Accessing MongoDB Purely via Dart
Author
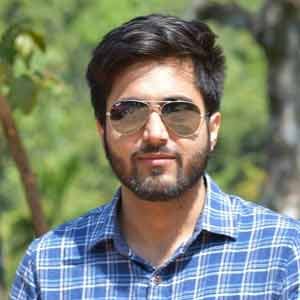
Date
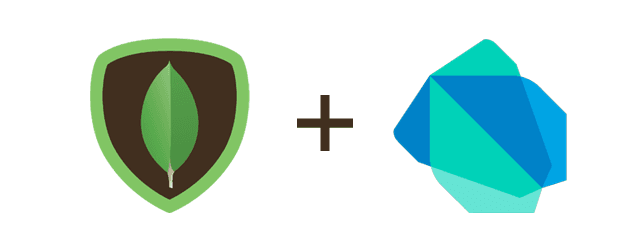
Book a call
While developing an app or a website, you most probably need to have a storage mechanism that can store user-related data or even the data which is used to show some kind of UI on the screen. Choosing a particular database that fully meets your project requirements can be tricky. Generally, the most important aspects that a developer looks for in a database are storing dynamic structured data, high scalability, and fast performance.
These requirements can be fulfilled by using a NoSQL database and having a powerful database like MongoDB really comes in handy.
Why use MongoDB?
The answer to this question is - to simply go further and faster while developing software applications that have to handle data of all sorts in a scalable way. Just to have an idea, thousands of companies like Bosch, Barclays, and Morgan Stanley run their businesses on MongoDB and use it to handle their most demanding apps in areas like IoT, Gaming, Logistics, Banking, e-Commerce, and Content Management.
For using the database, you need a programming language to access the database and perform queries on it. Mostly, Dart is used for making user interfaces with Google's UI software development kit Flutter, but it can effectively be used as a backend language due to its, single-threaded, garbage-collection, and null-safety features.
Together, Dart and MongoDB can be seen as a killer-combination for developers!
Dart & MongoDB
For those who don't have much idea about Dart and MongoDB, here is a brief:
MongoDB is an open-source, cross-platform, document-based-NoSQL database management system that stores data in the form of documents and collections and uses a JSON-style storage format known as binary JSON, or BSON, to achieve high throughput. BSON makes it easy for applications to extract and manipulate data. For more info please click here.
Dart is an open-source, general-purpose, object-oriented programming language with C-style syntax. Dart programming can be used to create a front-end user interface for the web and mobile apps and also to build backed solutions like API’s and other services. Want to know more about Dart? click here
Let’s get straight to business!
What we will be trying to achieve in this demo is to make a basic API that will send a request to the server and the server in turn will process that request and perform basic CRUD operations on the database based on the type/parameters of the request. We will only work with Dart programming language for making the API and to interact with the database (Dart is not just for making beautiful UI’s for mobile and web, it can do the backend work too!).
Let's get started with the installation and setup which is required for this demo.
First, you have to install Dart. You can easily do that by following the steps on Dart's official website here.
You can install the MongoDB community version from here. Select your operating system and follow the steps mentioned on the website.
After you install MongoDB, you can type the mongo
command in the terminal. It will log you into the mongo shell and from here you can interact with your database locally. The show dbs
command can be used to list out the locally available databases to you. To select a database to work with you can use the command use
, it will start using that database as the current database or will create one if the database is not already present.
You can also check the current database by typing the db
command in the mongo shell. It will return the name of the currently used database.
> show dbs admin 0.000GB config 0.000GB dart_test 0.000GB local 0.000GB test 0.000GB > use dart_test switched to db dart_test > db dart_test
Now, you are connected to the database and can play around by creating different collections and adding documents to those collections, but for this demo, we will be inserting dummy data to the database from a JSON file.
Start your MongoDB server by running the mongod
command (mongod
is the primary daemon process for the MongoDB system. It handles data requests, manages data access and performs background management operations).
Then, import the people.json
file in your database using the command:
mongoimport --jsonArray -d yourDatabaseName -c yourCollectionName --file path/To/File.json
You can check whether the JSON file is properly imported by running this command in the mongo shell:
db.yourCollectionName.find()
It should return all the documents inside the collection which you used while importing the dataset from the JSON file.
That's all the setup you need.
Let’s begin with the implementation.
Interaction With DB
To interact with the database, we will use an amazing package that will make this task extremely easy!
We will add the mongo dart package in our pubspec.yaml
file and run the command flutter packages get
to be able to use this package.
We can use this to open a connection with our database.
To open a connection, we need to create an object of the Db
class of the package by passing the URL of our database as a constructor parameter.
After this, we can use the open()
method on it.
That's it. Now we are connected to our database.
Now, we need to create a server to which we will make different requests e.g GET, POST, PUT, DELETE and which will handle these requests by performing the basic CRUD operations on the database based on the type of request. For creating a server we will be using the http_server
package that allows us to handle the HTTP requests made to the server.
We will create a server and start listening to a particular port where all the requests will be made. For this demo, we will be using /people
endpoint to make requests to the server (You can use any available port for listening).
If we make a request to the server using the /people
endpoint, it instantiates a PeopleController
class, which takes the request body and the database reference as the arguments to its constructor. This class can be considered as a controller class that handles the request. It is considered good practice to separate your logical component of the code for a better structure.
The constructor of the PeopleController
class invokes the handle()
which in turn calls handleGet()
, handlePost()
, handlePut()
, handleDelete()
based on the type of request passed to the function. Here, _store
refers to the particular collection name which we have in the database and _req
is the entire request object that we have sent while sending the HTTP request.
Now we have to implement these functions so that they can perform their own respective actions.
Implementing handleGet() method:
This method will be invoked when we make a GET
request to the /people
endpoint. We can either get all the documents of the collection or just a particular document based on the id
passed as a query parameter in the request. If there is no id
passed in the query parameter, we can simply return all the documents from the collection by calling the find()
method on the collection. If there is a particular id
passed as the query parameter, we can call the findOne()
function on it which takes a selector as an argument.
In the selector, we can add some conditions to filter out data based on our requirements.
Similarly, we can implement the handlePost()
, handlePut()
and handleDelete()
functions.
The complete source code is available at Github: https://github.com/SamvitChrungoo/dart_mongo_example
All Done !! You have implemented everything required to make this killer combination work.
Now, you just have to see everything in action.
Just run the main.dart
file using the command:
dart main.dart
You are now listening to the particular port you have targeted in the code. You can make requests to http://localhost:portno/people
using Postman or curl.
Play around with the request to test the above API and see your database changing locally.
Congrats !! You have implemented an API that interacts with MongoDB purely using Dart programming language.
Bonus Tip:
You can also download Robo 3T, a tool that allows you to visualize your databases and help you to do lots of other stuff like:
- Import and export in CSV, JSON, SQL and BSON/mongodump
- Write SQL to query MongoDB
- Auto-complete collection names, field names & more in the shell
- Use a drag-and-drop UI to build queries
- Find outliers in your data and errors in your schema
That’s all for this demo, I hope it helped you in one way or the other.
Thanks for reading. Until next time !! 👋 👋
Dive deep into our research and insights. In our articles and blogs, we explore topics on design, how it relates to development, and impact of various trends to businesses.