TypeScript: Building Blocks
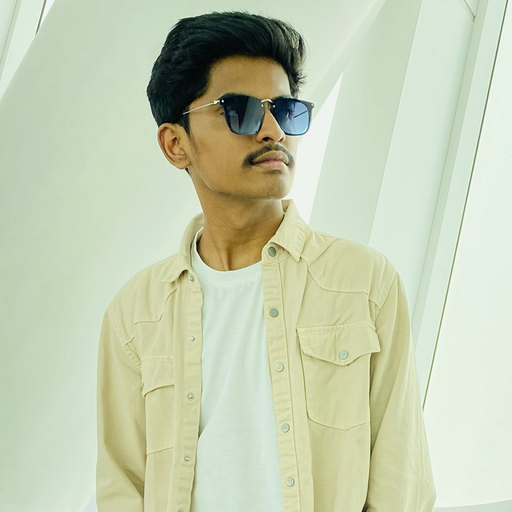
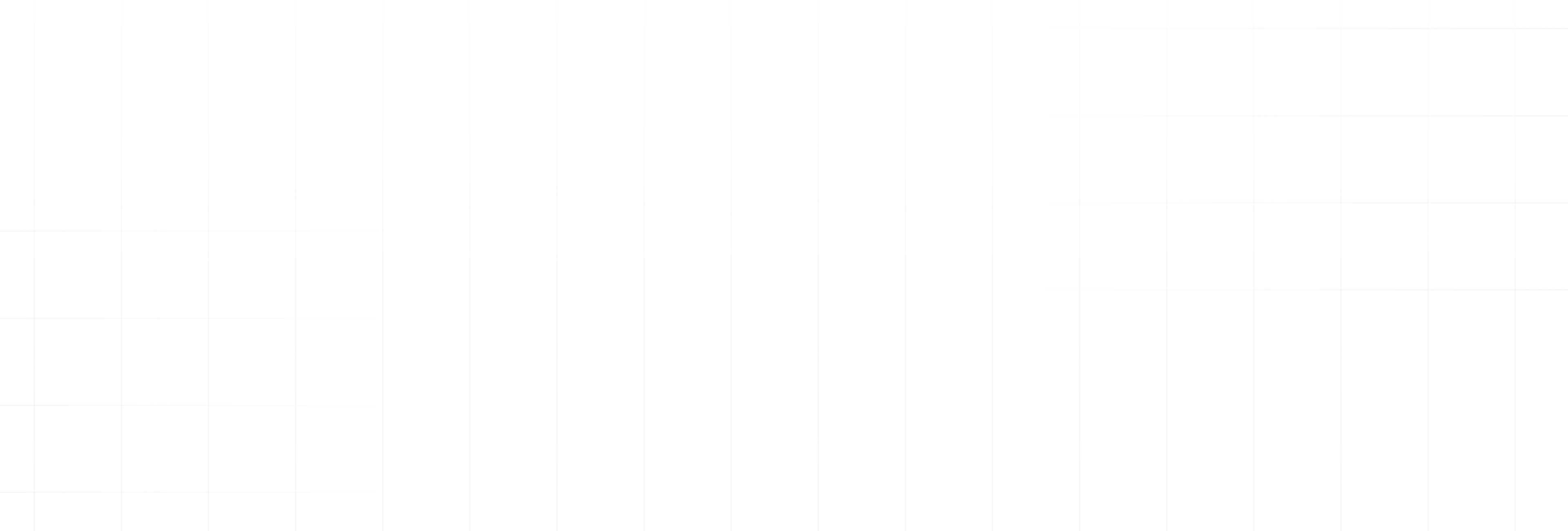
Type Annotations
TypeScript contains various annotations similar to data types in any static language in the form of strings, numbers, boolean, arrays, records, etc.
You can assign a type annotation to variables, functions arguments, etc.
Primitive Types
The cool thing about primitive types is that you don't need to manually assign any type when manually setting a default value.
In the code above, TypeScript will ensure that name always has a string type of value. This is referred to as an infer type.
You can begin writing code by visiting the typescript playground.
Let's see what happens when we assign a number to a name!
You will see the below message.
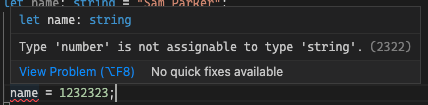
Type Safety in Functions
Let's understand what's going on here.
We are passing
name
as astring
argument. So the data type ofname
can only be astring
.At the end of the
)
bracket, we have added anotherstring
annotation. It will make sure thatgetEmailAddress
will always returnstring
type of data.
This is the beauty of TypeScript. Without even running the code, the code can easily be understood.
Let's see how we can provide type safety for traditional functions.
Non-Primitive Type Annotations
Typescript provides type safety to primitive types like array and objects.
The code above, looks similar to primitive code. We have added [] at the end of the string type annotation. This will let us add only string type of data to the array.
Examples of Non-Primitive Type Annotations
Tuple
A tuple is very similar to an array in Javascript. Typescript lets us be more specific while defining array-type annotations.
Let's see how we can use a tuple.
You can use a tuple to have multiple data types in an array at a specific location.
What about the objects, though?
To provide type safety for object data type, TypeScript offers different ways of implementation.
The syntax looks similar to how we create objects in JavaScript
.
We can make it reusable by creating interface
and type
.
interface
- type
Although they serve different purposes, interfaces
and types
function in the same way.
An interface
can extend another interface
in the same way that classes do.
types
are used to modify other types. It is known as types of types.
Optional Types
You only want to pass all of the arguments in rare instances, such as when an object contains optional properties. We can mark type annotations as optional. Let's see how we can do that.
When you run this code, you can skip the second argument. There will be no type error.
?
is used to make an optional type.
In functions, you need to pass optional types after the usual types, in order to avoid confusion.
Similarly, we can use ?
with interface
or type
to make some props optional.
You can use the same syntax for type
as well. All you need to do is add ?
after :
. This way you can create flexible types.
Type Operations
With TypeScript, we can modify types through a few operations. We can create another kind by combining different types. We don't need to write any extra code to accomplish this.
1. Intersection
An Intersection works as a logical and
operator. We can combine more than one type to create another type.
Use &
to perform intersection operations.
2. Union
Union works as a logical or
operator. We can combine types, but those types are optional.
Unlike intersection
, we can skip some types properties.
Use |
to perform union operations.
Generic Type
So far, we have seen how to create types statically, but what if we could create dynamic types? That's where the generic type comes into the picture.
Let's understand what is happening here. We have discovered a new syntax here in <T>
.
This is a signature of generic type. You can pass and accept type in <>
brackets.
- Here we are passing type
T
to the function like we pass an argument. - After that we are assigning that type
T
to the argument. Finally, the syntax will look like this.
<T>(args: T)
Generic type with traditional functions
Similarly, we can use generic types for non-primitive data types. Here's an example of how you can use generic types with objects.
Think generic type as functions of JavaScript. We can take type T
as an argument and then we can use that anywhere we want.
So far, we've looked at Typescript's fundamental building components.
The adventure does not end here. We'll delve deeper into typescript.
In the future blogs, we'll look at typescript tools, kinds of types, and conditional types.
Thank you for taking the time to read this blog.