Creating a Chat Application using Vue-Native and Firebase
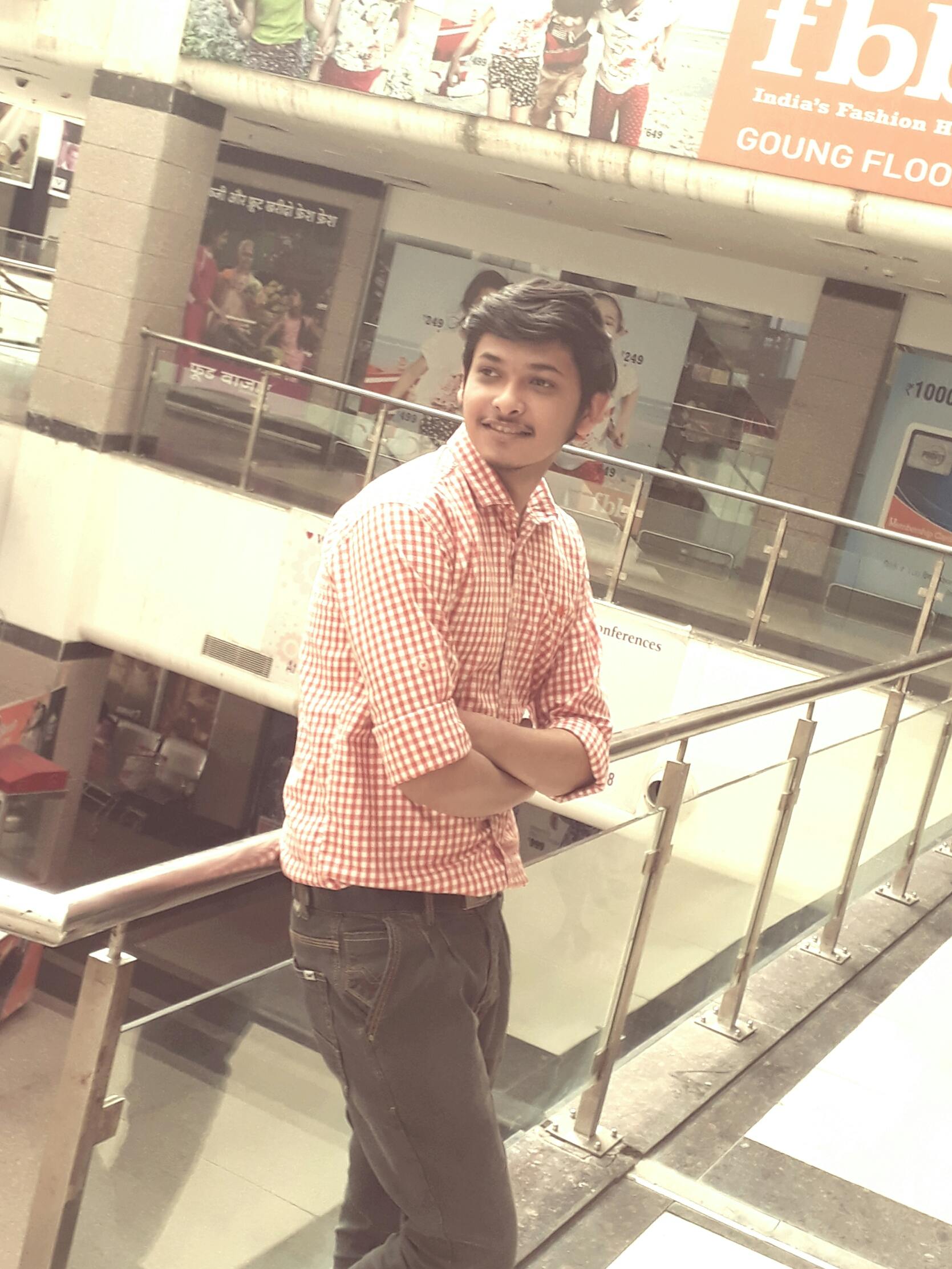
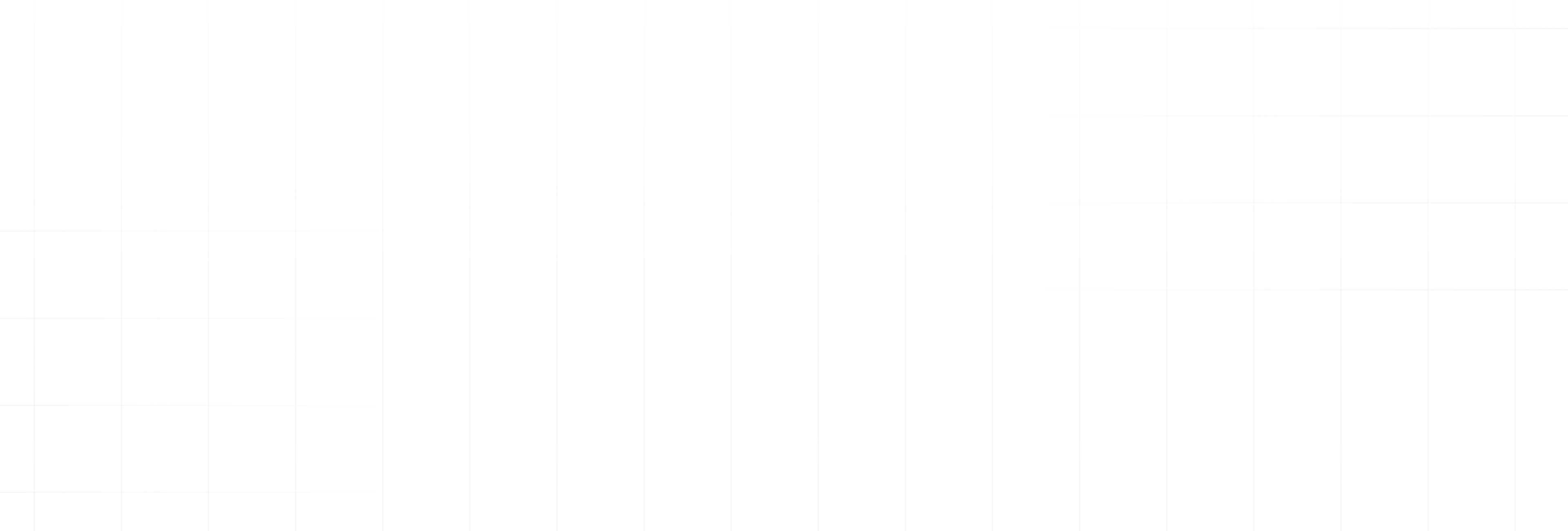
Vue Native is a framework created to bring the best of VueJS to build hybrid mobile apps.
In this tutorial, we are going to build a chat-application using Vue-Native and Firebase as a backend service. This project will include some basic features of a chat application. First of all, users will have to get through the authentication layer of the app to access their account and then they will be able to do real-time chat with their contacts. Before starting the steps to integrate Firebase, lets see some advantages of Vue-Native.
Internally, Vue-Native uses the React Native API which means getting benefits of both Vue and React Native properties under a single hood. Since Vue Native is based on VueJs, it gives developers the freedom to code in a template based syntax, like web, which makes transitioning to mobile based frameworks trouble-free. Firebase, on the other hand, is a powerful backend tool for building great mobile apps with services like crashlytics, authentication, storage etc.
Integrating Firebase with Vue Native is not as tough as it may sound. Following the below steps, you will be able to integrate Firebase with Vue Native in no time. If you are a newbie in Vue-Native, this link will help you with the installation and initial project setup.
Installing Firebase Dependency:
Open your Vue-Native project in the terminal and type the below commands to install firebase package.
npm install react-native-firebase
or
yarn add react-native-firebase
react-native link react-native-firebase
To achieve chat application features, we will be needing:
Firebase will provide us with all the above services.
Project Directory Setup:
Firebase Project Setup:
Firstly, sign up for the Firebase console and create a new project using the ‘+’ button in it. Enter the project's name and click on the 'create project' button. After creating a successful project, open the project's dashboard.
For creating a basic chat-app, we will create two collections in Firebase database. One for the Users information and one for the messages.
Android Setup:
After successful creation of project you will see 'add app +' icon on the project dashboard.
Click on it and add the android app. Post that, a list of steps will appear. Follow all the steps carefully shown in the dashboard to integrate it successfully in Android. Then:
On the top of this file, add apply plugin:'com.google.gms.google-services'
. Also add multiDexEnabled true
in the defaultConfig
object.
classpath 'com.android.tools.build:gradle:3.4.0'
to classpath 'com.android.tools.build:gradle:3.4.1'.
google()
in allprojects/repositories
module in build.gradle file.
new RNFirebaseAuthPackage()
, new RNFirebaseFirestorePackage()
inside the getPackages()
function.
react-native run-android
in the terminal to check if everything is working correctly.Log in to firebase console and you will see the android app icon with your project's information.
The Android integration journey is now complete. Pretty easy, right? I assure you, the iOS integration is even easier.
iOS Setup:
Open your project in the Firebase console, click on 'add app +' button and select iOS. A new screen will open.
Then:
pod install
.Open project.xcworkspace file of your project and click on play button. Check in the Firebase console for iOS app to see whether integration was successful.Wow!! Integration completed.
Vue Native is now completely integrated with Firebase. Now, we can make a small chat application.
Creating Registration and Sign In functionality:
Let's start by creating the authentication flow for the chat application. We will create a component named Register and Login in Register.vue
and login.vue
file respectively inside the components folder. You can check the basic Vue-Native components to create a basic template.
For using Firebase authentication service, we will be using email/password type authentication.
firebase.auth().createUserWithEmailAndPassword(email, password)
firebase.auth().signInWithEmailAndPassword(email,
password)
firebase.auth().signOut()
Creating contacts screen and its functionality:
After the authentication screen, let's start creating the 'contacts' screen functionality. Let's create a file named
Users.vue
for Users component inside the components folder.
getUsers()
function in mounted()
. This function will be called after the component renders for the first time.Creating Chat Screen and its functionality:
Now we are only left with chat functionality. For that, we will create the final component in file called Chat.vue
inside components folder.
For receiving messages, Consider the below function `addListener()
`.
Since we have added the messages
variable to data, it becomes reactive. Now for every change in messages array, there will be change in the UI or the view. At the end, import all components in App.vue to conditionally render according to user existing or not. Also you can simply use vue-native-router for navigation b/w screens.
If you followed the above steps correctly, a simple demo for the chat application using Vue Native & Firebase is ready for you.
Check out the full project on https://github.com/anmoljain10/chat-app-vue-native
It contains all Vue Native components and also the authentication layer for the app. If there are any queries or suggestions that you have, please leave them in the comments section on GitHub and I will respond to as many as I can.
For more information about Vue Native, you can go to this link.
Thank you for reading!
Book a Discovery Call.
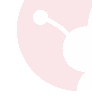