.json
file specifies the root files and the compiler options required to compile the project. A project is compiled in one of the following ways:
Using tsconfig :
- By invoking
tsc
with no input files, in which case the compiler searches for thetsconfig.json
file starting in the current directory and continuing up the parent directory chain. - By invoking
tsc
with no input files and a--project
(or just-p
) command line option that specifies the path of a directory containing atsconfig.json
file, or a path to a valid.json
file containing the configurations.
tsconfig.json☟
To connect the Apollo server to the client, all we need is your hosted server URL and a web socket link, which in my case is:
src/config/index.ts☟
Now it's time to finally start some coding. Navigate to the src
folder and create a file configureClient.js
where we will write all the configuration required to configure GraphQl Client.
src/configureClient.ts ☟
Now to add this configuration to your Next.js app navigate to the pages folder of your project directory and create a file with name _app.tsx
Note: Filename should be perfectly the same as _app.tsx
, nothing else.
_app.tsx ☟
That’s it. GraphQL Apollo Client Setup is complete….?
React makes it painless to create interactive UIs. Design simple views for each state in your application and React will efficiently update and render just the right components when your data changes. We will make a Next.js app with basic auth functionality using GraphQL.
So, Let’s start by creating a new app:
Folder Structure
Make the following folder structure to house everything:
Adding Dependencies
Now we will add all GraphQL dependencies required for react.js :
To connect the apollo server to the client all we need is your hosted server URL and a web socket link, which in my case is:
src/config/index.ts☟
Now it's time to finally start some coding. Navigate to the src
folder and create a file configureClient.js
where we will write all the configuration required to configure GraphQL Client
src/configureClient.ts ☟
Now to add this configuration to your react.js
app navigate to the src
folder of your project directory and edit App.tsx
src/App.tsx ☟
That’s it GraphQL Apollo Client Setup is complete.?
Conclusion
The main advantage of using this boilerplate is that every line of code is written in TypeScript. This is a programming language that’s used everywhere, both for client-side code and server-side code. With one language across tiers, there’s no need for context switching.
For tech stack with multiple programming languages, developers have to figure out how to interface them together. With the TypeScript stack, developers only need to be proficient in TypeScript and JSON.
Overall, using the MERN stack enables developers to build highly efficient web applications.
Book a Discovery Call
Dive deep into our research and insights. In our articles and blogs, we explore topics on design, how it relates to development, and impact of various trends to businesses.