Table of Contents
Typescript: Utility Types
Author
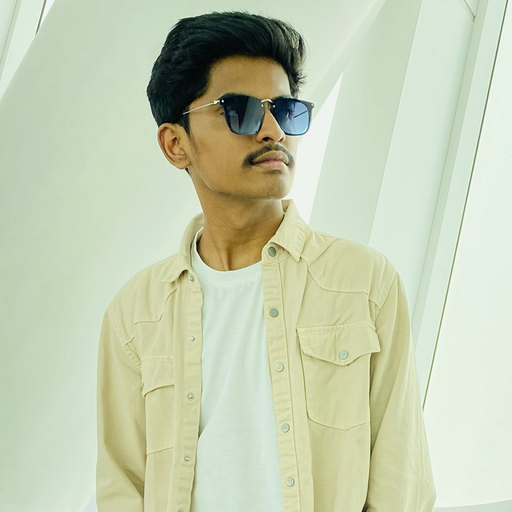
Date
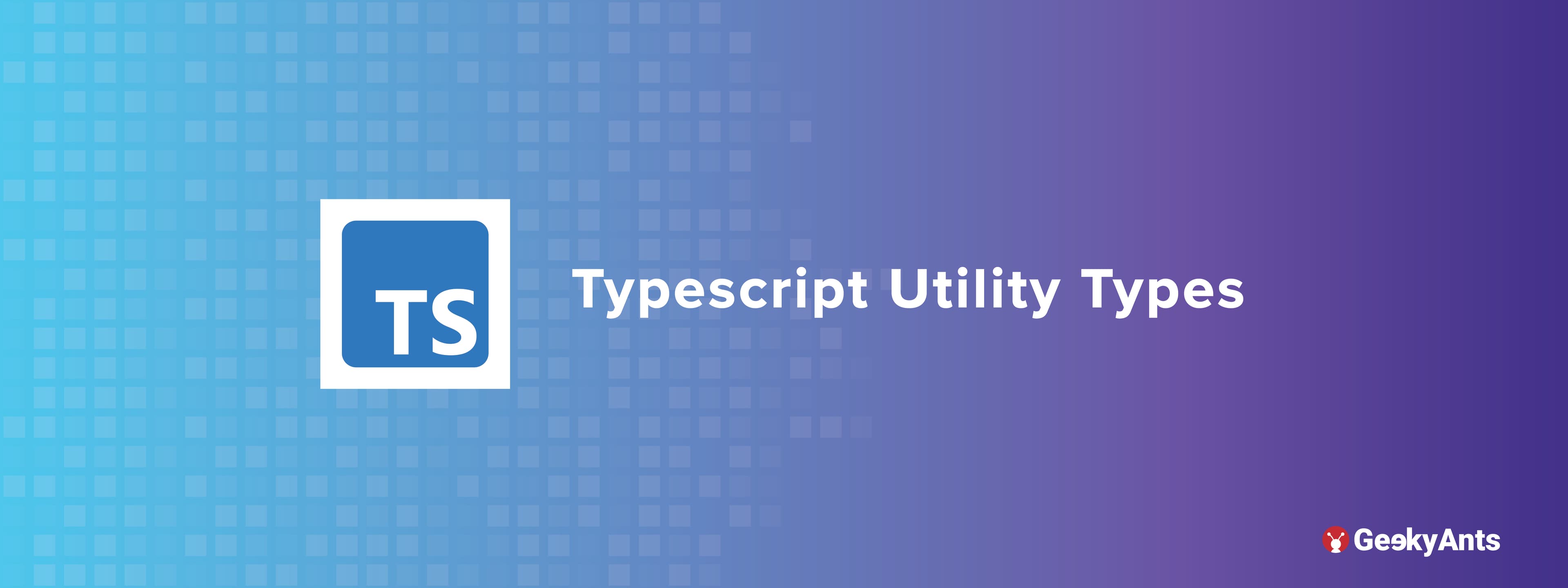
Book a call
Developers frequently claim that typescript requires them to write redundant code. The reasons can vary, but one of the most common is a failure to use utility types.
Types are analogous to utility functions in Javascript.
They are provided in typescript to make our lives easier. Let's take a look at them one at a time.
1. Partial
Partial<T>
allows you to create optional props with a single line of code. Without it, we'd need to duplicate the User type, which isn't ideal.
Below is an example of Partial
utility type.
Partial
takes type as an argument.
2. Required
Required<T>
is the inverse of Partial. It creates all the necessary props.
Required
also takes type as an argument.
3. Readonly
In JavaScript, readonly
functions similarly to const. You cannot reassign a type once it has been marked as readonly
.
4. NonNullable
NonNullable<T>
filters your type and removes null and undefined from the union of types.
5. Exclude
Exclude<T, K>
lets you remove type from the union types.
It takes two arguments. The first is type, while the second is a union of props.
6. Extract
Extract<T, K>
is the inverse of Exclude
. It will generate union types based on the specified union type.
7. Pick
Pick<T, K>
picks props from type. It will give you a new type.
8. Omit
Omit<T, K>
removes props from types.
9. ReturnType
ReturnType<T>
gives return type of function.
10. Record
Record<T, K>
creates an object type.
Instead of doing this:
You can do this:
Record also accepts union type as key that is not supported by interface and type.
As we can see, utility types help in avoiding redundancy in the codebase. It also enables maintaining a single source of truth.
That's it for today. I hope you enjoyed this blog. Stay tuned for upcoming blogs on the typescript.
Dive deep into our research and insights. In our articles and blogs, we explore topics on design, how it relates to development, and impact of various trends to businesses.