Creating Classic Bluetooth Turbo Module Using React Native New Architecture
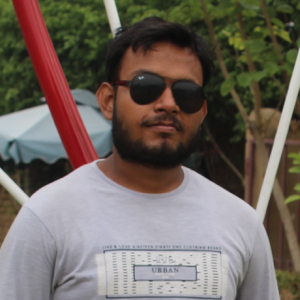
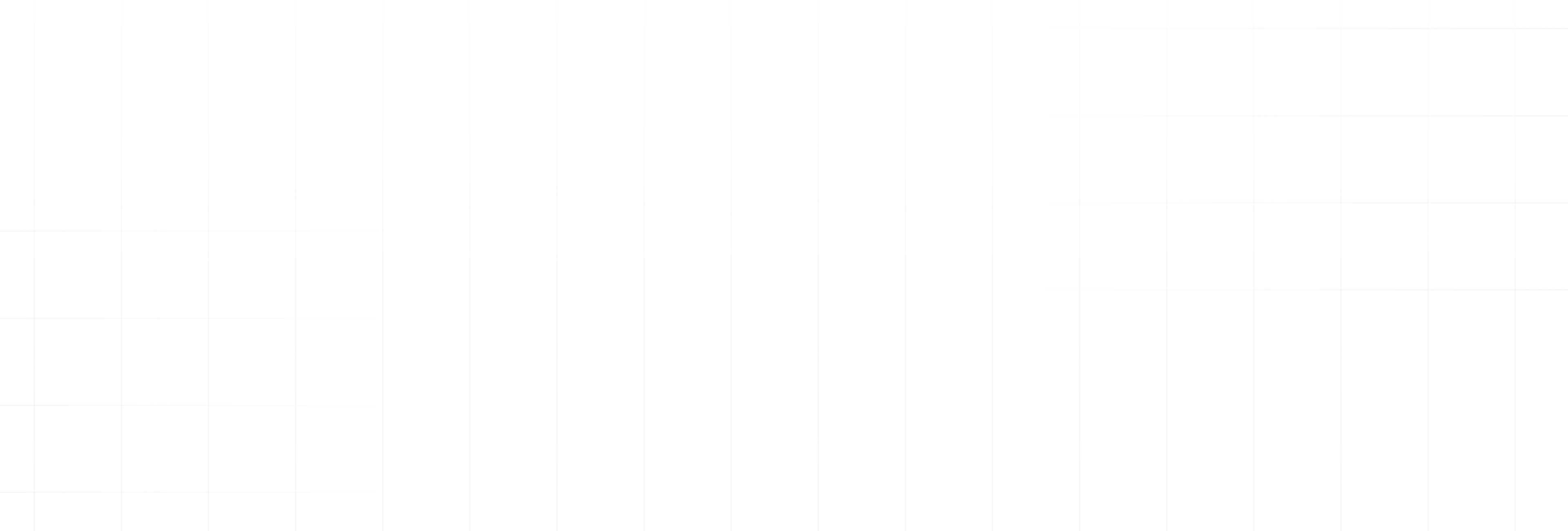
Introduction
This article discusses the creation of a Bluetooth module using React Native's new architecture. The module is designed to enable Bluetooth communication between mobile devices and other Bluetooth-enabled devices.
New Architecture
React Native's new architecture is designed to improve performance and reliability in mobile applications. It includes several new features that make it easier for developers to build high-quality apps, including:
- Fabric: A new architecture for rendering views that improves performance and memory usage
- TurboModules: A new system for building native modules that improves start-up time and reduces memory usage
- WebView: A new implementation of WebView that is faster and more reliable than the previous version
By using these new features, developers can build Bluetooth modules and other features that are faster, more reliable, and consume less memory on mobile devices.
Performance Comparison
Physical Device: Google Pixel 4
Scenario | Old Architecture | New Architecture | Difference |
---|---|---|---|
1500 View components | 282ms | 258ms | New Architecture is ~8% faster |
5000 View components | 1088ms | 1045ms | New Architecture is ~4% faster |
1500 Text components | 512ms | 505ms | New Architecture is ~1% faster |
5000 Text components | 2156ms | 2089ms | New Architecture is ~3% faster |
1500 Image components | 406ms | 404ms | New Architecture is neutral with Old Architecture |
5000 Image components | 1414ms | 1370ms | New Architecture is ~3% faster |
Bluetooth Classic Demo
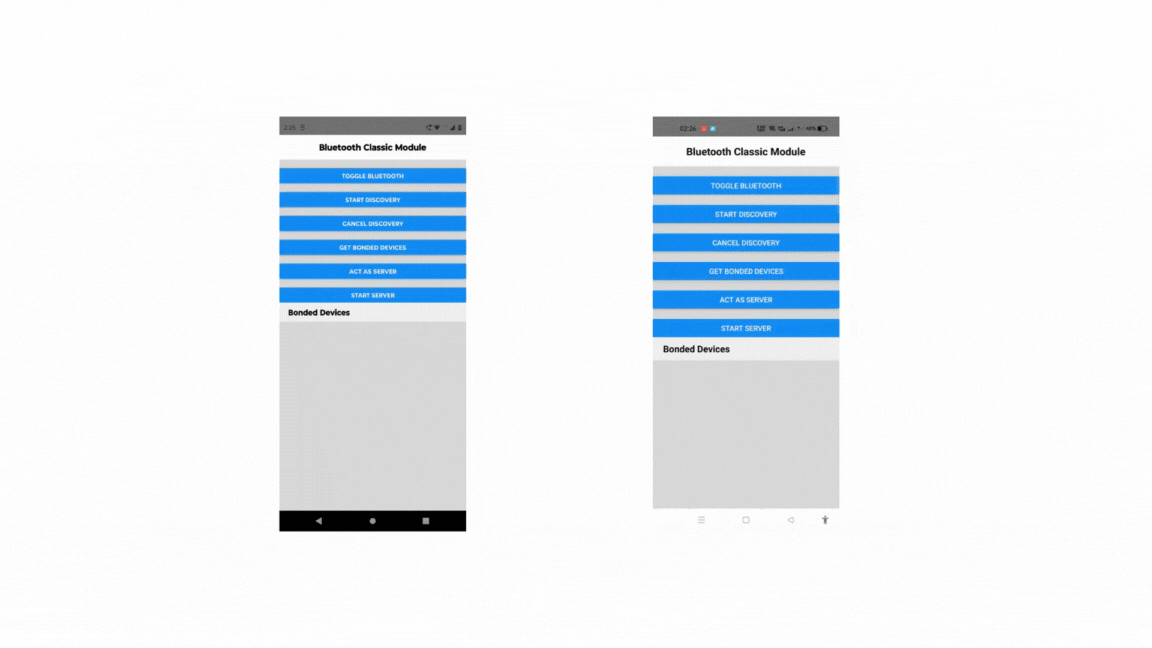
Steps for Creating a Turbo Module for Bluetooth (Android)
- Define the JavaScript specification.
- Configure the module so that Codegen can generate the scaffolding.
- Write the native code to finish implementing the module.
Folder Setup
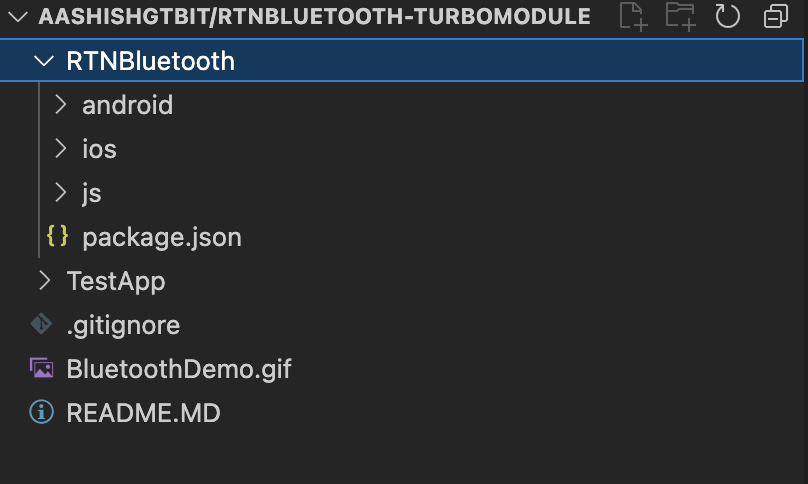
Defining Javascript Specification
Create a file in js/NativeBluetooth.ts
and add the following contents. The file name should follow the Native<ModuleName>.ts
. The exported Name should be Spec
.
Create a Package.json
file at the root of the project. Here we have added the basic details for our package and also added the Codegen
config, which contains the Java package name
for the android modules. Here the name
field should follow the particular naming convention RTN<PackageName>Spec
.
Creating Android-specific Changes
In the Android folder create a build.gradle
file with the following content build.gradle
Generating Android Code Using Codegen
From the root of the project, run the following script. Before that, please create a React Native basic app using react-native init
template with the name TestApp
. It will add the rtn-bluetooth
package to the node-modules. You can find the generated code in the following path TestApp/node_modules/rtn-bluetooth/android/build/generated/source/codegen
. The Codegen helps us to generate a lot of scaffolding code, which will save us time.
In the Android folder, create the following folder structure. src/main/java/com/rtnbluetooth
Create a file inside rtnbluetooth folder named [BlcManager.java](<https://github.com/Aashishgtbit/RTNBluetooth-TurboModule/blob/main/RTNBluetooth/android/src/main/java/com/rtnbluetooth/BlcManager.java>)
. Here we will add the logic for checking the Bluetooth support and enabling and disabling Bluetooth support.
Create BlcManager Class
In the above code, we have created a basic Java class that extends ReactContextBaseJavaModule
, and here in the constructor method, we are updating the value of reactContext and also attaching activityEventListener with the context. We will use this activityEventListener for listening to the opening and closing of activity on the native side, like opening of the Bluetooth permission dialog.
Here we have added the getBluetoothManager
and getBluetoothAdapter
, which will provide the bluetoothAdapter instance that exposes different APIs of Bluetooth like getBondedDevices
method (which will provide the list of already paired devices) , methods for enabling
and disabling
Bluetooth.
Finally, we have added checkBluetoothSupport
method, which will return a boolean promise based on Bluetooth availability on the device.
Enabling Disabling Bluetooth
Here we will expose one more public method toggleBluetooth
that will toggle the Bluetooth states. Here we are showing toast while disabling the Bluetooth, and if the Bluetooth is disabled, we are enabling it by asking the user for the BLUETOOTH_CONNECT
permission by checking the current BuildSDK version. If we have the required permission It will create an intent that will ask the user to enable Bluetooth.
After that, we will override onActivityResult
from the interface ActivityEventListener
. It will listen for the activity events like when the user either accepted or denied the Bluetooth opening request, and based on the request code we are sending the appropriate message and toasts.
Create BluetoothModule Class
Here we will create [BluetoothModule.java](<https://github.com/Aashishgtbit/RTNBluetooth-TurboModule/blob/main/RTNBluetooth/android/src/main/java/com/rtnbluetooth/BluetoothModule.java>)
file in the rtn-bluetooth
folder, which will call the Methods used in the BlcManager
class. This module extends the NativeBluetoothSpec
class, which is generated by the codegen
using the js/NativeBluetooth.ts
file exported Spec.
Now we will add the BluetoothPackage.java
class that will extend TurboReactPackage
class. This class uses the BluetoothModule we created by overriding methods from the TurboReactPackage
abstract class.
Finally, We need to make sure that we have enabled new architecture in our TestApp. We can enable it by going to TestApp/android/gradle.properties
and setting newArchEnabled=true
.
In our TestApp, we need to make sure that we have the following Bluetooth permission in our TestApp/android/app/src/main/AndroidManifest.xml
.
If we are making any changes to our RTNBluetooth
folder, we have to run the following command from the root of the project.
React Native Side Usage
App.tsx
Summing Up
🎉Woohooo… 😎 We have finally completed the basic Bluetooth classic module, which can handle the enabling and disabling of Bluetooth. In the same way, we can expose other Native Android APIs to the React native.
For the complete code with more functionality, you can visit https://github.com/Aashishgtbit/RTNBluetooth-TurboModule repo. I hope you liked this article, and if you did, please share it with your friends. For any queries, please connect with me on Twitter.
References:
Book a Discovery Call.
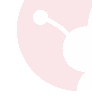