Full Stack (MERN) + GraphQL Boilerplate / Starter Part-I
Author
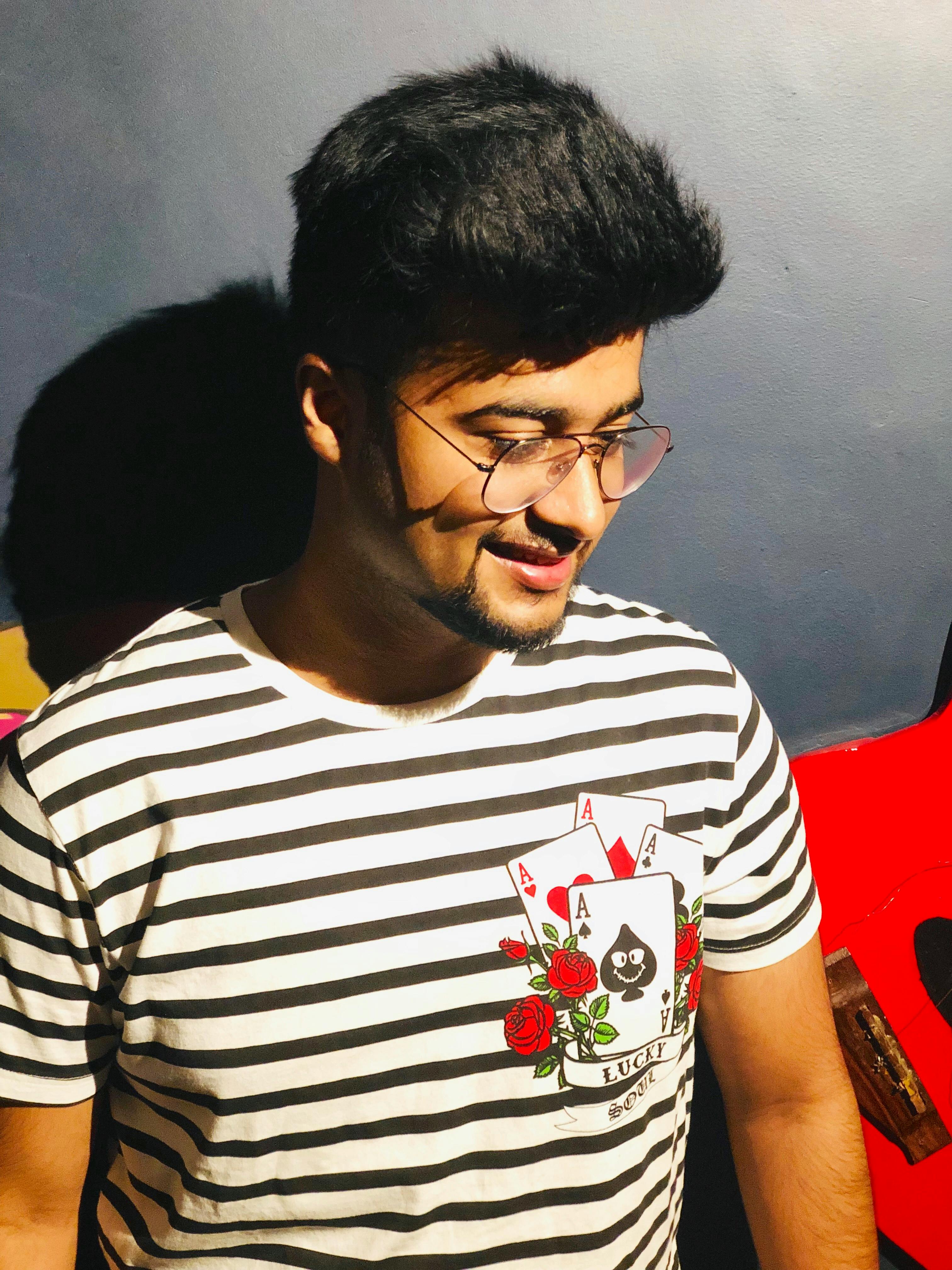
Date
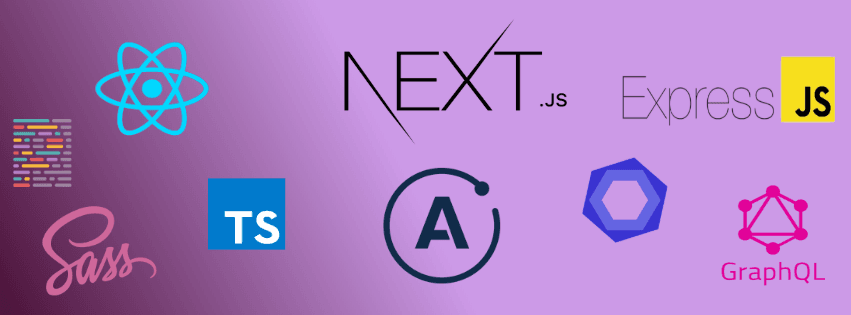
Book a call
Before we get started, I want to let you, the reader know, I plan to keep this updated for the foreseeable future. So, if something is broken, do leave a comment on the GitHub repo so I can fix it. I’m hoping to never have package installs with explicit version numbers unless absolutely necessary.
In this tutorial, we will create a next.js, react.js, and express app with apollo integration. I will be using MongoDB Database you can use whatever you find suitable for your project.
This is the first article of the Full Stack (MERN) + GraphQL Boilerplate / Starter series:
Repository Link: - https://github.com/garganurag893/Next.js_GraphQL_Express_Apollo_Boilerplate
Before we proceed we should know what features we are going to cover while learning from this tutorial.
Features.
GraphQL
GraphQL is a query language for APIs used for fulfilling queries with your existing data. GraphQL provides a complete and understandable description of the data in your API, gives clients the power to ask for exactly what they need and nothing more, makes it easier to evolve APIs over time and enables powerful developer tools.
Express
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications.
Next.js
Next.js extends React to provide a powerful method for loading a page's initial data, no matter where it is coming from. With a single place to prepopulate page context, server-side rendering with Next.js seamlessly integrates with any existing data-fetching strategy.
React
React makes it painless to create interactive UIs. Design simple views for each state in your application and React will efficiently update and render just the right components when your data changes.
React Apollo
React Apollo allows you to fetch data from your GraphQL server and use it in building complex and reactive UIs using the React framework. React Apollo may be used in any context that React may be used; in the browser, in React Native, or in Node.js when you want to do server-side rendering.
TypeScript
TypeScript is an open-source programming language developed and maintained by Microsoft. It is a strict syntactical superset of JavaScript and adds optional static typing to the language. TypeScript is designed for development of large applications and trans-compiles to JavaScript.
Why choose this boilerplate?
Github GraphQL API
You may be wondering why Github chose to start supporting GraphQL. Their API was designed to be RESTful and hypermedia-driven. They’re fortunate to have dozens of different open-source clients written in a plethora of languages & businesses grew around these endpoints. Like most technology, REST is not perfect and has some drawbacks. Their ambition to change their API is focused on solving two problems:
Read more about this here: https://github.blog/2016-09-14-the-github-graphql-api/
GraphQL At Artsy
Their team started using a GraphQL orchestration layer that connects various APIs with multiple front-end apps including iOS. It also handles caching and extracts some business logic out of their client apps. This helped them not only to be more consistent with the way they fetch data across apps but also improved developer happiness and even bridged teams by having their web and iOS developers work with the same API layer.
Read more at: https://artsy.github.io/blog/2016/11/02/improving-page-speed-with-graphql/ & https://www.graphql.com/case-studies/
Why choose Apollo for GraphQL?
Apollo platform is an implementation of GraphQL that can transfer data between the cloud (server) to the UI of your app. In fact, Apollo builds its environment in such a way that we can use it to handle GraphQL on the client as well as the server-side of the application.
Setting Up The Server:
So, Let’s start by creating a new project directory :
Navigate to project directory and run command :
Installing Dependencies:
Now we will install all the dependencies which are required for the basic setup as mentioned below:
- Bluebird:- To promisify all the Mongoose.
- Mongoose:- It manages relationships between data, provides schema validation and is used to translate between objects in code and the representation of those objects in MongoDB.
- Express:- To set up the server.
- Apollo-Server-Express:- Apollo Server is a community-maintained open-source GraphQL server that works with many Node.js HTTP server frameworks.
Install dependencies using the command:
Folder Structure
Make the following folder structure to house everything:
Adding TypeScript:
TypeScript is a typed superset of JavaScript that compiles to plain JavaScript.
The presence of a tsconfig.json
file in a directory indicates that the directory is the root of a TypeScript project. The tsconfig.json
file specifies the root files and the compiler options required to compile the project. A project is compiled in one of the following ways:
Using tsconfig :
- By invoking
tsc
with no input files, in which case the compiler searches for thetsconfig.json
file starting in the current directory and continuing up the parent directory chain. - By invoking
tsc
with no input files and a--project
(or just-p
) command line option that specifies the path of a directory containing atsconfig.json
file, or a path to a valid.json
file containing the configurations.
tsconfig.json☟
Environment Setup:
Before we begin coding, we should set up the development environment.
Dotenv is a zero-dependency module that loads environment variables from a .env
file into process.env
.
config/index.ts☟
After setting up the environment, let's proceed by setting up the Schema.
GraphQL Schema Setup:
It is required by GraphQL as every GraphQL service defines a set of types that completely describe the set of possible data you can query on that service. Then when queries come in, they are validated and executed against that Schema.
server/graphql/schema/index.js ☟
Now to complete the GraphQL setup we have to add Resolvers.
Adding GraphQL Resolvers:
It provides the instructions for turning a GraphQL operation (a query, mutation, or subscription) into data. They either return the same type of data we specify in our schema or a promise for that data.
server/graphql/resolvers/index.ts ☟
server/graphql/resolvers/user.ts ☟
To save time, I created a function for transforming user return objects so that I can call it every time I need to return a user object.
That's it! The GraphQL Setup is complete. Now it's time to configure express to set up the server.
Setting Up Express:
Setting up express is not difficult at all. Create a .ts file in the config folder and follow the code below:
config/express.ts☟
Now create a file index.js in the project directory where we will connect our database to the server.
index.ts☟
That’s it. Run the server and go live.?
Visit the below link to learn about the Next.js Client Setup ☟
https://geekyants.com/blog/full-stack-mern--graphql-boilerplate--starter-part-ii
Book a Discovery Call
Dive deep into our research and insights. In our articles and blogs, we explore topics on design, how it relates to development, and impact of various trends to businesses.