Debugging High-CPU Usage in Full-Stack Applications: A Comprehensive Guide
Author
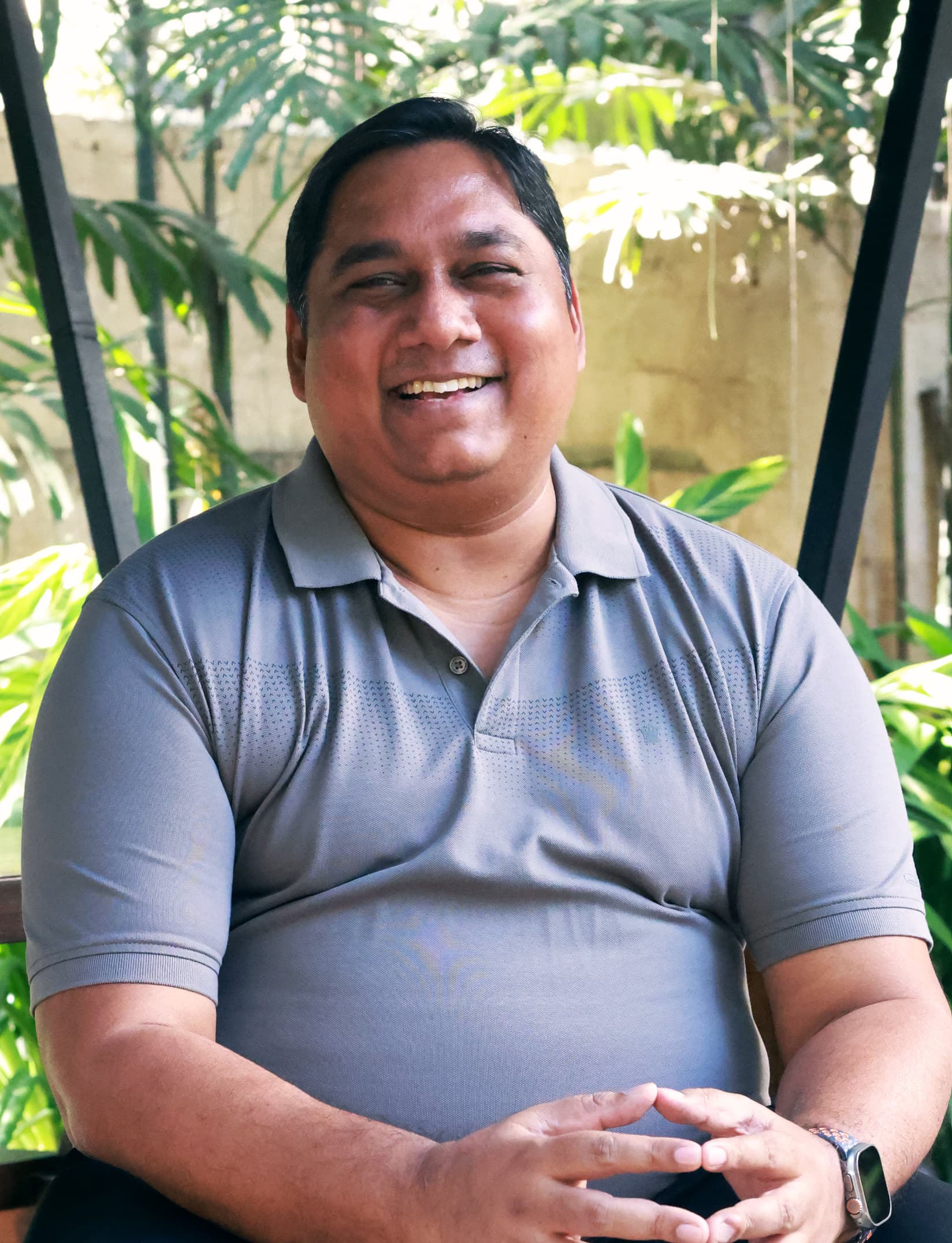
Date
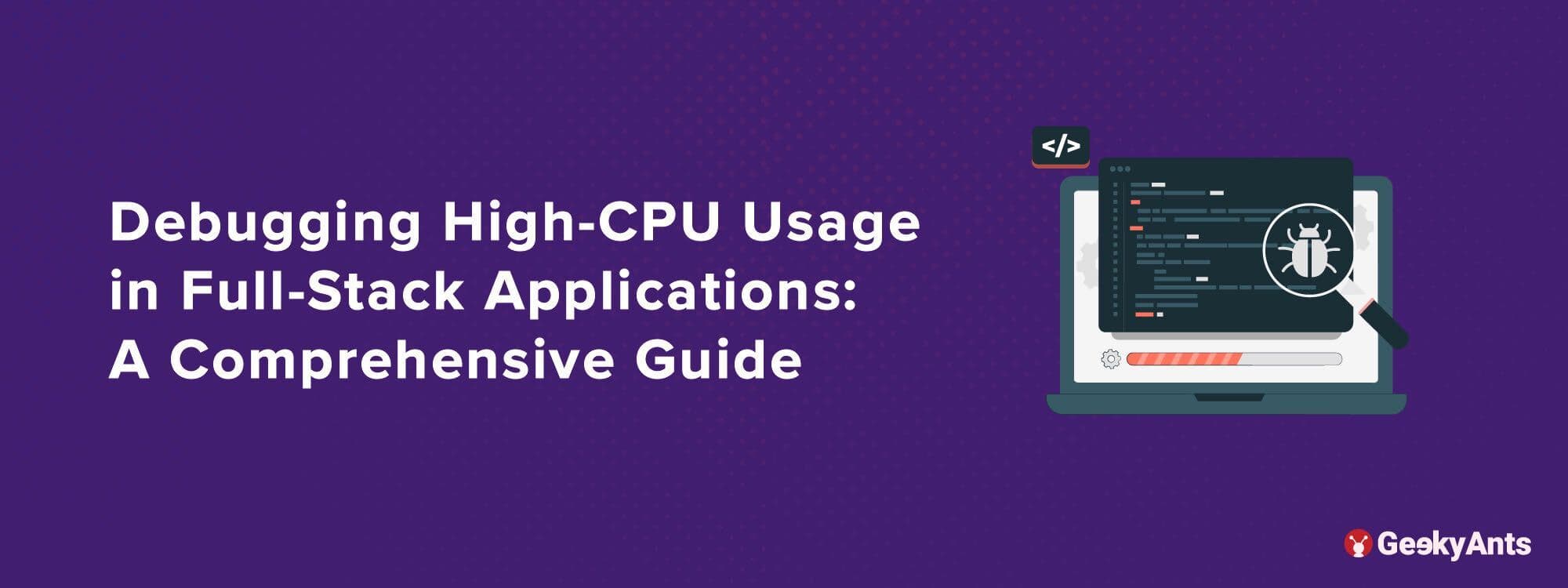
Book a call
In full-stack applications, high CPU usage can significantly impact performance and user experience. This blog post provides a comprehensive guide to debugging high CPU usage, covering issues at the database, API, and frontend levels.
Identifying Postgres Database Issues
When both the Node.js and Postgres layers experience high CPU usage, start by looking into the Postgres server. Consider the following steps:
a. Optimize queries: Ensure that all queries are optimized to avoid unnecessary processing.
b. Indexing: Proper indexing can vastly improve performance, so make sure your database is correctly indexed.
c. Connection management: If there's a high rate of connection creation and deletion, use connection pooling to reduce overhead. This can be implemented on the server-side or in the Node.js client.
d. Hardware limitations: Check if the server's hardware resources are adequate for the workload. Upgrading hardware or scaling the infrastructure might be necessary.
Identifying the Root Cause of High CPU Usage
Once you've ruled out database issues, try to identify the primary cause of high CPU usage. This can be due to bulk read operations or too many write operations. Implement queueing for writes or caching for reads to address these issues, while ensuring that the application remains unaffected.
Debugging Node.js API Layer Issues
If the Node.js API layer has high CPU usage while Postgres does not, investigate your code for potential issues. Since Node.js is single-threaded, look for blocking code, such as for-loops and array reads. Consider preprocessing data and storing it in a cache or directly in the database. Additional steps include:
a. Profiling: Use profiling tools to identify CPU-intensive functions or modules in your code.
b. Parallelism: Implement parallelism using worker threads or clustering to distribute the workload across multiple cores.
c. Event-driven architecture: Embrace asynchronous programming and event-driven architecture to avoid blocking the main thread.
d. Code optimization: Refactor and optimize your code for better performance, removing unnecessary loops or function calls.
Addressing Frontend Issues
Unnecessary API calls from the frontend can contribute to high CPU usage. Implement the following strategies to reduce calls:
a. Local storage: Use local storage to cache information and reduce the number of calls made to the backend.
b. Debounce: Implement debounce techniques to prevent excessive API calls due to user actions, such as dragging an item.
c. Throttling: Throttle user-generated events to limit the rate of API calls.
d. Lazy loading: Implement lazy loading for heavy assets or components to minimize resource consumption.
Conclusion
Debugging high CPU usage in full-stack applications can be a complex process, but by systematically analyzing each layer and implementing best practices, you can significantly improve performance. Keep in mind that optimizing your application requires a thorough understanding of its architecture and potential bottlenecks. Remember, the key is to remain patient and persistent in your debugging efforts. Always test and monitor your application to ensure that the implemented changes lead to the desired improvements.
Dive deep into our research and insights. In our articles and blogs, we explore topics on design, how it relates to development, and impact of various trends to businesses.