Table of Contents
In-App Analytics In React Native
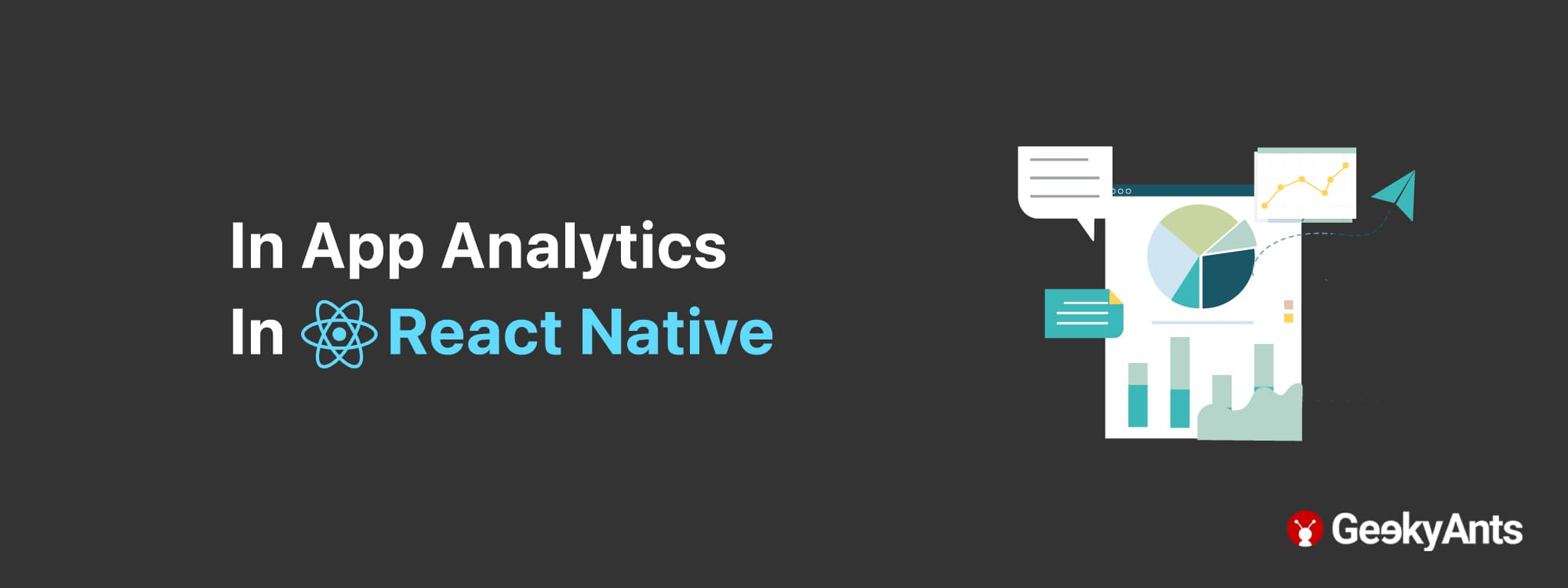
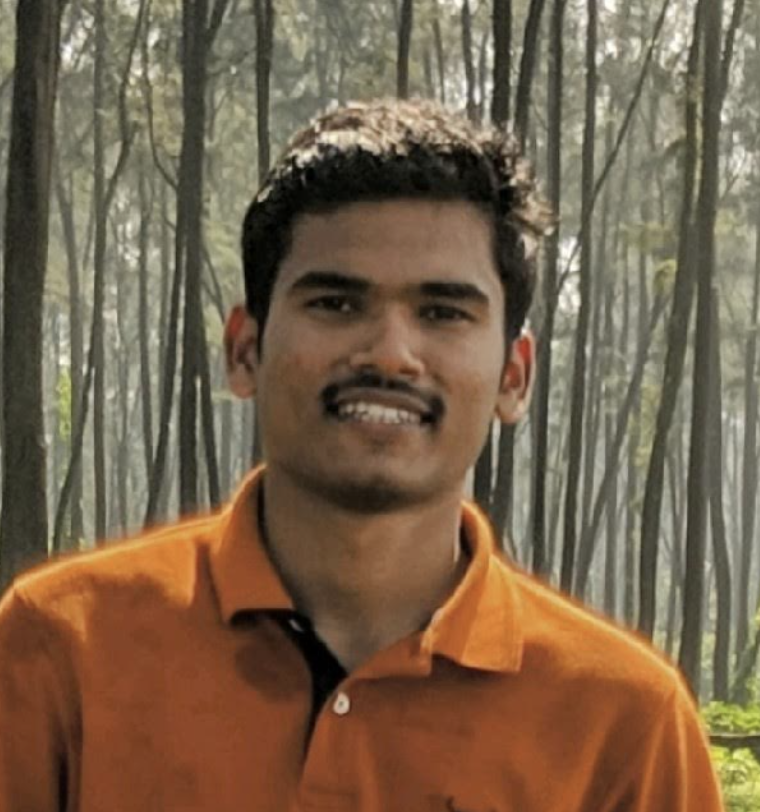
Book a call
What Is In-app Analytics?
Collection of data from mobile apps based on different user actions while using the app. And analyzing that data to get insights into user behavior patterns.
Why Do We Need It?
We use it to analyze the user behavior pattern on certain events.
For example:
- Which button is pressed by the user, and how many times has the user pressed it
- Which option is frequently selected by the user
- Which screen is viewed by the user, and what information is the user able to get
Types of Analytics Events
- Screen view event: When the user has opened any screen, she/he can see the information on that screen.
- Screen action event: Whenever the user performs an action on-screen, by pressing a button or anything similar.
How To Implement It In React Native?
In React Native, we can achieve this by using third-party supported analytics tools libraries. Like,
- Firebase analytics
- App Center Analytics
- Segment Analytics
Now, let us take a look at it by using these libraries.
1. Firebase Analytics
Firebase provides various options to collect data from the app. Let’s have a look at two basic types of it: logScreenView and logEvent.
First, let’s install and configure firebase in the React Native app. You can use the react-native-firebase/app package. Please go through these steps to complete the setup.
A. Screen view When the user opens this screen, then data will be collected on which screen has been viewed by the user.
B. Screen action When the user presses any category option, data will be collected on which category the user has selected.
Firebase also provides some other predefined methods for analytics.
App Center Analytics
App Center Analytics is an analytics tool developed by Microsoft.
App Center provides a method called trackEvent to collect the data for both screen view events and screen action events.
First, let’s install the app center package in our react native project.
yarn add appcenter appcenter-analytics appcenter-crashes --exact
For further configuration and setup in the project, follow these steps.
Let’s look for a code for the previous examples that we have seen.
A. Screen View
B. Screen Action
Segment Analytics
Segment analytics is another package available for React Native for in-app analytics, similar to what you have seen in the above two packages.
First, let’s install the segment analytics package in the React Native project.
yarn add @segment/analytics-react-native @segment/sovran-react-native @react-native-async-storage/async-storage
For further configuration and setup in the project, follow these steps.
I am sharing the implementation for the previous example.
A. Screen View
B. Screen action
This is how you can set up analytics in the React Native app. If you observe, a similar pattern is used by all these libraries, so it's easy to understand its implementation.
Thank you. 😊
Dive deep into our research and insights. In our articles and blogs, we explore topics on design, how it relates to development, and impact of various trends to businesses.